在Python中,暂停执行脚本的方法包括使用time.sleep()、input()函数、信号处理、以及线程等待等。其中,time.sleep()是最常用的方法。下面详细介绍time.sleep()的使用:
time.sleep()函数可以让程序暂停执行指定的秒数,这是通过引入time模块并调用sleep()函数实现的。例如,time.sleep(5)
会让程序暂停执行5秒钟。在某些情况下,我们可能需要让程序暂停一段时间以等待某些外部条件的变化,time.sleep()是一个非常有用的工具。
import time
print("Start")
time.sleep(5)
print("End")
在上面的例子中,程序在打印“Start”后暂停5秒钟,然后再打印“End”。
一、使用time.sleep()暂停执行脚本
1、基本用法
time.sleep()函数是Python标准库中的time模块提供的一个函数。它的参数是暂停的秒数,参数可以是整数或浮点数,表示秒数。
import time
暂停执行2秒
time.sleep(2)
在上面的例子中,程序会在执行到time.sleep(2)时暂停2秒钟,然后继续执行后面的代码。
2、使用浮点数
time.sleep()函数的参数可以是浮点数,这样可以实现更精确的暂停时间。
import time
暂停执行0.5秒
time.sleep(0.5)
在上面的例子中,程序会在执行到time.sleep(0.5)时暂停0.5秒钟,然后继续执行后面的代码。
3、结合循环使用
在循环中使用time.sleep()函数可以实现定时执行某些操作。
import time
for i in range(5):
print("Hello")
time.sleep(1)
在上面的例子中,程序会每隔1秒钟打印一次“Hello”,连续打印5次。
二、使用input()函数暂停执行脚本
1、基本用法
input()函数可以使程序暂停执行,直到用户输入内容并按下回车键。这个方法通常用于等待用户的输入。
# 暂停执行,等待用户输入
input("Press Enter to continue...")
在上面的例子中,程序会在执行到input()函数时暂停,直到用户输入内容并按下回车键后才会继续执行后面的代码。
2、结合循环使用
在循环中使用input()函数可以实现等待用户操作的功能。
for i in range(3):
print(f"Step {i + 1}")
input("Press Enter to continue...")
在上面的例子中,程序会在每一步操作后暂停,等待用户按下回车键后继续执行下一步操作。
三、使用信号处理暂停执行脚本
1、基本用法
在某些情况下,我们可能需要让程序在接收到特定信号时暂停执行。可以使用Python的signal模块来处理这种情况。
import signal
import time
def handler(signum, frame):
print("Signal received, pausing execution")
time.sleep(5)
print("Resuming execution")
signal.signal(signal.SIGUSR1, handler)
print("Waiting for signal")
while True:
time.sleep(1)
在上面的例子中,当程序接收到SIGUSR1信号时,会调用handler函数,程序在handler函数中暂停执行5秒钟。
2、发送信号
可以在另一个终端中使用kill命令发送SIGUSR1信号给运行中的Python脚本。
kill -SIGUSR1 <pid>
其中,
四、使用线程等待暂停执行脚本
1、基本用法
在多线程编程中,使用线程的条件变量(Condition)可以实现线程的暂停和唤醒。
import threading
import time
condition = threading.Condition()
def worker():
print("Worker started")
with condition:
condition.wait() # 暂停线程,等待通知
print("Worker resumed")
thread = threading.Thread(target=worker)
thread.start()
time.sleep(2)
print("Main thread notifying worker")
with condition:
condition.notify() # 通知暂停的线程继续执行
在上面的例子中,worker线程在调用condition.wait()后暂停,直到主线程调用condition.notify()通知它继续执行。
2、结合生产者消费者模型
线程等待可以在生产者消费者模型中使用,以协调多个线程之间的工作。
import threading
import time
import queue
q = queue.Queue()
condition = threading.Condition()
def producer():
for i in range(5):
time.sleep(1)
with condition:
q.put(i)
print(f"Produced {i}")
condition.notify() # 通知消费者线程
def consumer():
while True:
with condition:
condition.wait() # 等待通知
item = q.get()
print(f"Consumed {item}")
if item == 4:
break
thread1 = threading.Thread(target=producer)
