在Python中,修改字符串的方法有多种,主要包括使用字符串方法、切片操作、字符串拼接、字符串格式化、正则表达式等。下面将详细介绍每一种方法及其应用场景。
一、使用字符串方法
Python内置了许多字符串方法,可以帮助你轻松地修改字符串。这些方法包括replace()
, strip()
, split()
, join()
等。
1. replace()
方法
replace()
方法用于替换字符串中的某些部分。它的基本语法是:
str.replace(old, new[, count])
old
:要被替换的子字符串。new
:用于替换的子字符串。count
:可选参数,指定替换的次数。如果不指定,默认替换所有匹配的子字符串。
例子:
text = "Hello World"
modified_text = text.replace("World", "Python")
print(modified_text) # 输出:Hello Python
2. strip()
方法
strip()
方法用于去除字符串两端的指定字符(默认为空格)。它的基本语法是:
str.strip([chars])
chars
:可选参数,指定要去除的字符集合。
例子:
text = " Hello World "
modified_text = text.strip()
print(modified_text) # 输出:Hello World
3. split()
方法和join()
方法
split()
方法用于将字符串拆分成列表,而join()
方法用于将列表中的元素连接成字符串。
例子:
text = "Hello World"
split_text = text.split()
print(split_text) # 输出:['Hello', 'World']
joined_text = " ".join(split_text)
print(joined_text) # 输出:Hello World
二、切片操作
切片操作是一种非常强大的字符串操作方式,允许你通过索引来访问字符串的一部分。
1. 基本切片
基本切片的语法是:
str[start:stop:step]
start
:开始索引(包含)。stop
:结束索引(不包含)。step
:步长(可选)。
例子:
text = "Hello World"
sliced_text = text[0:5]
print(sliced_text) # 输出:Hello
2. 负索引切片
负索引允许你从字符串的末尾开始计数。
例子:
text = "Hello World"
sliced_text = text[-5:]
print(sliced_text) # 输出:World
三、字符串拼接
字符串拼接是将多个字符串组合成一个新的字符串。常用的方法有使用加号运算符(+
)和格式化字符串。
1. 使用加号运算符
加号运算符可以将两个或多个字符串连接在一起。
例子:
part1 = "Hello"
part2 = "World"
combined = part1 + " " + part2
print(combined) # 输出:Hello World
2. 使用格式化字符串
格式化字符串提供了一种更灵活的拼接方式。常用的方法有%
运算符、str.format()
方法和f字符串(Python 3.6+)。
例子:
name = "World"
formatted = "Hello %s" % name
print(formatted) # 输出:Hello World
formatted = "Hello {}".format(name)
print(formatted) # 输出:Hello World
formatted = f"Hello {name}"
print(formatted) # 输出:Hello World
四、字符串格式化
字符串格式化是指将变量值插入到字符串中的一种方式。常用的方法有%
运算符、str.format()
方法和f字符串。
1. %
运算符
%
运算符用于将一个值或多个值插入到字符串中。
例子:
name = "World"
formatted = "Hello %s" % name
print(formatted) # 输出:Hello World
2. str.format()
方法
str.format()
方法提供了一种更强大的字符串格式化方式。
例子:
name = "World"
formatted = "Hello {}".format(name)
print(formatted) # 输出:Hello World
3. f字符串(Python 3.6+)
f字符串提供了一种更加简洁和直观的格式化方式。
例子:
name = "World"
formatted = f"Hello {name}"
print(formatted) # 输出:Hello World
五、正则表达式
正则表达式是一种强大的字符串操作工具,适用于复杂的模式匹配和替换。Python的re
模块提供了正则表达式的支持。
1. re.sub()
方法
re.sub()
方法用于替换字符串中的匹配项。
例子:
import re
text = "Hello World"
modified_text = re.sub(r"World", "Python", text)
print(modified_text) # 输出:Hello Python
2. re.findall()
方法
re.findall()
方法用于查找所有匹配的子字符串。
例子:
import re
text = "Hello World"
matches = re.findall(r"\b\w+\b", text)
print(matches) # 输出:['Hello', 'World']
