Python可以通过使用图形库来绘制图形、生成艺术作品、进行数据可视化和图形处理。常用的库包括Matplotlib、Turtle、Pygame、PIL(Pillow)等。其中,Matplotlib主要用于数据可视化,Turtle适合教学和简单绘图,Pygame用于游戏开发和复杂图形处理,而PIL则用于图像处理。下面将详细介绍如何使用这些工具进行绘图。
一、MATPLOTLIB绘图
Matplotlib是Python中最常用的绘图库之一,常用于数据可视化。通过简单的代码,我们可以创建各种2D图形,如折线图、柱状图、散点图等。
-
安装和基础用法
要使用Matplotlib,首先需要安装它,可以通过pip命令来安装:
pip install matplotlib
安装完成后,可以开始使用它进行简单的绘图。以下是一个基本的折线图示例:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
plt.plot(x, y)
plt.title('Simple Line Plot')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.show()
这段代码创建了一个简单的折线图,显示了X轴和Y轴上的数据关系。
-
高级用法
Matplotlib不仅能够创建简单的图形,还支持复杂的定制化图形。可以使用子图、网格、注释等功能来增强图形的表现力。
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.figure(figsize=(10, 5))
plt.plot(x, y, label='Sine Wave', color='blue', linestyle='--')
plt.title('Advanced Plot Example')
plt.xlabel('Angle [radians]')
plt.ylabel('sin(x)')
plt.legend()
plt.grid(True)
plt.show()
这里使用了numpy生成数据,并在图中添加了图例、网格和自定义样式。
二、TURTLE绘图
Turtle是Python内置的一个绘图库,主要用于教学和简单图形的绘制。它通过模拟海龟在屏幕上行走来绘制图形。
-
基础用法
Turtle非常适合初学者进行简单的图形绘制。首先,导入turtle模块并创建一个画布。
import turtle
screen = turtle.Screen()
pen = turtle.Turtle()
pen.forward(100)
pen.left(90)
pen.forward(100)
pen.left(90)
pen.forward(100)
pen.left(90)
pen.forward(100)
screen.mainloop()
这段代码绘制了一个简单的正方形。
-
复杂图形绘制
Turtle也可以绘制复杂的图形,比如螺旋、星形等。通过循环和函数组合,可以实现更复杂的图案。
import turtle
pen = turtle.Turtle()
for i in range(36):
pen.forward(100)
pen.left(140)
pen.forward(100)
pen.left(10)
turtle.done()
这段代码通过循环绘制了一个复杂的星形图案。
三、PYGAME绘图
Pygame是一个用于游戏开发的Python库,但它也可以用于创建复杂的2D图形和动画。
-
安装和基本绘图
首先安装Pygame:
pip install pygame
然后,创建一个简单的绘图窗口。
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((400, 300))
pygame.display.set_caption('Simple Pygame Drawing')
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.fill((255, 255, 255))
pygame.draw.circle(screen, (0, 0, 255), (200, 150), 75)
pygame.display.flip()
pygame.quit()
sys.exit()
这个示例创建了一个窗口,并在其中绘制了一个蓝色的圆。
-
动画和交互
Pygame不仅可以绘制静态图形,还可以处理动画和用户交互。
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((400, 300))
pygame.display.set_caption('Pygame Animation')
x, y = 200, 150
dx, dy = 2, 2
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
x += dx
y += dy
if x < 0 or x > 400:
dx = -dx
if y < 0 or y > 300:
dy = -dy
screen.fill((255, 255, 255))
pygame.draw.circle(screen, (0, 0, 255), (x, y), 20)
pygame.display.flip()
pygame.time.delay(30)
pygame.quit()
sys.exit()
这段代码展示了一个简单的动画,蓝色圆在窗口中反弹。
四、PIL(Pillow)绘图
PIL(Python Imaging Library)是一个用于图像处理的库,Pillow是它的一个分支,提供了更多功能。
-
基础图像操作
Pillow可以用来打开、操作和保存图像文件。
from PIL import Image, ImageDraw
img = Image.new('RGB', (200, 200), (255, 255, 255))
draw = ImageDraw.Draw(img)
draw.rectangle([50, 50, 150, 150], fill=(255, 0, 0))
img.show()
这段代码创建了一个新的图像并在其中绘制了一个红色矩形。
-
高级图像处理
Pillow还支持更高级的图像处理功能,如图像滤镜、变换、文本绘制等。
from PIL import Image, ImageFilter
img = Image.open('example.jpg')
blurred_img = img.filter(ImageFilter.BLUR)
blurred_img.show()
这段代码打开一个图像文件并应用模糊滤镜。
通过以上介绍,我们可以看到Python拥有丰富的图形绘制能力,不论是简单的教学工具还是复杂的游戏开发,Python都能提供相应的解决方案。选择合适的绘图库,可以帮助我们更好地实现图形相关的任务。
相关问答FAQs:
如何在Python中绘制简单的图形?
在Python中,您可以使用多个库来绘制图形,比如Matplotlib和Turtle。Matplotlib适合绘制数据可视化图表,而Turtle更适合绘制简单的图形和动画。使用Turtle库时,只需导入该库并调用相应的绘图函数,即可轻松创建线条、圆形和多边形等图形。
Python中有哪些绘图库推荐?
除了Matplotlib和Turtle外,您还可以考虑使用Pygame和Pillow。Pygame是一个用于游戏开发的库,支持复杂的图形和动画。Pillow则是一个图像处理库,允许您创建和编辑图像,适合需要图像处理功能的项目。
如何在Python中使用Matplotlib绘制图形?
使用Matplotlib绘图的基本步骤包括导入库、定义数据、调用绘图函数并显示结果。例如,您可以使用plt.plot()
函数绘制折线图,或使用plt.bar()
绘制柱状图。设置图形标题和标签也是很简单的,使用plt.title()
和plt.xlabel()
可以增强图形的可读性。
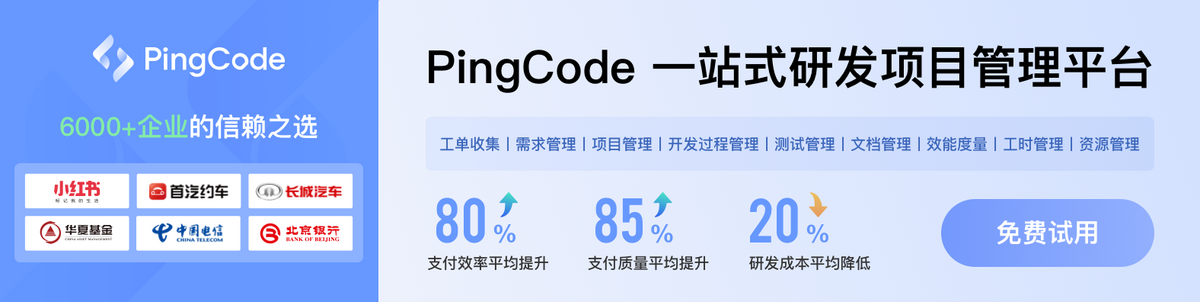