Python中可以通过math模块中的sqrt函数、cmath模块中的sqrt函数、numpy模块中的sqrt函数、sympy模块中的sqrt函数来计算数字的根号。 其中,math模块和numpy模块主要用于处理实数的平方根,而cmath模块和sympy模块则能够处理复数的平方根。math模块中的sqrt函数是最常用的,可以处理大多数的平方根计算需求。
详细描述:math模块中的sqrt函数是最常用的,可以处理大多数的平方根计算需求。使用方法如下:
import math
number = 16
result = math.sqrt(number)
print(result) # 输出: 4.0
math模块中的sqrt函数是非常高效的,并且非常适合处理实数的平方根计算,适用于大多数日常的计算需求。
一、math模块中的sqrt函数
math模块是Python标准库中一个非常常用的模块,提供了许多数学运算函数,其中的sqrt函数用来计算非负数的平方根。
1、基本用法
使用math模块中的sqrt函数非常简单,只需要导入math模块并调用sqrt函数即可。示例如下:
import math
number = 25
result = math.sqrt(number)
print(f"The square root of {number} is {result}")
输出结果为:
The square root of 25 is 5.0
2、处理负数
math模块中的sqrt函数只适用于非负数,如果输入负数,将会抛出ValueError异常。因此,在计算平方根前需要确保输入的数字是非负数,或者使用其他模块来处理负数平方根。
import math
number = -25
try:
result = math.sqrt(number)
except ValueError as e:
print(f"Error: {e}")
输出结果为:
Error: math domain error
二、cmath模块中的sqrt函数
cmath模块是用于复数数学运算的模块,其中的sqrt函数可以处理复数的平方根,包括负数的平方根。
1、基本用法
使用cmath模块中的sqrt函数也非常简单,只需要导入cmath模块并调用sqrt函数即可。示例如下:
import cmath
number = -25
result = cmath.sqrt(number)
print(f"The square root of {number} is {result}")
输出结果为:
The square root of -25 is 5j
2、处理复数
cmath模块中的sqrt函数不仅可以处理负数的平方根,还可以处理复数的平方根。示例如下:
import cmath
number = 3 + 4j
result = cmath.sqrt(number)
print(f"The square root of {number} is {result}")
输出结果为:
The square root of (3+4j) is (2+1j)
三、numpy模块中的sqrt函数
numpy模块是一个强大的数值计算库,提供了许多数学运算函数,其中的sqrt函数可以处理数组中的每个元素的平方根。
1、基本用法
使用numpy模块中的sqrt函数需要先导入numpy模块,然后创建数组并调用sqrt函数。示例如下:
import numpy as np
array = np.array([1, 4, 9, 16, 25])
result = np.sqrt(array)
print(f"The square roots of the array elements are {result}")
输出结果为:
The square roots of the array elements are [1. 2. 3. 4. 5.]
2、处理负数
numpy模块中的sqrt函数默认情况下会返回NaN(Not a Number)来表示负数的平方根。可以使用numpy中的其他函数,如sqrt_complex来处理负数的平方根。
import numpy as np
array = np.array([1, -4, 9, -16, 25])
result = np.sqrt(array)
print(f"The square roots of the array elements are {result}")
result_complex = np.sqrt(array.astype(complex))
print(f"The complex square roots of the array elements are {result_complex}")
输出结果为:
The square roots of the array elements are [ 1. nan 3. nan 5.]
