在Python中实现全屏显示,可以通过使用不同的GUI库如Tkinter、Pygame、PyQt等来实现。不同的库有不同的实现方法,其中最常用的方式包括修改窗口属性、使用全屏方法等。以下将详细介绍如何在这些库中实现全屏,以及每种方法的细节。
一、TKINTER实现全屏
Tkinter是Python自带的标准GUI库,非常适合用来创建简单的GUI应用程序。要在Tkinter中实现全屏显示,可以通过修改窗口属性和使用attributes
方法来实现。
-
使用
attributes
方法在Tkinter中,可以通过设置
attributes('-fullscreen', True)
来实现全屏显示。这种方法简单直接,只需在创建窗口后调用该方法即可。import tkinter as tk
root = tk.Tk()
root.attributes('-fullscreen', True)
root.mainloop()
在这段代码中,
root
是Tkinter窗口的实例,通过调用attributes
方法并传递参数'-fullscreen', True
,窗口就会以全屏模式显示。 -
绑定键盘事件退出全屏
使用全屏模式时,通常需要提供一种方式来退出全屏。可以通过绑定键盘事件来实现,例如按下“Escape”键退出全屏。
import tkinter as tk
def toggle_fullscreen(event=None):
root.attributes('-fullscreen', not root.attributes('-fullscreen'))
def end_fullscreen(event=None):
root.attributes('-fullscreen', False)
root = tk.Tk()
root.bind("<F11>", toggle_fullscreen)
root.bind("<Escape>", end_fullscreen)
root.attributes('-fullscreen', True)
root.mainloop()
在这段代码中,按下F11键可以在全屏和窗口模式之间切换,而按下Escape键可以退出全屏。
二、Pygame实现全屏
Pygame是一个用于开发2D游戏的库,在游戏开发中,全屏模式是常见需求。Pygame提供了一种简单的方法来实现全屏。
-
使用
display.set_mode
方法在Pygame中,可以通过
pygame.display.set_mode
方法并传递pygame.FULLSCREEN
参数来实现全屏。import pygame
pygame.init()
screen = pygame.display.set_mode((0, 0), pygame.FULLSCREEN)
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN and event.key == pygame.K_ESCAPE:
running = False
pygame.quit()
在这段代码中,
pygame.display.set_mode((0, 0), pygame.FULLSCREEN)
创建了一个全屏窗口。通过监听键盘事件,可以按下Escape键退出程序。 -
动态切换全屏
在游戏过程中,有时需要在全屏和窗口模式之间切换。可以通过重新调用
set_mode
方法来实现。import pygame
pygame.init()
screen = pygame.display.set_mode((800, 600))
fullscreen = False
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_f:
fullscreen = not fullscreen
if fullscreen:
screen = pygame.display.set_mode((0, 0), pygame.FULLSCREEN)
else:
screen = pygame.display.set_mode((800, 600))
pygame.quit()
在这段代码中,按下F键可以在全屏和窗口模式之间切换。
三、PyQt实现全屏
PyQt是一个功能强大的GUI库,适用于开发复杂的桌面应用程序。要在PyQt中实现全屏,可以使用showFullScreen
方法。
-
使用
showFullScreen
方法在PyQt中,通过调用
showFullScreen
方法可以让窗口以全屏模式显示。from PyQt5.QtWidgets import QApplication, QMainWindow
import sys
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.showFullScreen()
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
在这段代码中,
MainWindow
类继承自QMainWindow
,并在初始化时调用showFullScreen
方法,使窗口以全屏模式显示。 -
动态切换全屏
在PyQt中,可以通过检查窗口状态并调用
showNormal
或showFullScreen
来切换全屏状态。from PyQt5.QtWidgets import QApplication, QMainWindow, QAction
import sys
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.fullscreen = True
self.toggleAction = QAction("Toggle Fullscreen", self)
self.toggleAction.triggered.connect(self.toggle_fullscreen)
self.menuBar().addAction(self.toggleAction)
self.showFullScreen()
def toggle_fullscreen(self):
if self.fullscreen:
self.showNormal()
else:
self.showFullScreen()
self.fullscreen = not self.fullscreen
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
在这段代码中,通过菜单项的点击事件来切换全屏状态。调用
showNormal
可以退出全屏,而showFullScreen
可以进入全屏。
四、其他库的全屏实现
除了Tkinter、Pygame和PyQt,还有其他库可以实现全屏显示,如Kivy、wxPython等。以下简单介绍这些库的全屏实现方法。
-
Kivy
Kivy是一个开源的Python库,用于开发多点触控应用程序。要在Kivy中实现全屏,可以修改配置文件或在代码中设置窗口模式。
from kivy.app import App
from kivy.uix.label import Label
from kivy.core.window import Window
class MyApp(App):
def build(self):
Window.fullscreen = 'auto'
return Label(text='Hello, World!')
