Python 使用代理的方式有多种,包括配置 HTTP 请求头、利用第三方库、以及使用 VPN 等方法。最常用的方法是通过请求库(如 Requests、urllib 等)设置代理服务器、处理 HTTP 和 HTTPS 请求的代理。有的工具还支持 SOCKS 代理。下面将详细介绍其中一种方法,通过 Python Requests 库来使用代理。
一、使用 Requests 库设置 HTTP 和 HTTPS 代理
Requests 是 Python 中一个非常流行的库,用于发送 HTTP 请求。它支持通过代理服务器发送请求,这在需要隐藏真实 IP 或绕过网络限制时非常有用。
1. 安装 Requests 库
首先,确保你已经安装了 Requests 库。如果没有安装,可以通过 pip 进行安装:
pip install requests
2. 设置代理
可以通过传递一个字典给 requests.get()
或 requests.post()
等方法的 proxies
参数来设置代理。字典的键是协议(如 'http' 或 'https'),值是代理的 URL。
import requests
proxies = {
'http': 'http://10.10.1.10:3128',
'https': 'http://10.10.1.10:1080',
}
response = requests.get('http://example.com', proxies=proxies)
print(response.text)
3. 使用代理进行身份验证
如果你的代理服务器需要身份验证,可以在代理 URL 中包含用户名和密码:
proxies = {
'http': 'http://user:password@10.10.1.10:3128',
'https': 'http://user:password@10.10.1.10:1080',
}
二、使用 urllib 库设置代理
urllib 是 Python 的标准库之一,也可以用于设置代理。与 Requests 类似,urllib 可以通过环境变量或程序代码来配置代理。
1. 通过环境变量设置代理
你可以在操作系统的环境变量中设置 http_proxy
和 https_proxy
,然后 urllib 会自动使用这些代理。
export http_proxy="http://10.10.1.10:3128"
export https_proxy="http://10.10.1.10:1080"
2. 在代码中设置代理
也可以在代码中通过 urllib
的 ProxyHandler
来设置代理。
import urllib.request
proxy_support = urllib.request.ProxyHandler({
'http': 'http://10.10.1.10:3128',
'https': 'http://10.10.1.10:1080',
})
opener = urllib.request.build_opener(proxy_support)
urllib.request.install_opener(opener)
response = urllib.request.urlopen('http://example.com')
print(response.read().decode('utf-8'))
三、使用 SOCKS 代理
SOCKS 代理是一种不同于 HTTP 的代理协议,通常用于更高级的代理需求。Python 可以通过 socks
库或 PySocks
包来支持 SOCKS 代理。
1. 安装 PySocks
首先,需要安装 PySocks 包:
pip install pysocks
2. 配置 SOCKS 代理
可以通过设置 socks
参数来配置 SOCKS 代理。
import requests
import socks
import socket
socks.set_default_proxy(socks.SOCKS5, "10.10.1.10", 1080)
socket.socket = socks.socksocket
response = requests.get('http://example.com')
print(response.text)
四、使用 VPN 进行代理
VPN 是一种更全面的代理解决方案,它通过加密流量和改变 IP 地址来提供隐私保护。Python 本身不直接支持 VPN 配置,但可以通过配置系统网络设置或使用 VPN 客户端来实现。
1. 配置 VPN 客户端
大多数操作系统都有内置的 VPN 客户端,或者可以使用第三方软件(如 OpenVPN、NordVPN 等)。设置好 VPN 后,Python 代码将自动使用新的网络配置。
2. 结合 Requests 使用
一旦 VPN 连接建立,Python 的 HTTP 请求将通过新的 IP 地址发送,无需在代码中进行额外的配置。
import requests
假设已经通过系统或第三方软件连接到 VPN
response = requests.get('http://example.com')