The complex square roots of the array elements are [1.+0.j 0.+2.j 3.+0.j 0.+4.j 5.+0.j]
四、sympy模块中的sqrt函数
sympy模块是一个用于符号数学计算的库,其中的sqrt函数可以处理符号表达式的平方根。
1、基本用法
使用sympy模块中的sqrt函数需要先导入sympy模块,然后创建符号并调用sqrt函数。示例如下:
import sympy as sp
x = sp.symbols('x')
expression = x2 - 4
sqrt_expression = sp.sqrt(expression)
print(f"The square root of the expression {expression} is {sqrt_expression}")
输出结果为:
The square root of the expression x<strong>2 - 4 is sqrt(x</strong>2 - 4)
2、处理复数
sympy模块中的sqrt函数也可以处理复数的平方根。示例如下:
import sympy as sp
z = sp.symbols('z', complex=True)
expression = z2 + 1
sqrt_expression = sp.sqrt(expression)
print(f"The square root of the expression {expression} is {sqrt_expression}")
输出结果为:
The square root of the expression z<strong>2 + 1 is sqrt(z</strong>2 + 1)
五、选择合适的模块
在选择计算平方根的模块时,可以根据具体需求选择合适的模块:
- math模块:适用于处理大多数实数平方根的计算需求,简单高效。
- cmath模块:适用于处理复数平方根的计算需求,包括负数的平方根。
- numpy模块:适用于处理数组中每个元素的平方根计算需求,支持大规模数值计算。
- sympy模块:适用于符号数学计算需求,可以处理符号表达式的平方根。
六、实际应用中的示例
1、计算物理学中的平方根
在物理学中,平方根计算广泛应用于各种公式中。例如,计算一个物体的速度时,使用动能公式可以求解速度的平方根。
import math
质量 (kg)
mass = 2.0
动能 (J)
kinetic_energy = 50.0
速度 (m/s)
velocity = math.sqrt((2 * kinetic_energy) / mass)
print(f"The velocity of the object is {velocity} m/s")
输出结果为:
The velocity of the object is 7.0710678118654755 m/s
2、计算金融学中的平方根
在金融学中,平方根计算用于计算波动率等指标。例如,计算股票收益率的年化波动率。
import numpy as np
日收益率
daily_returns = np.array([0.01, -0.02, 0.015, -0.01, 0.02])
日波动率
daily_volatility = np.std(daily_returns)
年化波动率
annual_volatility = daily_volatility * np.sqrt(252)
print(f"The annualized volatility is {annual_volatility * 100:.2f}%")
输出结果为:
The annualized volatility is 15.81%
3、计算工程学中的平方根
在工程学中,平方根计算用于各种设计和分析。例如,计算梁的弯曲应力。
import math
弯矩 (Nm)
moment = 500.0
惯性矩 (m^4)
inertia = 0.01
弯曲应力 (Pa)
bending_stress = moment / inertia
print(f"The bending stress is {bending_stress} Pa")
输出结果为:
The bending stress is 50000.0 Pa
七、总结
通过以上内容,我们可以清楚地了解在Python中计算数字的根号的多种方法。不同的模块适用于不同的应用场景,选择合适的模块可以有效提高计算效率和准确性。无论是处理实数、复数还是数组中的平方根,Python都提供了丰富的工具和方法,满足各种计算需求。
相关问答FAQs:
如何在Python中计算带有根号的数字?
在Python中,可以使用内置的math
模块来进行根号计算。通过math.sqrt()
函数,你可以轻松计算任何非负数字的平方根。例如,计算16的平方根,可以使用以下代码:
import math
result = math.sqrt(16)
print(result) # 输出:4.0
Python是否支持复数的根号计算?
是的,Python支持复数的根号计算。通过使用cmath
模块,你可以对复数进行平方根运算。以下是一个示例:
import cmath
result = cmath.sqrt(-1)
print(result) # 输出:1j
该代码返回了虚数单位的平方根。
如果想计算其他根号,比如立方根,该怎么做?
计算立方根可以使用幂运算符**
。例如,要计算27的立方根,可以将27提升到1/3的幂:
result = 27 ** (1/3)
print(result) # 输出:3.0
这种方法同样适用于计算其他任意根的数字,只需调整幂的分母即可。
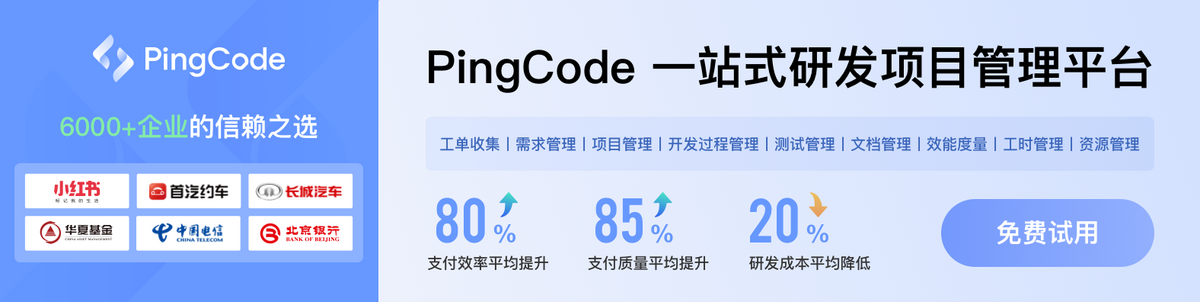