在Python中,打开程序文件的方法有很多种,主要包括使用内置的open
函数、使用with
语句管理文件上下文、使用不同模式读取或写入文件内容。使用内置的open
函数是最基本的方法,我们可以通过这种方法打开文件并进行读写操作。
例如,使用open
函数打开一个文件并读取其内容,可以按如下方式进行:
file = open('example.txt', 'r')
content = file.read()
print(content)
file.close()
在这个例子中,我们首先使用open
函数以读取模式('r')打开名为example.txt
的文件,然后使用read
方法读取文件的全部内容并打印出来,最后使用close
方法关闭文件。
接下来,我们将详细介绍不同的文件操作方式以及各种模式的使用。
一、使用内置的open
函数
内置的open
函数是Python中用于打开文件的最基础工具。使用open
函数可以指定文件名和模式,打开文件后返回一个文件对象,接着可以对文件对象进行各种操作。
1.1 打开文件的基本方法
open
函数的基本语法如下:
open(file, mode='r', buffering=-1, encoding=None, errors=None, newline=None, closefd=True, opener=None)
其中,常用的参数包括:
file
:要打开的文件名(包括路径)。mode
:文件打开模式,常见的模式有:'r'
:只读模式(默认)。'w'
:写入模式,会覆盖文件内容。'a'
:追加模式,写入内容会追加到文件末尾。'b'
:以二进制模式打开文件。't'
:以文本模式打开文件(默认)。'+'
:读写模式。
1.2 文件的读取操作
打开文件后,可以使用不同的方法读取文件内容:
read(size)
:读取指定大小的内容,若未指定大小则读取全部内容。readline()
:读取一行内容。readlines()
:读取所有行并返回列表。
例如:
file = open('example.txt', 'r')
content = file.read()
print(content)
file.close()
1.3 文件的写入操作
打开文件后,可以使用不同的方法写入文件内容:
write(string)
:写入字符串内容。writelines(lines)
:写入多个字符串行。
例如:
file = open('example.txt', 'w')
file.write('Hello, World!')
file.close()
二、使用with
语句管理文件上下文
为了简化文件的打开和关闭操作,Python提供了with
语句来管理文件上下文。使用with
语句可以确保文件在使用完毕后自动关闭,无需显式调用close
方法。
2.1 with
语句的基本用法
with
语句的基本语法如下:
with open(file, mode) as file_object:
# 对文件进行操作
例如:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
2.2 with
语句的优势
使用with
语句管理文件上下文有以下优势:
- 简洁性:代码更简洁,无需显式调用
close
方法。 - 安全性:确保文件在使用完毕后自动关闭,即使发生异常也能正确关闭文件,避免资源泄漏。
例如:
with open('example.txt', 'w') as file:
file.write('Hello, World!')
三、文件操作模式详解
不同的文件操作模式适用于不同的场景,我们需要根据具体需求选择合适的模式。
3.1 只读模式('r')
只读模式用于读取文件内容,文件必须存在,否则会引发异常。
例如:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
3.2 写入模式('w')
写入模式用于写入文件内容,文件不存在时会创建新文件,文件存在时会覆盖原有内容。
例如:
with open('example.txt', 'w') as file:
file.write('Hello, World!')
3.3 追加模式('a')
追加模式用于在文件末尾追加内容,文件不存在时会创建新文件。
例如:
with open('example.txt', 'a') as file:
file.write('Hello, again!')
3.4 二进制模式('b')
二进制模式用于读取或写入二进制文件,例如图片或音频文件。
例如:
with open('example.jpg', 'rb') as file:
content = file.read()
# 处理二进制内容
3.5 读写模式('+')
读写模式允许同时进行读写操作,根据具体需求选择合适的组合模式('r+'、'w+'、'a+')。
例如:
with open('example.txt', 'r+') as file:
content = file.read()
print(content)
file.write('Hello, World!')
四、文件指针与文件操作
文件指针用于标记当前文件读取或写入的位置,理解文件指针的操作对文件处理非常重要。
4.1 文件指针的基本操作
tell()
:返回文件指针的当前位置。seek(offset, whence)
:移动文件指针到指定位置。
例如:
with open('example.txt', 'r') as file:
content = file.read(5)
print(content)
print(file.tell())
file.seek(0)
print(file.read())
4.2 文件指针的实际应用
文件指针的操作在处理大文件时非常有用,例如:
with open('example.txt', 'r') as file:
while True:
line = file.readline()
if not line:
break
print(line.strip())
if file.tell() > 100:
break
五、文件和目录操作
除了基本的文件读写操作外,Python还提供了一些用于操作文件和目录的模块和函数。
5.1 os
模块
os
模块提供了丰富的文件和目录操作函数,例如:
os.path.exists(path)
:检查文件或目录是否存在。os.makedirs(path)
:递归创建目录。os.remove(path)
:删除文件。os.rename(src, dst)
:重命名文件或目录。
例如:
import os
if not os.path.exists('example_dir'):
os.makedirs('example_dir')
with open('example_dir/example.txt', 'w') as file:
file.write('Hello, World!')
