要用Python获取NPC位置,可以通过多种方式实现,这取决于您所使用的游戏引擎或平台。通过API调用、解析日志文件、使用图像识别等方法都可以达到目的。下面将详细介绍如何通过API调用来获取NPC位置。
一、通过API调用获取NPC位置
1、理解API
许多现代游戏都会提供API接口,允许开发者获取游戏中的各种数据,包括NPC的位置。通常,这些API接口会返回JSON格式的数据,Python可以轻松解析这些数据。
2、使用requests库调用API
首先,您需要找到API的URL和必要的参数。假设我们有一个游戏API,它提供了一个端点来获取NPC的位置。以下是一个示例代码:
import requests
def get_npc_position(npc_id):
url = f"https://api.examplegame.com/npc/{npc_id}/position"
response = requests.get(url)
if response.status_code == 200:
data = response.json()
return data['position']
else:
print(f"Failed to get NPC position: {response.status_code}")
return None
npc_id = 123
position = get_npc_position(npc_id)
print(f"NPC position: {position}")
在这个示例中,我们使用requests
库发送GET请求到API端点,并解析返回的JSON数据以获取NPC的位置。API的调用和数据解析是获取NPC位置的核心步骤。
3、处理错误和异常
在实际应用中,您可能会遇到各种网络错误或API错误。为了确保您的程序稳健,您需要处理这些错误和异常。
import requests
def get_npc_position(npc_id):
url = f"https://api.examplegame.com/npc/{npc_id}/position"
try:
response = requests.get(url)
response.raise_for_status() # Raises an HTTPError if the HTTP request returned an unsuccessful status code
data = response.json()
return data['position']
except requests.exceptions.HTTPError as http_err:
print(f"HTTP error occurred: {http_err}")
except Exception as err:
print(f"Other error occurred: {err}")
return None
npc_id = 123
position = get_npc_position(npc_id)
if position:
print(f"NPC position: {position}")
else:
print("Failed to retrieve NPC position.")
二、解析游戏日志文件获取NPC位置
某些游戏会将NPC的位置记录在日志文件中。通过解析这些日志文件,您也可以获取NPC的位置。
1、读取日志文件
首先,您需要找到游戏日志文件的位置,并读取文件内容。
def read_log_file(file_path):
with open(file_path, 'r') as file:
log_data = file.readlines()
return log_data
2、解析日志内容
假设日志文件中包含类似于以下的行:
[NPC] ID: 123 Position: (100, 200, 300)
您可以编写代码来解析这些行并提取NPC的位置。
import re
def parse_npc_position(log_data, npc_id):
pattern = re.compile(rf'\[NPC\] ID: {npc_id} Position: \((\d+), (\d+), (\d+)\)')
for line in log_data:
match = pattern.search(line)
if match:
x, y, z = match.groups()
return int(x), int(y), int(z)
return None
log_data = read_log_file('game.log')
npc_id = 123
position = parse_npc_position(log_data, npc_id)
if position:
print(f"NPC position: {position}")
else:
print("NPC position not found in log.")
三、使用图像识别获取NPC位置
在某些情况下,您可能无法直接获取NPC位置数据,这时可以使用图像识别技术来获取NPC的位置。
1、安装所需库
首先,您需要安装OpenCV和其他必要的库:
pip install opencv-python
2、捕获屏幕图像
您需要捕获游戏的屏幕图像以进行处理。可以使用pyautogui
库来实现:
import pyautogui
screenshot = pyautogui.screenshot()
screenshot.save('screenshot.png')
3、使用模板匹配
假设您有一个NPC的模板图像,您可以使用模板匹配来找到NPC的位置:
import cv2
import numpy as np
def find_npc_position(screenshot_path, template_path):
screenshot = cv2.imread(screenshot_path)
template = cv2.imread(template_path, 0)
screenshot_gray = cv2.cvtColor(screenshot, cv2.COLOR_BGR2GRAY)
result = cv2.matchTemplate(screenshot_gray, template, cv2.TM_CCOEFF_NORMED)
min_val, max_val, min_loc, max_loc = cv2.minMaxLoc(result)
if max_val >= 0.8: # Assuming a threshold of 0.8 for match quality
return max_loc
else:
return None
screenshot_path = 'screenshot.png'
template_path = 'npc_template.png'
npc_position = find_npc_position(screenshot_path, template_path)
if npc_position:
print(f"NPC position: {npc_position}")
else:
print("NPC not found in screenshot.")