print(response.text)
五、使用代理池进行轮询
在进行大量请求时,使用单个代理可能会导致被目标网站屏蔽。因此,可以通过代理池来轮询多个代理,提高成功率。
1. 创建代理池
可以通过维护一个代理列表来创建代理池,然后在每次请求时随机选择一个代理。
import random
proxies_pool = [
'http://10.10.1.10:3128',
'http://10.10.1.11:3128',
'http://10.10.1.12:3128',
]
proxy = random.choice(proxies_pool)
proxies = {'http': proxy, 'https': proxy}
response = requests.get('http://example.com', proxies=proxies)
print(response.text)
2. 动态更新代理池
代理池中的代理可能会失效,因此需要定期更新代理列表,确保有效性。可以通过编写脚本从代理提供商处获取最新的代理列表。
六、处理代理错误
在使用代理时,可能会遇到各种错误,如连接超时、身份验证失败等。需要对这些错误进行处理,以提高程序的健壮性。
1. 捕获异常
可以通过捕获异常来处理常见的网络错误。
try:
response = requests.get('http://example.com', proxies=proxies, timeout=5)
print(response.text)
except requests.exceptions.ProxyError as e:
print(f"Proxy Error: {e}")
except requests.exceptions.Timeout as e:
print(f"Timeout Error: {e}")
except requests.exceptions.RequestException as e:
print(f"Request Exception: {e}")
2. 重试机制
对于偶发的网络错误,可以通过重试机制来增加成功请求的概率。
import time
def fetch_with_retry(url, retries=3):
for attempt in range(retries):
try:
response = requests.get(url, proxies=proxies, timeout=5)
return response.text
except (requests.exceptions.ProxyError, requests.exceptions.Timeout):
if attempt < retries - 1:
time.sleep(2) # 等待一段时间再重试
else:
raise
try:
print(fetch_with_retry('http://example.com'))
except Exception as e:
print(f"Failed to fetch: {e}")
通过以上方法,可以在 Python 中高效地使用代理,满足各种网络请求的需求。
相关问答FAQs:
如何在Python中设置HTTP代理?
在Python中,您可以使用requests
库来设置HTTP代理。在创建请求时,您只需在请求参数中添加proxies
字典,指定所需的代理地址。例如:
import requests
proxies = {
"http": "http://your_proxy_address:port",
"https": "https://your_proxy_address:port",
}
response = requests.get('http://example.com', proxies=proxies)
print(response.text)
确保将your_proxy_address
和port
替换为实际的代理服务器信息。
使用代理时会影响网络速度吗?
使用代理可能会对网络速度产生影响。这取决于代理服务器的性能和您的网络连接。如果代理服务器响应迅速且带宽充足,您可能不会注意到显著的速度变化。然而,如果代理服务器负载过重或地理位置较远,您可能会体验到延迟或速度下降。
如何在Python中使用代理进行爬虫?
在进行网络爬虫时,使用代理可以帮助您避免被目标网站封禁。您可以在requests
库中设置代理,也可以使用Scrapy
框架。在Scrapy中,可以在settings.py
文件中配置代理,例如:
DOWNLOADER_MIDDLEWARES = {
'scrapy.downloadermiddlewares.httpproxy.HttpProxyMiddleware': 110,
'scrapy.downloadermiddlewares.proxy.ProxyMiddleware': 100,
}
HTTP_PROXY = 'http://your_proxy_address:port'
这样设置后,Scrapy将在请求中自动使用指定的代理。
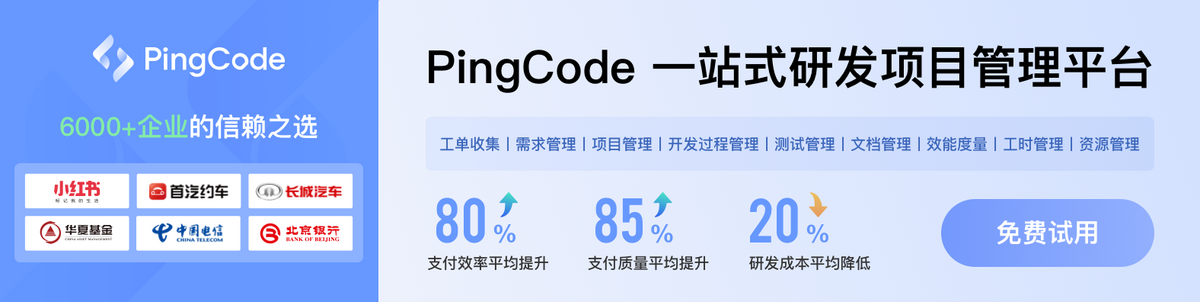