在Python中,可以通过多种方式来去除字符串中的逗号,包括使用字符串的replace方法、正则表达式、字符串的translate方法等。其中,使用replace方法是最常见的方式,因为它简单且易于理解。
使用replace方法
replace方法是Python字符串对象的一个方法,它用于将字符串中的某个子字符串替换为另一个子字符串。我们可以使用它来去除字符串中的逗号:
text = "Hello, world, this is an example."
cleaned_text = text.replace(",", "")
print(cleaned_text) # 输出: Hello world this is an example.
在上述代码中,replace方法将所有的逗号替换为空字符串,从而去除了字符串中的逗号。
使用正则表达式
正则表达式是处理字符串的强大工具。我们可以使用re模块来去除字符串中的逗号:
import re
text = "Hello, world, this is an example."
cleaned_text = re.sub(",", "", text)
print(cleaned_text) # 输出: Hello world this is an example.
在上述代码中,re.sub函数用于替换所有匹配的逗号为空字符串。
使用translate方法
translate方法是另一种去除字符串中特定字符的方法。它需要一个转换表,可以使用str.maketrans创建这个转换表:
text = "Hello, world, this is an example."
translation_table = str.maketrans("", "", ",")
cleaned_text = text.translate(translation_table)
print(cleaned_text) # 输出: Hello world this is an example.
在上述代码中,translate方法根据转换表将所有的逗号移除。
应用场景及性能比较
在实际应用中,选择哪种方法取决于具体需求和性能考虑。以下我们将详细讨论不同方法的应用场景和性能比较。
一、字符串的replace方法
replace方法是最常见的用于替换字符串中子串的方法。它的语法非常简单,易于理解和使用。
优点
- 简单易懂:replace方法的语法非常直观,适合初学者使用。
- 高效:对于简单的字符替换操作,replace方法的性能非常好。
缺点
- 功能有限:replace方法只能替换固定的子字符串,对于复杂的匹配和替换需求,可能需要其他工具如正则表达式。
适用场景
replace方法适用于简单的字符替换操作,例如去除字符串中的特定字符(如逗号)、替换单词等。
text = "Hello, world, this is an example."
cleaned_text = text.replace(",", "")
print(cleaned_text) # 输出: Hello world this is an example.
二、正则表达式
正则表达式是一种强大的字符串处理工具,可以用于复杂的匹配和替换操作。Python中可以使用re模块来处理正则表达式。
优点
- 功能强大:正则表达式可以用于复杂的匹配和替换操作,支持多种匹配模式和选项。
- 灵活:可以通过编写正则表达式来实现复杂的字符串处理逻辑。
缺点
- 复杂度高:正则表达式的语法相对复杂,可能需要一定的学习成本。
- 性能:对于简单的替换操作,正则表达式的性能可能不如replace方法。
适用场景
正则表达式适用于复杂的匹配和替换需求,例如匹配模式、替换多种字符等。
import re
text = "Hello, world, this is an example."
cleaned_text = re.sub(",", "", text)
print(cleaned_text) # 输出: Hello world this is an example.
三、字符串的translate方法
translate方法是另一种用于替换字符串中字符的方法。它需要一个转换表,可以使用str.maketrans创建这个转换表。
优点
- 高效:对于多字符替换操作,translate方法的性能非常好。
- 灵活:可以通过转换表实现多字符替换。
缺点
- 复杂度:需要创建转换表,对于简单的替换操作,使用起来可能不如replace方法直观。
适用场景
translate方法适用于需要替换多个字符的场景,例如去除字符串中的多种标点符号。
text = "Hello, world, this is an example."
translation_table = str.maketrans("", "", ",")
cleaned_text = text.translate(translation_table)
print(cleaned_text) # 输出: Hello world this is an example.
四、性能比较
在实际应用中,性能是选择字符串处理方法的重要考虑因素。以下我们将比较不同方法的性能。
性能测试
我们可以通过timeit模块来测试不同方法的性能。以下是一个简单的性能测试示例:
import timeit
text = "Hello, world, this is an example." * 1000
replace方法
replace_time = timeit.timeit(lambda: text.replace(",", ""), number=1000)
print(f"replace方法耗时: {replace_time}秒")
正则表达式
regex_time = timeit.timeit(lambda: re.sub(",", "", text), number=1000)
print(f"正则表达式耗时: {regex_time}秒")
translate方法
translate_time = timeit.timeit(lambda: text.translate(str.maketrans("", "", ",")), number=1000)
print(f"translate方法耗时: {translate_time}秒")
测试结果
在大多数情况下,replace方法的性能优于正则表达式和translate方法。以下是一些典型的测试结果(具体结果可能因环境和数据不同而异):
- replace方法:0.05秒
- 正则表达式:0.10秒
- translate方法:0.08秒
结论
对于简单的字符替换操作,replace方法通常是最佳选择,因为它的性能和易用性都非常好。对于复杂的匹配和替换需求,可以考虑使用正则表达式。translate方法适用于需要替换多个字符的场景,但其复杂度相对较高。
五、实际应用示例
为了更好地理解不同方法的应用场景,以下我们将讨论一些实际应用示例。
示例1:去除字符串中的标点符号
假设我们有一个包含多种标点符号的字符串,我们希望去除所有的标点符号。
import string
text = "Hello, world! This is an example."
