要用Python编写大富翁游戏,你需要使用面向对象编程(OOP)来设计游戏逻辑、创建图形用户界面(GUI)库以呈现游戏界面、以及处理游戏的各种事件。大富翁游戏的编写主要包括以下几个方面:游戏板块的设计、玩家的创建与管理、游戏规则的实现、图形界面的设计与事件处理。在这些方面中,游戏板块的设计是核心部分,需要详细展开。
一、游戏板块的设计
游戏板块是大富翁游戏的基础,它决定了玩家的移动、购买财产、支付租金等一系列行为。设计游戏板块时需要考虑以下几点:
-
创建板块类(Board Class)
板块类是整个游戏的基础,它包含了所有游戏格子的定义和属性。每个格子可能有不同的类型,例如起点、地产、机会卡、命运卡等。
class Board:
def __init__(self):
self.spaces = self.create_spaces()
def create_spaces(self):
spaces = []
# 创建每个格子,初始化属性
for i in range(40): # 假设一共40个格子
if i == 0:
spaces.append(Space("Start"))
elif i % 5 == 0:
spaces.append(Space("Special", special_type="Chance"))
else:
spaces.append(Space("Property", price=100 + i * 10))
return spaces
-
定义格子类(Space Class)
格子类表示每个游戏格子,可能包含不同的属性和方法,例如格子的类型、价格、所有者等。
class Space:
def __init__(self, space_type, price=0, special_type=None):
self.space_type = space_type
self.price = price
self.special_type = special_type
self.owner = None
def buy(self, player):
if self.owner is None and player.money >= self.price:
self.owner = player
player.money -= self.price
player.properties.append(self)
def pay_rent(self, player):
if self.owner and self.owner != player:
rent = self.price // 10
player.money -= rent
self.owner.money += rent
二、玩家的创建与管理
玩家是游戏的主体,他们通过掷骰子移动,并根据落在的格子做出相应的操作。玩家类需要包含玩家的基本信息和操作方法。
-
定义玩家类(Player Class)
玩家类表示每个玩家,包含玩家的资金、位置、拥有的地产等信息。
class Player:
def __init__(self, name):
self.name = name
self.money = 1500 # 初始资金
self.position = 0
self.properties = []
def move(self, steps):
self.position = (self.position + steps) % 40
def buy_property(self, space):
space.buy(self)
def pay_rent(self, space):
space.pay_rent(self)
-
管理玩家
游戏通常有多个玩家,需要一个类来管理所有玩家的轮流操作和游戏状态。
class Game:
def __init__(self, players):
self.board = Board()
self.players = players
self.current_player_index = 0
def next_turn(self):
player = self.players[self.current_player_index]
steps = self.roll_dice()
player.move(steps)
current_space = self.board.spaces[player.position]
self.handle_space(player, current_space)
self.current_player_index = (self.current_player_index + 1) % len(self.players)
def roll_dice(self):
import random
return random.randint(1, 6) + random.randint(1, 6)
def handle_space(self, player, space):
if space.space_type == "Property" and space.owner is None:
player.buy_property(space)
elif space.space_type == "Property" and space.owner != player:
player.pay_rent(space)
# 其他类型格子的处理逻辑
三、游戏规则的实现
大富翁游戏有许多规则,包括购买地产、支付租金、触发事件等。需要在代码中实现这些规则。
-
购买地产
玩家落在一个未被购买的地产格子上时,可以选择购买该地产。
def handle_space(self, player, space):
if space.space_type == "Property" and space.owner is None:
if player.money >= space.price:
player.buy_property(space)
else:
print(f"{player.name} 没有足够的资金购买 {space.price} 的地产")
elif space.space_type == "Property" and space.owner != player:
player.pay_rent(space)
# 其他类型格子的处理逻辑
-
支付租金
玩家落在一个已被其他玩家购买的地产格子上时,需要支付租金给该地产的所有者。
def handle_space(self, player, space):
if space.space_type == "Property" and space.owner is not None and space.owner != player:
if player.money >= space.price // 10:
player.pay_rent(space)
else:
print(f"{player.name} 没有足够的资金支付租金")
# 其他类型格子的处理逻辑
-
触发特殊事件
玩家落在特殊事件格子(例如机会卡、命运卡)上时,需要触发相应的事件。
def handle_space(self, player, space):
if space.space_type == "Special" and space.special_type == "Chance":
self.draw_chance_card(player)
