理解Python中的线程需要掌握几个核心概念:线程的基本概念、GIL(全局解释器锁)、线程的创建与管理、线程同步机制、线程池的使用。其中,GIL是Python线程的一个重要特性,深入理解GIL对于正确使用多线程编程至关重要。
GIL(全局解释器锁)是Python解释器的一种机制,它确保在任意时刻只有一个线程在执行Python字节码。这意味着,即使在多核处理器上,Python的多线程程序也不能同时执行多个线程的Python代码,导致多线程在CPU密集型任务上表现不佳。然而,对于I/O密集型任务,Python线程仍然可以提高程序的效率。
一、线程的基本概念
线程是操作系统能够进行运算调度的最小单位。它被包含在进程之中,是进程中的实际运作单位。一个进程可以包含多个线程,这些线程共享进程的资源,但线程之间执行是独立的。
1、线程的优点
使用线程可以提高程序的响应能力和利用多核处理器的能力。例如,GUI应用程序可以在一个线程中处理用户输入,在另一个线程中执行后台任务,从而提高用户体验。
2、线程的缺点
多线程编程需要处理线程间的同步问题,容易出现死锁、竞争条件等问题。尤其在Python中,由于GIL的存在,多线程在CPU密集型任务上表现不佳。
二、GIL(全局解释器锁)
GIL是Python解释器的一个全局锁,它限制了在任意时刻只有一个线程可以执行Python字节码。这是为了简化Python解释器的实现,特别是内存管理和垃圾回收机制。
1、GIL的影响
GIL的存在意味着Python多线程程序在CPU密集型任务上不能利用多核处理器的优势。在这种情况下,多线程程序的性能可能甚至不如单线程程序。然而,对于I/O密集型任务,如文件读写、网络通信,多线程仍然可以提高程序的效率,因为这些任务大部分时间花在等待I/O操作完成上,GIL在等待期间会释放,其他线程可以继续执行。
2、如何应对GIL
对于CPU密集型任务,可以使用多进程代替多线程。Python的multiprocessing模块提供了与线程类似的接口,但每个进程有自己的Python解释器和GIL,可以利用多核处理器的优势。此外,还可以使用C扩展模块,绕过GIL,直接调用C函数来进行计算。
三、线程的创建与管理
Python提供了多种方式来创建和管理线程,最常用的是threading模块。
1、使用threading模块创建线程
import threading
def worker():
print("Thread is running")
创建线程
thread = threading.Thread(target=worker)
启动线程
thread.start()
等待线程结束
thread.join()
2、使用类继承创建线程
import threading
class MyThread(threading.Thread):
def run(self):
print("Thread is running")
创建线程
thread = MyThread()
启动线程
thread.start()
等待线程结束
thread.join()
四、线程同步机制
由于线程共享进程的资源,可能会出现多个线程同时修改共享资源的情况,导致数据不一致。为了避免这种情况,需要使用线程同步机制。
1、锁(Lock)
锁是最简单的同步机制,确保在同一时刻只有一个线程可以访问共享资源。
import threading
lock = threading.Lock()
shared_resource = 0
def worker():
global shared_resource
lock.acquire()
try:
shared_resource += 1
finally:
lock.release()
threads = []
for _ in range(10):
thread = threading.Thread(target=worker)
thread.start()
threads.append(thread)
for thread in threads:
thread.join()
print(shared_resource)
2、递归锁(RLock)
递归锁允许同一个线程多次获得锁,而不会导致死锁。
import threading
lock = threading.RLock()
shared_resource = 0
def worker():
global shared_resource
lock.acquire()
try:
lock.acquire()
try:
shared_resource += 1
finally:
lock.release()
finally:
lock.release()
threads = []
for _ in range(10):
thread = threading.Thread(target=worker)
thread.start()
threads.append(thread)
for thread in threads:
thread.join()
print(shared_resource)