os.rename('example_dir/example.txt', 'example_dir/renamed.txt')
os.remove('example_dir/renamed.txt')
os.rmdir('example_dir')
5.2 shutil
模块
shutil
模块提供了高级的文件和目录操作函数,例如:
shutil.copy(src, dst)
:复制文件。shutil.copytree(src, dst)
:递归复制目录。shutil.move(src, dst)
:移动文件或目录。shutil.rmtree(path)
:递归删除目录。
例如:
import shutil
with open('example.txt', 'w') as file:
file.write('Hello, World!')
shutil.copy('example.txt', 'example_copy.txt')
shutil.move('example_copy.txt', 'example_moved.txt')
shutil.rmtree('example_dir')
六、处理CSV文件
CSV(Comma-Separated Values)文件是一种常见的数据存储格式,Python提供了csv
模块用于处理CSV文件。
6.1 读取CSV文件
读取CSV文件的基本方法如下:
import csv
with open('example.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
6.2 写入CSV文件
写入CSV文件的基本方法如下:
import csv
with open('example.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Name', 'Age'])
writer.writerow(['Alice', 30])
writer.writerow(['Bob', 25])
6.3 使用字典读取和写入CSV文件
csv.DictReader
和csv.DictWriter
类允许使用字典读取和写入CSV文件。
例如:
import csv
with open('example.csv', 'r') as file:
reader = csv.DictReader(file)
for row in reader:
print(row)
with open('example.csv', 'w', newline='') as file:
fieldnames = ['Name', 'Age']
writer = csv.DictWriter(file, fieldnames=fieldnames)
writer.writeheader()
writer.writerow({'Name': 'Alice', 'Age': 30})
writer.writerow({'Name': 'Bob', 'Age': 25})
七、处理JSON文件
JSON(JavaScript Object Notation)是一种轻量级的数据交换格式,Python提供了json
模块用于处理JSON文件。
7.1 读取JSON文件
读取JSON文件的基本方法如下:
import json
with open('example.json', 'r') as file:
data = json.load(file)
print(data)
7.2 写入JSON文件
写入JSON文件的基本方法如下:
import json
data = {'Name': 'Alice', 'Age': 30}
with open('example.json', 'w') as file:
json.dump(data, file)
7.3 处理复杂的JSON数据
处理复杂的JSON数据时,可以使用自定义编码器和解码器。
例如:
import json
from datetime import datetime
class CustomEncoder(json.JSONEncoder):
def default(self, obj):
if isinstance(obj, datetime):
return obj.isoformat()
return super().default(obj)
data = {'Name': 'Alice', 'Age': 30, 'Date': datetime.now()}
with open('example.json', 'w') as file:
json.dump(data, file, cls=CustomEncoder)
with open('example.json', 'r') as file:
data = json.load(file)
print(data)
八、处理XML文件
XML(eXtensible Markup Language)是一种常见的数据交换格式,Python提供了xml.etree.ElementTree
模块用于处理XML文件。
8.1 读取XML文件
读取XML文件的基本方法如下:
import xml.etree.ElementTree as ET
tree = ET.parse('example.xml')
root = tree.getroot()
for child in root:
print(child.tag, child.attrib)
8.2 写入XML文件
写入XML文件的基本方法如下:
import xml.etree.ElementTree as ET
root = ET.Element('root')
child = ET.SubElement(root, 'child', {'name': 'Alice'})
child.text = 'Hello, World!'
tree = ET.ElementTree(root)
tree.write('example.xml')
8.3 处理复杂的XML数据
处理复杂的XML数据时,可以使用更多的XML操作函数,例如:
import xml.etree.ElementTree as ET
tree = ET.parse('example.xml')
root = tree.getroot()
for child in root:
if child.tag == 'child' and child.attrib.get('name') == 'Alice':
child.text = 'Hello, again!'