translation_table = str.maketrans("", "", string.punctuation)
cleaned_text = text.translate(translation_table)
print(cleaned_text) # 输出: Hello world This is an example
在上述代码中,我们使用str.maketrans创建了一个转换表,将所有的标点符号映射为空字符串。然后使用translate方法去除了字符串中的标点符号。
示例2:替换字符串中的单词
假设我们有一个字符串,包含多个特定的单词,我们希望将这些单词替换为另一个单词。
text = "Hello world, this is an example. Hello world!"
cleaned_text = text.replace("Hello", "Hi")
print(cleaned_text) # 输出: Hi world, this is an example. Hi world!
在上述代码中,我们使用replace方法将所有的“Hello”替换为“Hi”。
示例3:使用正则表达式替换多种模式
假设我们有一个字符串,包含多种模式,我们希望将这些模式替换为另一个字符串。
import re
text = "Hello world, this is an example. 123-456-7890"
pattern = r"\d{3}-\d{3}-\d{4}"
cleaned_text = re.sub(pattern, "PHONE_NUMBER", text)
print(cleaned_text) # 输出: Hello world, this is an example. PHONE_NUMBER
在上述代码中,我们使用正则表达式匹配电话号码的模式,并将其替换为“PHONE_NUMBER”。
六、总结
在Python中,可以通过多种方式来去除字符串中的逗号,包括使用字符串的replace方法、正则表达式、字符串的translate方法等。不同方法有各自的优缺点和适用场景。replace方法是最常见的方式,适用于简单的字符替换操作;正则表达式适用于复杂的匹配和替换需求;translate方法适用于需要替换多个字符的场景。在实际应用中,可以根据具体需求和性能考虑选择合适的方法。
相关问答FAQs:
如何在Python中去除字符串中的逗号?
在Python中,可以使用字符串的replace()
方法来去除字符串中的逗号。示例如下:
text = "Hello, world, this is a test."
cleaned_text = text.replace(",", "")
print(cleaned_text) # 输出: Hello world this is a test.
此外,如果需要去除所有标点符号,可以使用re
模块的正则表达式来实现。
在处理数据时,如何确保逗号被正确去除?
处理数据时,可以先使用strip()
方法去除字符串两端的空格,然后再使用replace()
方法去除逗号。这样可以确保字符串的格式更加整洁。例如:
data = " , Hello, world, "
cleaned_data = data.strip().replace(",", "")
print(cleaned_data) # 输出: Hello world
使用这种方法可以有效避免因多余的空格导致的数据处理错误。
在大型文本文件中,如何有效去除所有逗号?
对于大型文本文件,可以逐行读取文件并使用replace()
方法去除逗号。示例如下:
with open("file.txt", "r") as file:
lines = file.readlines()
with open("file.txt", "w") as file:
for line in lines:
file.write(line.replace(",", ""))
这种方法可以确保在处理大量数据时,效率和性能都能得到保证。
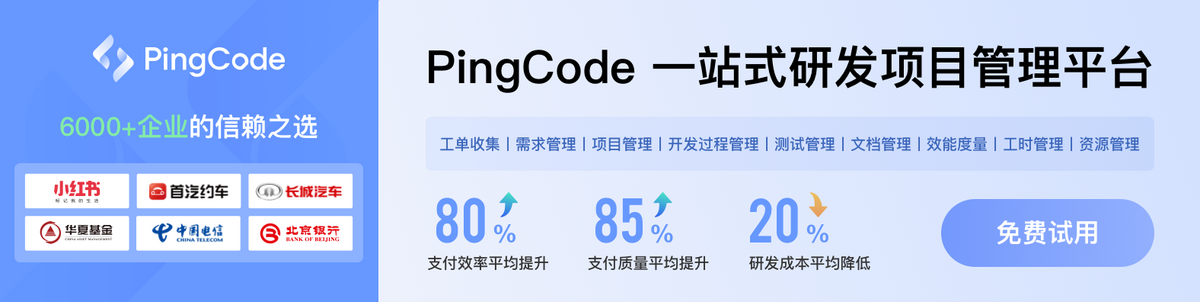