六、综合应用
在实际应用中,往往需要结合多种方法来实现复杂的字符串修改。下面是一个综合应用的例子。
例子:清洗文本数据
假设你需要清洗一段文本数据,包括去除多余的空格、替换特定的词语、格式化输出等。
import re
def clean_text(text):
# 去除多余的空格
text = text.strip()
# 替换特定的词语
text = text.replace("bad", "good")
# 使用正则表达式去除标点符号
text = re.sub(r'[^\w\s]', '', text)
# 格式化输出
words = text.split()
cleaned_text = " ".join(words)
return cleaned_text
original_text = " This is a bad example. But it will become good! "
cleaned_text = clean_text(original_text)
print(cleaned_text) # 输出:This is a good example But it will become good
通过以上步骤,你可以有效地修改和清洗字符串,以满足实际需求。
七、其他字符串处理技巧
1. 大小写转换
Python提供了一些方法来修改字符串的大小写,包括upper()
, lower()
, capitalize()
, title()
等。
例子:
text = "hello world"
print(text.upper()) # 输出:HELLO WORLD
print(text.lower()) # 输出:hello world
print(text.capitalize()) # 输出:Hello world
print(text.title()) # 输出:Hello World
2. 字符串查找
Python提供了一些方法来查找子字符串的位置,包括find()
, rfind()
, index()
, rindex()
等。
例子:
text = "Hello World"
print(text.find("World")) # 输出:6
print(text.rfind("o")) # 输出:7
print(text.index("World")) # 输出:6
print(text.rindex("o")) # 输出:7
3. 检查字符串内容
Python提供了一些方法来检查字符串的内容,包括isalpha()
, isdigit()
, isalnum()
, isspace()
等。
例子:
text = "Hello"
print(text.isalpha()) # 输出:True
text = "1234"
print(text.isdigit()) # 输出:True
text = "Hello123"
print(text.isalnum()) # 输出:True
text = " "
print(text.isspace()) # 输出:True
八、字符串的编码与解码
在处理不同编码的字符串时,了解如何进行编码和解码是非常重要的。Python提供了encode()
和decode()
方法来进行编码和解码。
1. encode()
方法
encode()
方法用于将字符串编码为字节序列。
例子:
text = "Hello World"
encoded_text = text.encode("utf-8")
print(encoded_text) # 输出:b'Hello World'
2. decode()
方法
decode()
方法用于将字节序列解码为字符串。
例子:
encoded_text = b'Hello World'
decoded_text = encoded_text.decode("utf-8")
print(decoded_text) # 输出:Hello World
九、总结
通过以上介绍,你应该对Python中修改字符串的各种方法有了全面的了解。无论是使用字符串方法、切片操作、字符串拼接、字符串格式化,还是正则表达式,每种方法都有其独特的应用场景。在实际开发中,往往需要结合多种方法来实现复杂的字符串操作。掌握这些技巧,将大大提高你的编程效率和代码质量。
相关问答FAQs:
1. 如何在Python中替换字符串中的特定字符或子字符串?
在Python中,可以使用字符串的replace()
方法来替换特定的字符或子字符串。这个方法接收两个参数:要被替换的子字符串和新的子字符串。例如,my_string.replace("old", "new")
将把字符串中的“old”替换为“new”。此外,您还可以指定替换的次数,my_string.replace("old", "new", count)
会限制替换的次数。
2. 有哪些方法可以在Python中删除字符串的开头和结尾空白字符?
Python提供了strip()
、lstrip()
和rstrip()
方法来处理空白字符。strip()
方法会删除字符串两端的空白字符,而lstrip()
只会删除开头的空白,rstrip()
则只删除结尾的空白。使用示例:my_string.strip()
将返回去除前后空白的新字符串。
3. 如何在Python中将字符串转换为大写或小写?
可以使用upper()
和lower()
方法将字符串转换为大写或小写。my_string.upper()
会将字符串中的所有字母转换为大写字母,而my_string.lower()
则会将所有字母转换为小写字母。此外,还有capitalize()
方法,它会将字符串的首字母转换为大写,其他字母转换为小写,适合用于格式化句子开头的字母。
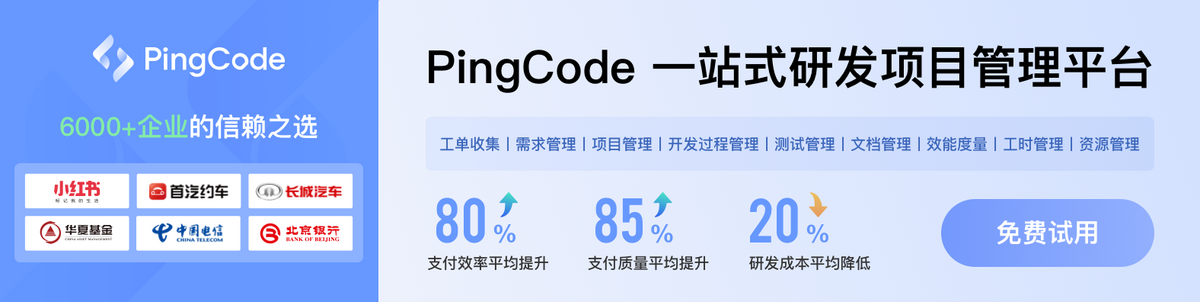