要用Python读取网页文本,可以使用以下几种方法:requests库、BeautifulSoup库、urllib库。其中,requests库是最常用的,因为它提供了简单易用的API。下面将详细描述如何使用requests库读取网页文本。
requests库是一个非常流行的HTTP库,可以很容易地发送HTTP请求并获取响应。首先需要安装requests库,可以使用以下命令:
pip install requests
安装完成后,可以通过以下代码读取网页文本:
import requests
发送HTTP GET请求
response = requests.get('https://example.com')
检查请求是否成功
if response.status_code == 200:
# 获取网页内容
html_content = response.text
print(html_content)
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
在这段代码中,我们首先使用requests.get()方法发送HTTP GET请求,获取网页响应。然后通过检查response.status_code来确认请求是否成功。如果成功,则通过response.text获取网页的HTML内容。
接下来我们将详细介绍另外两种方法,即使用BeautifulSoup库和urllib库读取网页文本。
一、使用BeautifulSoup库
BeautifulSoup是一个用于解析HTML和XML文件的库,通常与requests库配合使用,以便更方便地提取网页内容。
1、安装BeautifulSoup库
首先,需要安装BeautifulSoup库及其依赖库lxml:
pip install beautifulsoup4 lxml
2、使用BeautifulSoup解析网页
import requests
from bs4 import BeautifulSoup
发送HTTP GET请求
response = requests.get('https://example.com')
检查请求是否成功
if response.status_code == 200:
# 获取网页内容
html_content = response.text
# 解析HTML内容
soup = BeautifulSoup(html_content, 'lxml')
# 提取并打印网页文本
print(soup.get_text())
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
在这段代码中,我们首先使用requests.get()方法获取网页内容,然后将其传递给BeautifulSoup进行解析。通过调用soup.get_text()方法,可以提取并打印网页的纯文本内容。
二、使用urllib库
urllib是Python标准库中的一个模块,用于处理URL和HTTP请求。它比requests库稍微复杂一些,但同样可以用来读取网页文本。
1、使用urllib库读取网页
import urllib.request
发送HTTP GET请求
with urllib.request.urlopen('https://example.com') as response:
# 检查请求是否成功
if response.status == 200:
# 获取网页内容
html_content = response.read().decode('utf-8')
print(html_content)
else:
print(f"Failed to retrieve the webpage. Status code: {response.status}")
在这段代码中,我们使用urllib.request.urlopen()方法发送HTTP GET请求,并获取响应。通过检查response.status来确认请求是否成功。如果成功,则通过response.read().decode('utf-8')获取网页的HTML内容。
三、结合requests与BeautifulSoup进行高级解析
在实际应用中,requests库和BeautifulSoup库常常结合使用,以便更方便地解析和提取网页内容。以下是一个结合使用requests和BeautifulSoup进行高级解析的例子:
import requests
from bs4 import BeautifulSoup
发送HTTP GET请求
response = requests.get('https://example.com')
检查请求是否成功
if response.status_code == 200:
# 获取网页内容
html_content = response.text
# 解析HTML内容
soup = BeautifulSoup(html_content, 'lxml')
# 查找所有标题元素
titles = soup.find_all('h1')
# 打印所有标题文本
for title in titles:
print(title.get_text())
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
在这段代码中,我们首先使用requests.get()方法获取网页内容,然后将其传递给BeautifulSoup进行解析。通过调用soup.find_all('h1')方法,查找所有h1标题元素,并打印其文本内容。
四、处理网页中的动态内容
有些网页的内容是通过JavaScript动态生成的,在这种情况下,直接使用requests或urllib库可能无法获取到完整的网页内容。此时,可以使用Selenium库来模拟浏览器行为,获取动态内容。
1、安装Selenium库及其依赖
首先,需要安装Selenium库:
pip install selenium
还需要下载对应浏览器的驱动程序,例如ChromeDriver,下载地址:https://sites.google.com/a/chromium.org/chromedriver/
2、使用Selenium读取动态网页内容
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
设置ChromeDriver路径
chrome_driver_path = '/path/to/chromedriver'
初始化Chrome浏览器
service = Service(chrome_driver_path)
driver = webdriver.Chrome(service=service)
访问网页
driver.get('https://example.com')
try:
# 等待页面加载完成
WebDriverWait(driver, 10).until(EC.presence_of_element_located((By.TAG_NAME, 'body')))
# 获取网页内容
html_content = driver.page_source
print(html_content)
finally:
# 关闭浏览器
driver.quit()
在这段代码中,我们使用Selenium模拟浏览器行为,访问网页并等待页面加载完成。通过driver.page_source获取网页的HTML内容。
五、处理网页中的表单提交
有时需要在读取网页内容之前提交表单,例如登录页面。可以使用requests库模拟表单提交。
1、使用requests库提交表单
import requests
表单数据
form_data = {
'username': 'your_username',
'password': 'your_password'
}
发送POST请求提交表单
response = requests.post('https://example.com/login', data=form_data)