thread2 = threading.Thread(target=consumer)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
在上面的例子中,生产者线程生产数据,并通知消费者线程消费数据,消费者线程在接收到通知后继续执行。
五、使用asyncio实现异步暂停
1、基本用法
在异步编程中,可以使用asyncio.sleep()函数实现异步暂停。
import asyncio
async def main():
print("Start")
await asyncio.sleep(2)
print("End")
asyncio.run(main())
在上面的例子中,程序会在执行到await asyncio.sleep(2)时暂停2秒钟,然后继续执行后面的代码。
2、结合异步任务
可以在多个异步任务中使用asyncio.sleep()函数,实现异步任务的暂停和调度。
import asyncio
async def task1():
print("Task 1 start")
await asyncio.sleep(1)
print("Task 1 end")
async def task2():
print("Task 2 start")
await asyncio.sleep(2)
print("Task 2 end")
async def main():
await asyncio.gather(task1(), task2())
asyncio.run(main())
在上面的例子中,两个异步任务task1和task2会并发执行,分别暂停1秒钟和2秒钟。
六、使用定时器暂停执行脚本
1、基本用法
可以使用threading.Timer类实现定时器功能,在指定时间后执行特定操作。
import threading
def timeout():
print("Timeout reached")
timer = threading.Timer(3, timeout)
timer.start()
print("Timer started")
在上面的例子中,程序在启动定时器3秒钟后会执行timeout函数。
2、取消定时器
可以在定时器到期前取消定时器。
import threading
def timeout():
print("Timeout reached")
timer = threading.Timer(3, timeout)
timer.start()
print("Timer started")
timer.cancel()
print("Timer canceled")
在上面的例子中,定时器在启动后立即被取消,因此timeout函数不会被执行。
七、使用事件暂停执行脚本
1、基本用法
可以使用threading.Event类实现线程之间的同步,使用事件对象控制线程的暂停和继续执行。
import threading
import time
event = threading.Event()
def worker():
print("Worker waiting")
event.wait() # 暂停线程,等待事件触发
print("Worker resumed")
thread = threading.Thread(target=worker)
thread.start()
time.sleep(2)
print("Main thread setting event")
event.set() # 触发事件,通知等待的线程继续执行
在上面的例子中,worker线程在调用event.wait()后暂停,直到主线程调用event.set()触发事件。
2、重置事件
可以在事件触发后重置事件,使其可以再次等待。
import threading
import time
event = threading.Event()
def worker():
print("Worker waiting")
event.wait() # 暂停线程,等待事件触发
print("Worker resumed")
thread = threading.Thread(target=worker)
thread.start()
time.sleep(2)
print("Main thread setting event")
event.set() # 触发事件,通知等待的线程继续执行
time.sleep(1)
event.clear() # 重置事件,使其可以再次等待
print("Event cleared")
在上面的例子中,worker线程在调用event.wait()后暂停,直到主线程调用event.set()触发事件,之后事件被重置,可以再次等待。
八、使用条件变量暂停执行脚本
1、基本用法
可以使用threading.Condition类实现线程之间的同步,使用条件变量控制线程的暂停和继续执行。
import threading
condition = threading.Condition()
def worker():
with condition:
print("Worker waiting")
condition.wait() # 暂停线程,等待通知
print("Worker resumed")
thread = threading.Thread(target=worker)
thread.start()
import time
time.sleep(2)
with condition:
print("Main thread notifying worker")
condition.notify() # 通知等待的线程继续执行
在上面的例子中,worker线程在调用condition.wait()后暂停,直到主线程调用condition.notify()通知它继续执行。
