在Python中,你可以使用文件写入功能将print输出保存到文件中、使用日志模块记录打印信息、重定向标准输出流到文件。最简单的方法是使用Python内置的文件操作功能,具体步骤包括打开一个文件、使用write方法将信息写入文件、关闭文件。使用日志模块能提供更灵活和强大的信息记录功能,适合需要详细记录程序运行情况的场景。重定向标准输出流则能将所有print输出重定向到文件中,适用于需要在不修改大量print语句的情况下保存输出的场景。
一、文件写入
使用Python内置的文件操作功能保存print输出是最直接的方法。你可以通过打开一个文件,将信息写入文件,然后关闭文件来实现。
-
打开文件
首先,你需要使用
open()
函数打开一个文件。该函数的第一个参数是文件名,第二个参数是文件模式。常见的文件模式包括:'w'
:写入模式,如果文件已存在,则会被覆盖;'a'
:追加模式,信息会被添加到文件末尾;'r'
:读取模式。
file = open('output.txt', 'w')
-
写入信息
使用文件对象的
write()
方法将信息写入文件。注意,write()
方法接受字符串类型的数据,因此需要将非字符串数据转换为字符串。file.write('This is a test.\n')
-
关闭文件
完成写入后,务必使用
close()
方法关闭文件,以确保数据完整写入并释放系统资源。file.close()
-
示例代码
以下是一个完整的示例代码,将print输出保存到文件中:
output = 'Hello, World!'
file = open('output.txt', 'w')
file.write(output + '\n')
file.close()
二、使用日志模块
Python的logging
模块提供了一个简单的方式来记录程序的运行信息。与直接将print输出保存到文件相比,logging
模块提供了更多的功能,如设置日志级别、格式化日志信息等。
-
导入模块
首先,需要导入
logging
模块。import logging
-
配置日志
使用
basicConfig()
函数配置日志,设置日志文件名、日志级别、日志格式等。logging.basicConfig(filename='app.log', level=logging.INFO,
format='%(asctime)s - %(levelname)s - %(message)s')
-
记录信息
使用
logging
模块中的方法记录信息,如info()
、warning()
、error()
等。logging.info('This is an info message.')
-
示例代码
以下是一个完整的示例代码,将信息记录到日志文件中:
import logging
logging.basicConfig(filename='app.log', level=logging.INFO,
format='%(asctime)s - %(levelname)s - %(message)s')
logging.info('Application started.')
logging.info('This is an info message.')
logging.warning('This is a warning message.')
logging.error('This is an error message.')
三、重定向标准输出流
在某些情况下,你可能需要将所有的print输出重定向到文件中,而不必逐一修改每个print语句。Python提供了一种方法来重定向标准输出流,实现这一功能。
-
导入模块
需要导入
sys
模块。import sys
-
重定向标准输出
使用
sys.stdout
重定向标准输出流到文件中。original_stdout = sys.stdout # Save a reference to the original standard output
with open('output.txt', 'w') as f:
sys.stdout = f # Change the standard output to the file we created.
print('This will be written to the file.')
sys.stdout = original_stdout # Reset the standard output to its original value
-
示例代码
以下是一个完整的示例代码,将所有print输出重定向到文件中:
import sys
original_stdout = sys.stdout # Save a reference to the original standard output
with open('output.txt', 'w') as f:
sys.stdout = f # Change the standard output to the file we created.
print('This will be written to the file.')
print('So will this.')
sys.stdout = original_stdout # Reset the standard output to its original value
print('This will be printed on the console.')
四、结论
在Python中,有多种方法可以将print输出保存到文件中。选择哪种方法取决于你的具体需求:
- 如果只是偶尔需要保存一些输出,使用文件写入功能可能是最简单的方法。
- 如果需要记录程序的运行情况,使用
logging
模块可以提供更强大的功能。 - 如果需要在不修改大量print语句的情况下保存输出,重定向标准输出流是一个不错的选择。
在使用这些方法时,请注意文件的读写权限,以及在写入完成后关闭文件以释放系统资源。通过合理使用这些方法,你可以更有效地管理和记录程序的输出。
相关问答FAQs:
如何将Python中的print输出保存为文件?
在Python中,可以使用文件操作将print输出保存到文件。可以使用open()
函数创建或打开一个文件,并使用file.write()
方法将内容写入文件。例如:
with open('output.txt', 'w') as f:
print("Hello, World!", file=f)
这段代码会将“Hello, World!”的输出保存到output.txt
文件中。
在Python中是否可以将print输出保存为变量?
是的,可以使用io.StringIO
模块来捕获print输出并保存为变量。以下是一个示例:
import io
import sys
output = io.StringIO()
sys.stdout = output
print("Hello, World!")
sys.stdout = sys.__stdout__ # 恢复stdout
saved_output = output.getvalue()
output.close()
以上代码将print输出保存到saved_output
变量中。
如何格式化print输出并保存到文件中?
在保存print输出到文件时,可以使用格式化字符串来更好地控制输出内容。例如:
name = "Alice"
age = 30
with open('output.txt', 'w') as f:
print(f"My name is {name} and I am {age} years old.", file=f)
这种方法可以方便地将变量的值插入到字符串中,并将最终的内容保存到文件。
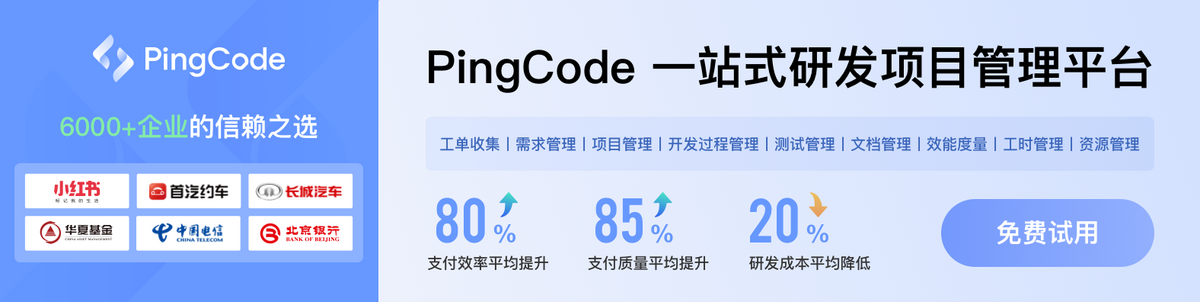