检查请求是否成功
if response.status_code == 200:
# 获取提交表单后的网页内容
html_content = response.text
print(html_content)
else:
print(f"Failed to submit the form. Status code: {response.status_code}")
在这段代码中,我们使用requests.post()方法发送POST请求,提交表单数据。通过检查response.status_code来确认请求是否成功。如果成功,则通过response.text获取提交表单后的网页内容。
六、处理网页中的Cookies和Session
在某些情况下,需要处理Cookies和Session,以便在多个请求之间保持状态。可以使用requests库中的Session对象来管理Cookies和Session。
1、使用requests库处理Cookies和Session
import requests
创建Session对象
session = requests.Session()
发送POST请求提交表单并保存Cookies
login_data = {
'username': 'your_username',
'password': 'your_password'
}
response = session.post('https://example.com/login', data=login_data)
检查登录是否成功
if response.status_code == 200:
# 使用保存的Cookies发送另一个请求
response = session.get('https://example.com/protected_page')
# 获取受保护页面的内容
if response.status_code == 200:
html_content = response.text
print(html_content)
else:
print(f"Failed to retrieve the protected page. Status code: {response.status_code}")
else:
print(f"Failed to log in. Status code: {response.status_code}")
在这段代码中,我们首先使用Session对象发送POST请求提交表单并保存Cookies。然后使用保存的Cookies发送另一个请求,获取受保护页面的内容。
七、处理网页中的重定向
有些网页在访问时会发生重定向,可以使用requests库处理重定向。
1、使用requests库处理重定向
import requests
发送HTTP GET请求
response = requests.get('https://example.com', allow_redirects=True)
检查请求是否成功
if response.status_code == 200:
# 获取网页内容
html_content = response.text
print(html_content)
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
在这段代码中,我们使用requests.get()方法发送HTTP GET请求,并通过allow_redirects=True参数允许重定向。通过检查response.status_code来确认请求是否成功。如果成功,则通过response.text获取网页的HTML内容。
八、处理网页中的编码问题
在读取网页内容时,可能会遇到编码问题。可以使用requests库的encoding属性解决编码问题。
1、处理网页中的编码问题
import requests
发送HTTP GET请求
response = requests.get('https://example.com')
检查请求是否成功
if response.status_code == 200:
# 设置正确的编码
response.encoding = 'utf-8'
# 获取网页内容
html_content = response.text
print(html_content)
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
在这段代码中,我们首先使用requests.get()方法发送HTTP GET请求,然后通过设置response.encoding属性为正确的编码解决编码问题。通过response.text获取网页的HTML内容。
九、处理网页中的异常情况
在读取网页内容时,可能会遇到各种异常情况。可以使用try-except语句处理异常情况。
1、处理网页中的异常情况
import requests
try:
# 发送HTTP GET请求
response = requests.get('https://example.com')
# 检查请求是否成功
if response.status_code == 200:
# 获取网页内容
html_content = response.text
print(html_content)
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
except requests.RequestException as e:
print(f"An error occurred: {e}")
在这段代码中,我们使用try-except语句捕获并处理请求过程中可能出现的异常情况。通过检查response.status_code来确认请求是否成功。如果成功,则通过response.text获取网页的HTML内容。
十、总结
在本篇文章中,我们详细介绍了如何使用Python读取网页文本,包括使用requests库、BeautifulSoup库和urllib库的基本方法。还探讨了处理动态内容、表单提交、Cookies和Session、重定向、编码问题以及异常处理等高级应用场景。
通过结合使用requests和BeautifulSoup库,可以方便地解析和提取网页内容。而对于动态内容,可以借助Selenium库模拟浏览器行为。处理表单提交、Cookies和Session、重定向、编码问题和异常情况,可以使网页读取更加稳定和可靠。
这些方法和技巧将帮助你在实际应用中更有效地读取和解析网页文本,从而更好地完成各种网页数据获取任务。
相关问答FAQs:
如何在Python中提取网页中的特定内容?
要提取网页中的特定内容,可以使用Beautiful Soup库。首先,安装Beautiful Soup和requests库。接着,使用requests获取网页内容,并用Beautiful Soup解析HTML。你可以通过查找特定标签或类名来提取所需的信息。例如,使用soup.find_all('h1')
可以获取所有的h1标题。
使用Python读取网页文本时,有哪些常见的错误及解决方法?
在读取网页文本时,常见的错误包括网络连接失败、请求被拒绝或页面结构变化等。确保你的网络连接正常,使用适当的用户代理(User-Agent)来模拟浏览器请求。此外,查看网页的HTML结构,确认你使用的选择器是否仍然有效,必要时更新你的代码。
如何处理读取网页文本后的编码问题?
在读取网页文本时,可能会遇到编码不一致的问题。使用requests库时,可以通过response.encoding
属性指定编码格式。如果网页使用UTF-8编码,确保你的代码中也使用相同的编码。处理文本时,可以使用.encode()
和.decode()
方法来转换不同的编码格式,确保文本在处理过程中的一致性。
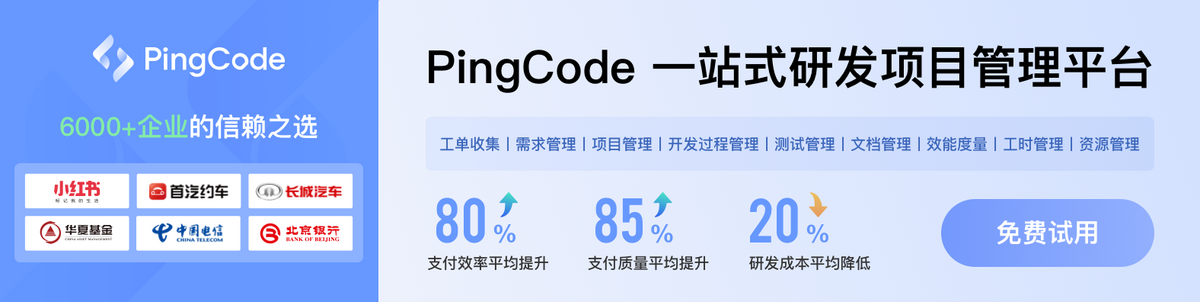