在Python中使用pip安装MySQL的方法是:使用pip命令、配置好MySQL连接、验证安装成功。 下面将详细介绍如何进行这些步骤。
一、使用pip命令安装MySQL
在Python中使用pip安装MySQL的步骤如下:
-
确保pip已经安装:首先确保你的系统中已经安装了pip。你可以通过以下命令来检查:
pip --version
如果没有安装,可以通过以下命令安装:
python -m ensurepip --default-pip
-
安装mysql-connector-python:这是官方推荐的MySQL连接器库。使用以下命令安装:
pip install mysql-connector-python
二、配置好MySQL连接
安装完成后,你需要配置好MySQL连接。以下是一个简单的示例代码:
import mysql.connector
配置数据库连接信息
config = {
'user': 'your_username',
'password': 'your_password',
'host': 'localhost',
'database': 'your_database',
'raise_on_warnings': True
}
连接到数据库
try:
connection = mysql.connector.connect(config)
print("Connection established!")
except mysql.connector.Error as err:
print(f"Error: {err}")
finally:
if connection.is_connected():
connection.close()
print("Connection closed.")
三、验证安装成功
要验证MySQL连接器是否安装成功,可以运行上面的代码。如果输出显示“Connection established!”并且没有错误信息,那么恭喜你,安装成功了。否则,请检查配置中的用户名、密码、主机名和数据库名是否正确。
四、常见问题与解决方法
1. 安装过程中遇到问题
- 问题描述:在使用
pip install mysql-connector-python
命令时,可能会遇到一些问题,如“找不到匹配的分发包”。 - 解决方法:确保你的pip版本是最新的,可以使用以下命令升级pip:
pip install --upgrade pip
如果仍然遇到问题,可以尝试使用其他MySQL连接器库,如
PyMySQL
:pip install pymysql
2. 连接数据库失败
- 问题描述:运行连接代码时,出现“Access denied for user”等错误。
- 解决方法:检查数据库的用户名和密码是否正确,确保你的MySQL服务器正在运行,并且配置允许远程连接(如果你不在本地连接)。
3. 连接慢或超时
- 问题描述:连接数据库时,速度很慢或者直接超时。
- 解决方法:可以检查网络连接是否稳定,数据库服务器的性能是否正常,或者增加连接超时时间。例如:
config = {
'user': 'your_username',
'password': 'your_password',
'host': 'localhost',
'database': 'your_database',
'raise_on_warnings': True,
'connection_timeout': 10 # 设置连接超时时间为10秒
}
五、使用MySQL进行基本的数据库操作
在成功安装和连接到MySQL数据库之后,可以进行一些基本的数据库操作,如查询、插入、更新和删除数据。
1. 创建表
import mysql.connector
config = {
'user': 'your_username',
'password': 'your_password',
'host': 'localhost',
'database': 'your_database'
}
try:
connection = mysql.connector.connect(config)
cursor = connection.cursor()
cursor.execute(
"CREATE TABLE IF NOT EXISTS employees ("
"id INT AUTO_INCREMENT PRIMARY KEY,"
"name VARCHAR(255) NOT NULL,"
"position VARCHAR(255),"
"hire_date DATE"
")"
)
print("Table created successfully.")
except mysql.connector.Error as err:
print(f"Error: {err}")
finally:
if connection.is_connected():
cursor.close()
connection.close()
2. 插入数据
import mysql.connector
config = {
'user': 'your_username',
'password': 'your_password',
'host': 'localhost',
'database': 'your_database'
}
try:
connection = mysql.connector.connect(config)
cursor = connection.cursor()
add_employee = (
"INSERT INTO employees (name, position, hire_date) "
"VALUES (%s, %s, %s)"
)
data_employee = ('John Doe', 'Software Engineer', '2023-01-01')
cursor.execute(add_employee, data_employee)
connection.commit()
print(f"Inserted {cursor.rowcount} row(s) of data.")
except mysql.connector.Error as err:
print(f"Error: {err}")
finally:
if connection.is_connected():
cursor.close()
connection.close()
3. 查询数据
import mysql.connector
config = {
'user': 'your_username',
'password': 'your_password',
'host': 'localhost',
'database': 'your_database'
}
try:
connection = mysql.connector.connect(config)
cursor = connection.cursor()
query = "SELECT id, name, position, hire_date FROM employees"
cursor.execute(query)
for (id, name, position, hire_date) in cursor:
print(f"{id}: {name}, {position}, {hire_date}")
except mysql.connector.Error as err:
print(f"Error: {err}")
finally:
if connection.is_connected():
cursor.close()
connection.close()
4. 更新数据
import mysql.connector
config = {
'user': 'your_username',
'password': 'your_password',
'host': 'localhost',
'database': 'your_database'
}
try:
connection = mysql.connector.connect(config)
cursor = connection.cursor()
update_employee = (
"UPDATE employees SET position = %s WHERE name = %s"
)
data_employee = ('Senior Software Engineer', 'John Doe')
cursor.execute(update_employee, data_employee)
connection.commit()
print(f"Updated {cursor.rowcount} row(s) of data.")
except mysql.connector.Error as err:
print(f"Error: {err}")
finally:
if connection.is_connected():
cursor.close()
connection.close()
5. 删除数据
import mysql.connector
config = {
'user': 'your_username',
'password': 'your_password',
'host': 'localhost',
'database': 'your_database'
}
try:
connection = mysql.connector.connect(config)
cursor = connection.cursor()
delete_employee = (
"DELETE FROM employees WHERE name = %s"
)
data_employee = ('John Doe',)
cursor.execute(delete_employee, data_employee)
connection.commit()
print(f"Deleted {cursor.rowcount} row(s) of data.")