tree.write('example.xml')
九、处理Excel文件
Excel文件是一种常见的数据存储格式,Python提供了openpyxl
模块用于处理Excel文件。
9.1 读取Excel文件
读取Excel文件的基本方法如下:
import openpyxl
workbook = openpyxl.load_workbook('example.xlsx')
sheet = workbook.active
for row in sheet.iter_rows(values_only=True):
print(row)
9.2 写入Excel文件
写入Excel文件的基本方法如下:
import openpyxl
workbook = openpyxl.Workbook()
sheet = workbook.active
sheet['A1'] = 'Name'
sheet['B1'] = 'Age'
sheet.append(['Alice', 30])
sheet.append(['Bob', 25])
workbook.save('example.xlsx')
9.3 处理复杂的Excel数据
处理复杂的Excel数据时,可以使用更多的Excel操作函数,例如:
import openpyxl
workbook = openpyxl.load_workbook('example.xlsx')
sheet = workbook.active
for row in sheet.iter_rows(min_row=2, values_only=True):
name, age = row
if name == 'Alice':
sheet.cell(row=row[0].row, column=2, value=31)
workbook.save('example.xlsx')
十、处理PDF文件
PDF文件是一种常见的文档格式,Python提供了PyPDF2
模块用于处理PDF文件。
10.1 读取PDF文件
读取PDF文件的基本方法如下:
import PyPDF2
with open('example.pdf', 'rb') as file:
reader = PyPDF2.PdfFileReader(file)
for page_num in range(reader.numPages):
page = reader.getPage(page_num)
print(page.extractText())
10.2 写入PDF文件
写入PDF文件的基本方法如下:
import PyPDF2
pdf_writer = PyPDF2.PdfFileWriter()
pdf_writer.addBlankPage(width=72, height=72)
with open('example.pdf', 'wb') as file:
pdf_writer.write(file)
10.3 处理复杂的PDF数据
处理复杂的PDF数据时,可以使用更多的PDF操作函数,例如:
import PyPDF2
pdf_writer = PyPDF2.PdfFileWriter()
with open('example.pdf', 'rb') as file:
reader = PyPDF2.PdfFileReader(file)
for page_num in range(reader.numPages):
page = reader.getPage(page_num)
pdf_writer.addPage(page)
with open('example_copy.pdf', 'wb') as file:
pdf_writer.write(file)
十一、处理二进制文件
二进制文件是一种包含非文本数据的文件,Python提供了多种处理二进制文件的方法。
11.1 读取二进制文件
读取二进制文件的基本方法如下:
with open('example.bin', 'rb') as file:
data = file.read()
print(data)
11.2 写入二进制文件
写入二进制文件的基本方法如下:
with open('example.bin', 'wb') as file:
file.write(b'\x00\x01\x02\x03')
11.3 处理复杂的二进制数据
处理复杂的二进制数据时,可以使用更多的二进制操作函数,例如:
import struct
data = struct.pack('i', 12345)
with open('example.bin', 'wb') as file:
file.write(data)
with open('example.bin', 'rb') as file:
data = file.read()
value = struct.unpack('i', data)[0]
print(value)
总结起来,Python提供了丰富的文件操作方法和模块,可以满足各种文件处理需求。通过合理选择和使用这些方法和模块,可以高效地进行文件操作和数据处理。
相关问答FAQs:
如何在Python中打开和读取文件?
要在Python中打开和读取文件,可以使用内置的open()
函数。这个函数允许你指定文件的路径和打开模式(如读取、写入等)。例如,使用with open('file.txt', 'r') as file:
语句可以安全地打开文件并在操作完成后自动关闭它。读取文件内容可以使用file.read()
、file.readline()
或file.readlines()
等方法,根据你的需求选择合适的读取方式。
Python支持哪些文件打开模式?
Python支持多种文件打开模式,包括:
'r'
:只读模式,文件必须存在。'w'
:写入模式,如果文件存在则覆盖,如果不存在则创建新文件。'a'
:追加模式,写入内容将添加到现有文件末尾。'b'
:二进制模式,可以与其他模式结合使用,适用于非文本文件。'x'
:独占写入模式,文件必须不存在,创建新文件。
如何处理文件打开过程中可能出现的异常?
在打开文件时,可能会遇到文件不存在、权限不足等问题。可以使用try...except
结构来捕获和处理这些异常。例如,使用try:
尝试打开文件,并在except FileNotFoundError:
中处理文件未找到的错误。这样可以确保程序在遇到问题时不会崩溃,并能够提供用户友好的错误信息。
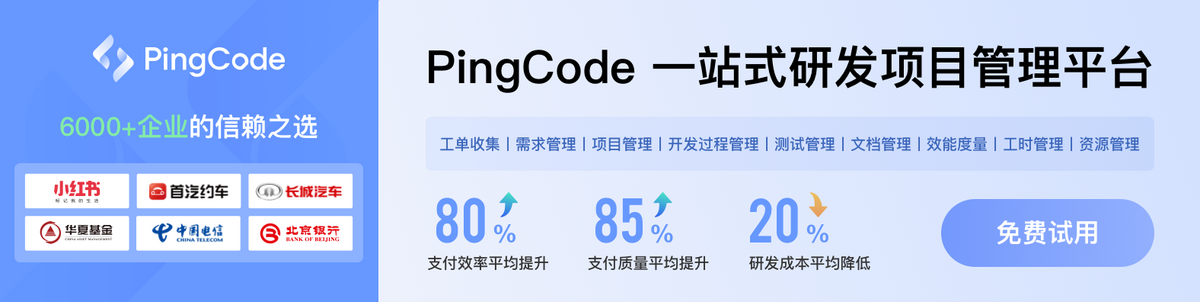