elif space.space_type == "Special" and space.special_type == "Community Chest":
self.draw_community_chest_card(player)
# 其他类型格子的处理逻辑
四、图形界面的设计与事件处理
为了让游戏更具互动性,可以使用Python的图形界面库,例如Tkinter或Pygame,来设计游戏的图形界面。
-
使用Tkinter设计界面
Tkinter是Python内置的图形界面库,适合用于简单的游戏界面设计。
import tkinter as tk
class MonopolyGUI:
def __init__(self, root, game):
self.root = root
self.game = game
self.create_widgets()
def create_widgets(self):
self.board_frame = tk.Frame(self.root)
self.board_frame.pack()
self.create_board()
self.create_controls()
def create_board(self):
for i in range(10):
for j in range(10):
label = tk.Label(self.board_frame, text=f"{i},{j}", borderwidth=1, relief="solid")
label.grid(row=i, column=j)
def create_controls(self):
self.control_frame = tk.Frame(self.root)
self.control_frame.pack()
self.roll_button = tk.Button(self.control_frame, text="Roll Dice", command=self.roll_dice)
self.roll_button.pack()
def roll_dice(self):
self.game.next_turn()
self.update_board()
def update_board(self):
# 更新界面上的玩家位置、资金等信息
pass
if __name__ == "__main__":
root = tk.Tk()
players = [Player("Player 1"), Player("Player 2")]
game = Game(players)
gui = MonopolyGUI(root, game)
root.mainloop()
-
使用Pygame设计界面
Pygame是一个功能更强大的图形界面库,适合用于设计复杂的游戏界面。
import pygame
class MonopolyGUI:
def __init__(self, game):
pygame.init()
self.screen = pygame.display.set_mode((800, 600))
self.game = game
self.running = True
def run(self):
while self.running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
self.running = False
elif event.type == pygame.MOUSEBUTTONDOWN:
self.handle_click(event)
self.update()
self.draw()
def handle_click(self, event):
# 处理点击事件
pass
def update(self):
# 更新游戏状态
pass
def draw(self):
self.screen.fill((255, 255, 255))
# 绘制游戏板、玩家、地产等
pygame.display.flip()
if __name__ == "__main__":
players = [Player("Player 1"), Player("Player 2")]
game = Game(players)
gui = MonopolyGUI(game)
gui.run()
pygame.quit()
五、扩展与优化
在基本功能实现后,可以根据需要进行扩展和优化,例如增加更多的游戏规则、优化界面、增加AI玩家等。
-
增加更多的游戏规则
可以增加更多的游戏规则,例如拍卖未被购买的地产、玩家破产时的处理等。
def handle_space(self, player, space):
if space.space_type == "Property" and space.owner is None:
if player.money >= space.price:
player.buy_property(space)
else:
self.start_auction(space)
elif space.space_type == "Property" and space.owner != player:
if player.money >= space.price // 10:
player.pay_rent(space)
else:
self.handle_bankruptcy(player)
# 其他类型格子的处理逻辑
-
优化界面
可以优化界面设计,使其更加美观和用户友好,例如使用更好的图形资源、添加动画效果等。
-
增加AI玩家
可以为游戏增加AI玩家,使其可以与计算机对战。需要设计AI的决策逻辑,例如何时购买地产、何时拍卖等。
class AIPlayer(Player):
def decide(self, game):
# 设计AI决策逻辑
pass
通过以上步骤,我们可以用Python编写一个完整的大富翁游戏。这个过程不仅可以帮助我们熟悉面向对象编程的基本概念,还可以锻炼我们的逻辑思维和编程能力。希望这个教程对你有所帮助,祝你编程愉快!
相关问答FAQs:
如何开始使用Python编写大富翁游戏?
要开始使用Python编写大富翁游戏,首先需要理解游戏的基本规则和结构,包括游戏板、玩家、骰子、事件和交易等。建议先绘制游戏设计图,列出每个功能模块。然后,可以选择使用Python的图形库,如Pygame,来实现游戏界面和交互。熟悉Python的基本语法和面向对象编程将帮助你更好地组织代码和管理游戏状态。
大富翁游戏中如何实现玩家的移动和骰子投掷功能?
实现玩家的移动和骰子投掷功能通常需要创建一个玩家类和一个骰子类。玩家类可以包含属性如位置、资金和拥有的资产等。骰子类则负责生成随机数,模拟投掷结果。玩家在每次回合开始时投掷骰子,根据结果更新其在游戏板上的位置,并处理相应的事件,如购买地产或支付租金。
在Python编写大富翁游戏时,如何处理游戏中的交易和事件?
交易和事件是大富翁游戏的核心部分。在编写相关功能时,可以创建一个事件类,用于处理不同类型的事件,例如抽卡、支付或获得资金等。交易功能可以通过定义买卖地产的逻辑来实现,包括检查资金是否充足、更新玩家资产和地产状态等。确保在游戏循环中不断检查玩家状态,以便及时触发相应的事件和交易。
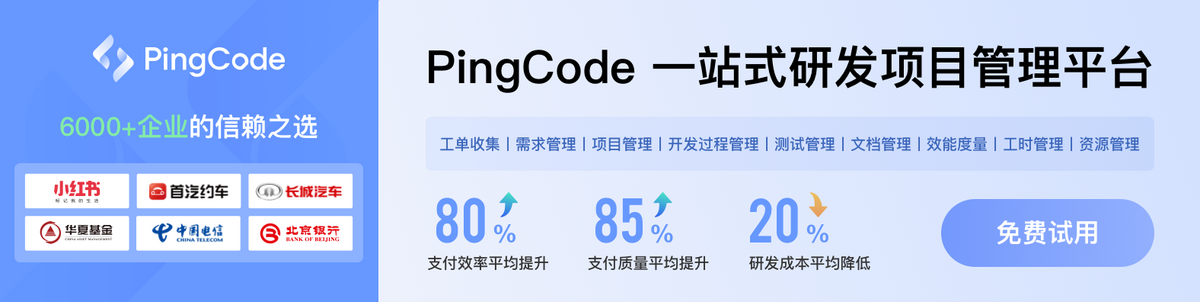