except mysql.connector.Error as err:
print(f"Error: {err}")
finally:
if connection.is_connected():
cursor.close()
connection.close()
六、进阶操作与优化
在进行基本的数据库操作之后,你可能需要进行一些更为复杂的操作和优化,以确保你的应用程序能够高效、稳定地运行。
1. 使用连接池
使用连接池可以提高数据库连接的效率,减少连接的开销。以下是一个使用连接池的示例:
import mysql.connector
from mysql.connector import pooling
config = {
'user': 'your_username',
'password': 'your_password',
'host': 'localhost',
'database': 'your_database'
}
try:
connection_pool = mysql.connector.pooling.MySQLConnectionPool(pool_name="mypool",
pool_size=5,
config)
connection = connection_pool.get_connection()
cursor = connection.cursor()
cursor.execute("SELECT DATABASE()")
db_name = cursor.fetchone()
print(f"Connected to database: {db_name}")
except mysql.connector.Error as err:
print(f"Error: {err}")
finally:
if connection.is_connected():
cursor.close()
connection.close()
2. 执行批量插入操作
批量插入操作可以提高数据插入的效率。以下是一个批量插入数据的示例:
import mysql.connector
config = {
'user': 'your_username',
'password': 'your_password',
'host': 'localhost',
'database': 'your_database'
}
try:
connection = mysql.connector.connect(config)
cursor = connection.cursor()
add_employee = (
"INSERT INTO employees (name, position, hire_date) "
"VALUES (%s, %s, %s)"
)
data_employees = [
('John Doe', 'Software Engineer', '2023-01-01'),
('Jane Smith', 'Data Scientist', '2023-02-01'),
('Mike Johnson', 'Product Manager', '2023-03-01')
]
cursor.executemany(add_employee, data_employees)
connection.commit()
print(f"Inserted {cursor.rowcount} rows of data.")
except mysql.connector.Error as err:
print(f"Error: {err}")
finally:
if connection.is_connected():
cursor.close()
connection.close()
3. 使用事务管理
在进行一些需要保证数据一致性的操作时,可以使用事务管理。以下是一个使用事务管理的示例:
import mysql.connector
config = {
'user': 'your_username',
'password': 'your_password',
'host': 'localhost',
'database': 'your_database'
}
try:
connection = mysql.connector.connect(config)
cursor = connection.cursor()
connection.start_transaction()
cursor.execute("UPDATE employees SET position = 'Lead Developer' WHERE name = 'John Doe'")
cursor.execute("UPDATE employees SET position = 'Lead Data Scientist' WHERE name = 'Jane Smith'")
connection.commit()
print("Transaction committed.")
except mysql.connector.Error as err:
connection.rollback()
print(f"Error: {err}")
finally:
if connection.is_connected():
cursor.close()
connection.close()
七、总结
通过以上的讲解,你应该已经掌握了在Python中使用pip安装MySQL的方法,并且了解了如何配置数据库连接、进行基本的数据库操作以及一些进阶的操作和优化。使用pip安装mysql-connector-python是最推荐的方式,因为它是MySQL官方提供的连接器,功能完善且性能优异。配置好MySQL连接是确保你的应用程序能够正常连接到数据库的关键步骤,而验证安装成功可以帮助你快速确认环境配置是否正确。希望这些内容能对你有所帮助!
相关问答FAQs:
在Python中使用pip安装MySQL的步骤是什么?
要在Python中使用pip安装MySQL,你需要确保已经安装了Python和pip。打开命令行界面,输入以下命令:pip install mysql-connector-python
。这个命令将安装MySQL连接器,使你能够在Python中与MySQL数据库进行交互。
安装MySQL连接库后如何测试是否成功?
安装完MySQL连接库后,可以通过编写一个简单的Python脚本来测试。创建一个名为test_mysql.py
的文件,写入以下代码:
import mysql.connector
try:
conn = mysql.connector.connect(
host="localhost",
user="your_username",
password="your_password"
)
print("Successfully connected to MySQL")
except mysql.connector.Error as err:
print(f"Error: {err}")
finally:
if conn.is_connected():
conn.close()
替换your_username
和your_password
为你自己的MySQL用户信息。运行这个脚本,如果能成功连接,就说明安装成功。
如果在安装过程中遇到错误该如何解决?
常见的错误可能包括找不到pip或mysql-connector-python库。首先,确保pip已经正确安装并在系统路径中。可以通过命令python -m ensurepip
来安装pip。如果库无法找到,检查你的网络连接,或者尝试使用pip install --upgrade pip
来更新pip版本后重试安装。
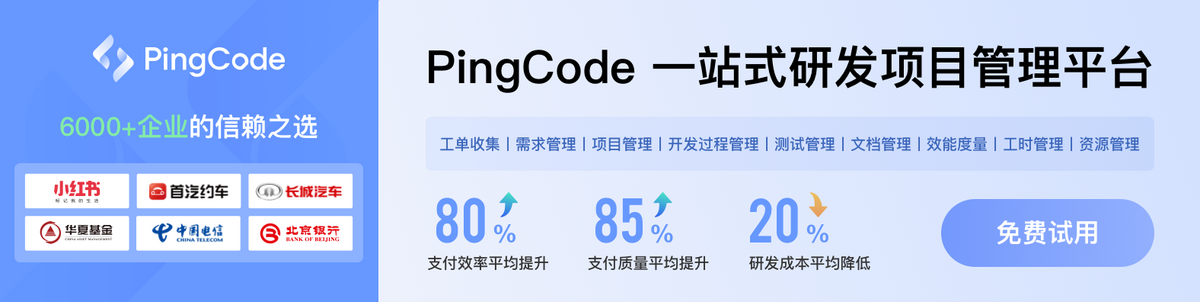