if __name__ == '__main__':
MyApp().run()
在这段代码中,通过设置
Window.fullscreen
为'auto'
,应用程序将以全屏模式启动。 -
wxPython
wxPython是Python的另一个GUI库,提供了丰富的控件和功能。在wxPython中,可以通过设置窗口样式来实现全屏。
import wx
class MyFrame(wx.Frame):
def __init__(self, parent, title):
super(MyFrame, self).__init__(parent, title=title, size=(800, 600))
self.ShowFullScreen(True)
app = wx.App(False)
frame = MyFrame(None, "Full Screen Example")
frame.Show()
app.MainLoop()
在这段代码中,
ShowFullScreen(True)
使窗口以全屏模式显示。
通过以上介绍,可以看出在Python中实现全屏显示的方法多种多样,选择合适的库和方法可以根据具体的应用需求来决定。无论是创建简单的GUI应用还是开发复杂的游戏和桌面应用,Python都能提供灵活的全屏解决方案。
相关问答FAQs:
如何在Python中实现全屏模式?
在Python中实现全屏模式通常涉及使用图形用户界面(GUI)库,如Tkinter、Pygame或PyQt。以Tkinter为例,您可以通过设置窗口的几何属性来使窗口全屏。具体而言,可以使用attributes('-fullscreen', True)
方法来将窗口设置为全屏状态。
使用Pygame如何设置全屏显示?
在Pygame中,可以通过在创建窗口时指定pygame.FULLSCREEN
标志来实现全屏显示。例如,您可以使用screen = pygame.display.set_mode((0, 0), pygame.FULLSCREEN)
来创建一个全屏窗口。这种方法会使窗口覆盖整个屏幕。
全屏模式下如何处理退出事件?
在全屏模式下处理退出事件非常重要。以Tkinter为例,您可以绑定窗口的关闭事件,通过设置一个退出函数来处理。例如,可以使用window.protocol("WM_DELETE_WINDOW", exit_function)
来确保在用户尝试关闭全屏窗口时能够优雅地退出程序。对于Pygame,可以监听QUIT
事件并相应地退出游戏。
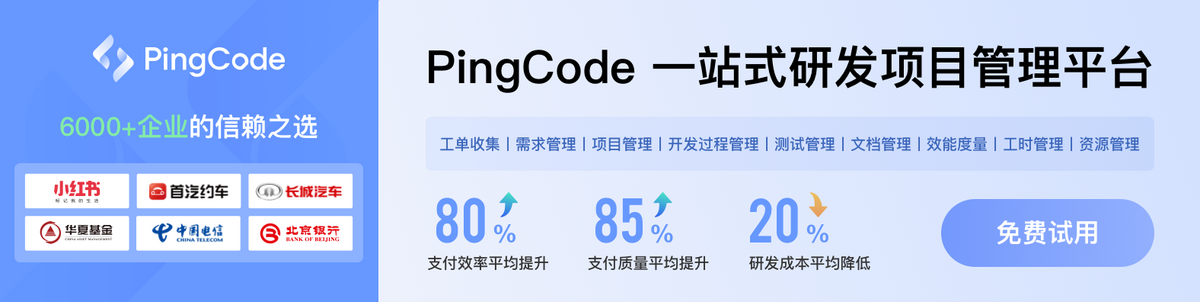