在这个示例中,我们使用OpenCV的模板匹配功能来找到NPC的位置。图像识别技术在某些情况下是获取NPC位置的有效方法。
四、结合多种方法获取更准确的NPC位置
在实际应用中,您可能需要结合多种方法来获取更准确的NPC位置。例如,您可以首先使用API获取大致位置,然后使用图像识别技术进行精确定位。
1、综合方法示例
def get_npc_position_combined(api_url, npc_id, screenshot_path, template_path):
api_position = get_npc_position_from_api(api_url, npc_id)
if api_position:
print(f"API provided position: {api_position}")
# Use API position to narrow down the search area in the screenshot
screenshot = pyautogui.screenshot()
screenshot.save(screenshot_path)
npc_position = find_npc_position(screenshot_path, template_path)
if npc_position:
print(f"Final NPC position: {npc_position}")
return npc_position
else:
print("Failed to get position from API, using only image recognition.")
npc_position = find_npc_position(screenshot_path, template_path)
return npc_position
api_url = "https://api.examplegame.com/npc"
npc_id = 123
screenshot_path = 'screenshot.png'
template_path = 'npc_template.png'
npc_position = get_npc_position_combined(api_url, npc_id, screenshot_path, template_path)
if npc_position:
print(f"NPC position: {npc_position}")
else:
print("NPC position not found.")
在这个综合示例中,我们首先尝试通过API获取NPC的位置,然后使用图像识别技术进一步确定NPC的精确位置。这种综合方法能够提高NPC位置获取的准确性和可靠性。
五、总结
通过API调用、解析日志文件、使用图像识别技术,我们可以实现用Python获取NPC位置的目标。每种方法都有其优缺点,具体选择哪种方法应根据实际情况而定。在实际应用中,结合多种方法往往能取得更好的效果。
API调用:优点是简单直接,缺点是依赖于游戏是否提供API支持。
解析日志文件:优点是可行性高,缺点是需要了解日志文件格式和结构。
图像识别技术:优点是适用范围广,缺点是需要处理图像数据,可能比较复杂。
通过上述方法的详细介绍和示例代码,希望能帮助您更好地理解和实现用Python获取NPC位置的功能。
相关问答FAQs:
如何用Python获取游戏中的NPC位置?
要获取游戏中的NPC位置,可以使用游戏的API或数据结构。如果游戏提供了Python接口,您可以通过调用相应的函数来获取NPC的坐标信息。此外,您还可以通过解析游戏内存或使用游戏引擎提供的功能来获取这些数据。确保您了解游戏的开发文档,以便找到适合的方式获取NPC位置。
获取NPC位置时需要注意哪些事项?
在获取NPC位置时,注意游戏的更新频率和位置变化可能会影响获取到的数据的准确性。有些游戏会在每帧更新位置,因此在处理时需要考虑到这些变化。此外,确保遵守游戏的使用条款,避免使用不当手段获取信息。
是否可以使用Python脚本自动化获取NPC位置?
是的,您可以编写Python脚本来自动化获取NPC位置。如果游戏提供了Python SDK或API,您可以通过这些工具编写脚本定期检查NPC的位置。利用定时任务和网络请求等功能,可以实现自动监测和记录NPC的动态位置。确保按照游戏规则进行操作,以免影响游戏体验。
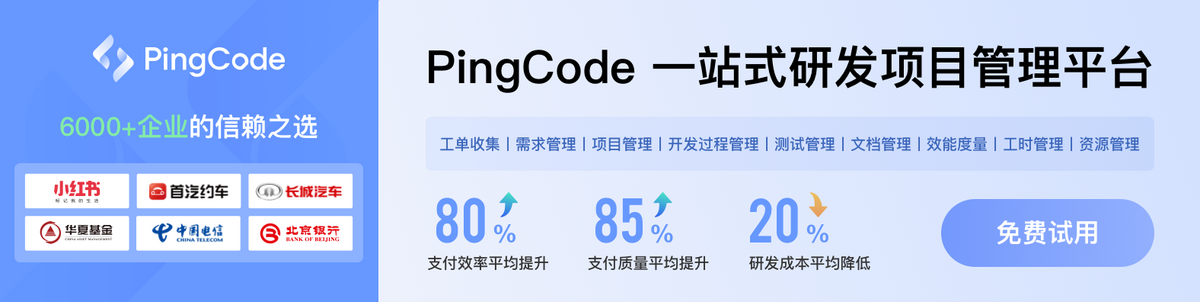