2、结合生产者消费者模型
条件变量可以在生产者消费者模型中使用,以协调多个线程之间的工作。
import threading
import time
import queue
q = queue.Queue()
condition = threading.Condition()
def producer():
for i in range(5):
time.sleep(1)
with condition:
q.put(i)
print(f"Produced {i}")
condition.notify() # 通知消费者线程
def consumer():
while True:
with condition:
condition.wait() # 等待通知
item = q.get()
print(f"Consumed {item}")
if item == 4:
break
thread1 = threading.Thread(target=producer)
thread2 = threading.Thread(target=consumer)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
在上面的例子中,生产者线程生产数据,并通知消费者线程消费数据,消费者线程在接收到通知后继续执行。
九、使用进程间通信暂停执行脚本
1、基本用法
可以使用multiprocessing模块中的Queue类实现进程间的通信,使用队列控制进程的暂停和继续执行。
import multiprocessing
import time
def worker(queue):
print("Worker waiting")
queue.get() # 暂停进程,等待队列中的消息
print("Worker resumed")
queue = multiprocessing.Queue()
process = multiprocessing.Process(target=worker, args=(queue,))
process.start()
time.sleep(2)
print("Main process sending message")
queue.put("Resume") # 发送消息,通知等待的进程继续执行
在上面的例子中,worker进程在调用queue.get()后暂停,直到主进程向队列中发送消息。
2、结合生产者消费者模型
进程间通信可以在生产者消费者模型中使用,以协调多个进程之间的工作。
import multiprocessing
import time
def producer(queue):
for i in range(5):
time.sleep(1)
queue.put(i)
print(f"Produced {i}")
def consumer(queue):
while True:
item = queue.get()
print(f"Consumed {item}")
if item == 4:
break
queue = multiprocessing.Queue()
producer_process = multiprocessing.Process(target=producer, args=(queue,))
consumer_process = multiprocessing.Process(target=consumer, args=(queue,))
producer_process.start()
consumer_process.start()
producer_process.join()
consumer_process.join()
在上面的例子中,生产者进程生产数据,并通过队列传递给消费者进程,消费者进程在接收到数据后继续执行。
十、总结
在Python中,有多种方法可以实现暂停执行脚本的功能,包括使用time.sleep()、input()函数、信号处理、线程等待、asyncio实现异步暂停、定时器、事件、条件变量以及进程间通信。每种方法都有其适用的场景和优缺点,可以根据具体需求选择合适的方法。
time.sleep()函数是最常用的暂停执行脚本的方法,它简单易用,适用于大多数需要暂停执行的场景。input()函数适用于等待用户输入,常用于交互式脚本。信号处理适用于处理外部信号,可以在接收到特定信号时暂停执行。线程等待和事件、条件变量适用于多线程编程,可以实现线程之间的同步和协调。asyncio实现异步暂停适用于异步编程,可以实现异步任务的暂停和调度。定时器可以在指定时间后执行特定操作,适用于需要定时执行操作的场景。进程间通信适用于多进程编程,可以实现进程之间的同步和协调。
通过掌握这些方法,我们可以在不同的场景下实现脚本的暂停执行,从而更好地控制程序的执行流程。
相关问答FAQs:
如何在Python中实现脚本暂停?
在Python中,可以使用time
模块的sleep()
函数来暂停脚本的执行。只需导入time
模块,然后调用sleep(seconds)
,其中seconds
是要暂停的时间(以秒为单位)。例如,time.sleep(5)
将使脚本暂停5秒。
在Python中暂停脚本后如何恢复执行?
一旦使用sleep()
函数暂停了脚本的执行,Python会自动在指定的时间后继续执行后面的代码。因此,无需额外的步骤来恢复执行,代码将按顺序继续执行。
除了使用time.sleep()之外,还有其他暂停脚本的方法吗?
是的,除了sleep()
函数,还有其他几种方法可以实现脚本的暂停。例如,可以使用input()
函数等待用户输入,脚本将在此行暂停,直到用户按下回车键。此外,使用信号处理,可以在特定条件下暂停脚本执行。根据需求选择合适的方法。
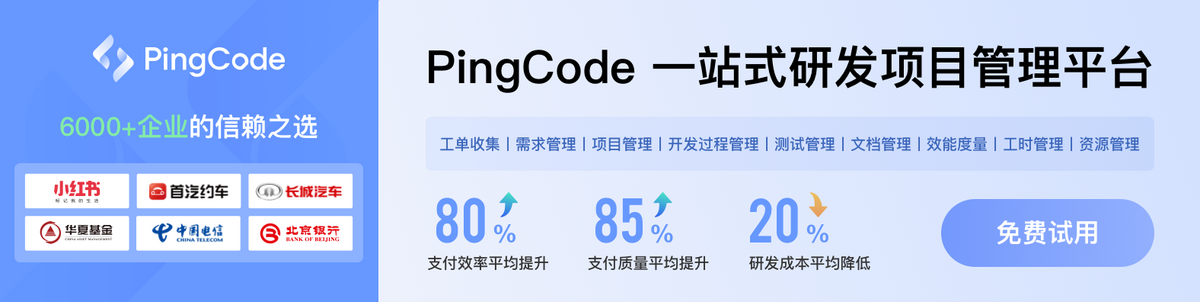