五、线程池的使用
线程池是一种线程管理机制,通过维护一定数量的线程来执行任务,避免频繁创建和销毁线程带来的开销。
1、使用concurrent.futures模块
from concurrent.futures import ThreadPoolExecutor
def worker(number):
return number * 2
with ThreadPoolExecutor(max_workers=5) as executor:
futures = [executor.submit(worker, i) for i in range(10)]
results = [future.result() for future in futures]
print(results)
2、线程池的优点
线程池可以提高资源利用率和程序的响应速度,适合处理大量短小的任务。通过控制线程池的大小,可以限制同时运行的线程数量,避免过多线程导致系统资源耗尽。
六、线程的高级使用
除了基本的线程创建和同步机制,Python还提供了一些高级的线程操作方法,如条件变量、信号量和事件。
1、条件变量(Condition)
条件变量允许线程等待某个条件变为真。
import threading
condition = threading.Condition()
shared_resource = 0
def producer():
global shared_resource
with condition:
shared_resource += 1
condition.notify()
def consumer():
global shared_resource
with condition:
condition.wait()
print(shared_resource)
producer_thread = threading.Thread(target=producer)
consumer_thread = threading.Thread(target=consumer)
consumer_thread.start()
producer_thread.start()
producer_thread.join()
consumer_thread.join()
2、信号量(Semaphore)
信号量用于控制对共享资源的访问数量。
import threading
semaphore = threading.Semaphore(2)
shared_resource = 0
def worker():
global shared_resource
semaphore.acquire()
try:
shared_resource += 1
print(shared_resource)
finally:
semaphore.release()
threads = []
for _ in range(5):
thread = threading.Thread(target=worker)
thread.start()
threads.append(thread)
for thread in threads:
thread.join()
3、事件(Event)
事件用于线程间的通信,线程可以等待事件的发生。
import threading
event = threading.Event()
def worker():
event.wait()
print("Event occurred")
thread = threading.Thread(target=worker)
thread.start()
触发事件
event.set()
thread.join()
七、线程安全的数据结构
Python提供了一些线程安全的数据结构,避免手动加锁的复杂性。
1、Queue模块
Queue模块提供了线程安全的队列。
import threading
import queue
q = queue.Queue()
def producer():
for i in range(5):
q.put(i)
def consumer():
while not q.empty():
item = q.get()
print(item)
q.task_done()
producer_thread = threading.Thread(target=producer)
consumer_thread = threading.Thread(target=consumer)
producer_thread.start()
producer_thread.join()
consumer_thread.start()
consumer_thread.join()
2、其他线程安全的数据结构
除了Queue,Python还提供了LifoQueue、PriorityQueue等线程安全的数据结构,适用于不同的应用场景。
八、线程调试技巧
多线程程序调试较为困难,需要一些技巧和工具。
1、使用logging模块
logging模块可以帮助记录多线程程序的运行状态。
import threading
import logging
logging.basicConfig(level=logging.DEBUG, format='%(threadName)s: %(message)s')
def worker():
logging.debug('Starting')
logging.debug('Exiting')
threads = []
for i in range(2):
thread = threading.Thread(name=f'Thread-{i}', target=worker)
thread.start()
threads.append(thread)
for thread in threads:
thread.join()
2、使用pdb调试器
pdb调试器可以用于多线程程序的调试。
import threading
import pdb
def worker():
pdb.set_trace()
print("Thread is running")
thread = threading.Thread(target=worker)
thread.start()
thread.join()
九、线程的最佳实践
为了写出高效、可靠的多线程程序,需要遵循一些最佳实践。
1、避免共享可变状态
尽量避免多个线程共享可变状态,使用不可变对象或线程安全的数据结构。
2、使用高层次的同步机制
优先使用Condition、Semaphore、Event等高层次的同步机制,避免直接使用Lock。
3、避免死锁
确保获取多个锁时总是以相同的顺序,避免循环依赖,防止死锁。
4、使用线程池
对于大量短小任务,使用线程池可以提高程序性能和资源利用率。
5、考虑使用多进程
对于CPU密集型任务,考虑使用多进程代替多线程,绕过GIL的限制。
十、线程与异步编程
除了多线程编程,Python还支持异步编程,通过asyncio模块可以实现高效的I/O并发。
1、asyncio模块
asyncio模块提供了事件循环、协程和任务等异步编程工具。
import asyncio
async def worker():
print("Worker is running")
await asyncio.sleep(1)
print("Worker is done")
async def main():
await asyncio.gather(worker(), worker())
asyncio.run(main())
2、线程与异步结合
在某些情况下,可以结合使用线程和异步编程。
import asyncio
import threading
async def async_worker():
print("Async worker is running")
await asyncio.sleep(1)
print("Async worker is done")
def thread_worker(loop):
asyncio.set_event_loop(loop)
loop.run_until_complete(async_worker())
loop = asyncio.new_event_loop()
thread = threading.Thread(target=thread_worker, args=(loop,))
thread.start()
thread.join()
结论
理解Python中的线程需要掌握线程的基本概念、GIL的影响、线程的创建与管理、线程同步机制、线程池的使用等方面的知识。在实际编程中,需要根据任务的特点选择合适的并发编程方式,避免共享可变状态,使用高层次的同步机制,避免死锁,并考虑使用多进程或异步编程来提高程序性能和资源利用率。通过遵循最佳实践,可以编写出高效、可靠的多线程程序。
相关问答FAQs:
在Python中,线程与进程有什么区别?
线程是进程中的一个执行单元,它共享进程的资源(如内存),而进程则是操作系统分配资源的基本单位。Python中的线程可以更轻松地进行任务切换和共享数据,但由于全局解释器锁(GIL)的存在,多线程在CPU密集型任务中可能无法有效提高性能。相反,进程是独立的,适用于需要完全隔离资源的任务。
使用Python线程时,如何避免竞争条件?
竞争条件发生在多个线程同时访问共享数据时。为避免这种情况,可以使用线程锁(如threading.Lock
)来保证同一时间只有一个线程能访问共享资源。此外,使用条件变量和信号量也可以有效管理线程之间的协作,确保数据安全。
在Python中,如何创建和管理线程?
可以使用threading
模块来创建线程。通过定义一个继承自threading.Thread
的类或者使用threading.Thread
类的实例,结合start()
方法启动线程。在需要时,可以使用join()
方法等待线程完成,确保主程序在所有线程执行结束后再继续进行。使用daemon
属性可以设置线程为守护线程,使得主程序结束时自动终止该线程。
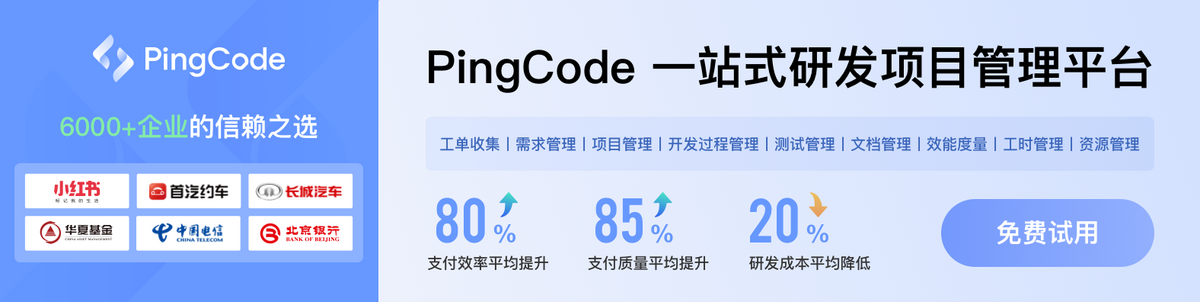