在Python中,取消特定操作或中止某个功能通常取决于具体上下文或应用场景。以下是一些常见情况下的解决方案:
1、取消循环中的操作
如果您在一个循环中并希望取消或跳过某次迭代,可以使用continue
语句。若要完全退出循环,可以使用break
语句。
for i in range(10):
if i == 5:
continue # 跳过本次迭代
if i == 8:
break # 退出循环
print(i)
在上面的例子中,当i
等于5时,continue
语句将跳过本次循环,不执行print(i)
;当i
等于8时,break
语句将终止整个循环。
2、取消函数的执行
在函数中,您可以通过return
语句来中止函数的执行。
def example_function(n):
if n < 0:
return # 取消函数的执行
print(f"Processing number: {n}")
example_function(-1) # 函数将不执行任何操作
example_function(5) # 将会打印“Processing number: 5”
3、取消线程
在多线程编程中,取消一个线程通常需要使用某种信号或标志,因为Python的线程没有直接的取消机制。可以通过设置一个标志变量来通知线程停止。
import threading
import time
def worker(stop_event):
while not stop_event.is_set():
print("Thread is running")
time.sleep(1)
stop_event = threading.Event()
thread = threading.Thread(target=worker, args=(stop_event,))
thread.start()
time.sleep(5)
stop_event.set() # 通知线程停止
thread.join()
4、取消异步任务
在异步编程中,使用asyncio
库可以取消异步任务。您可以通过调用Task
对象的cancel()
方法来取消任务。
import asyncio
async def example_task():
try:
await asyncio.sleep(10)
except asyncio.CancelledError:
print("Task was cancelled")
async def main():
task = asyncio.create_task(example_task())
await asyncio.sleep(1)
task.cancel() # 取消任务
await task
asyncio.run(main())
5、取消信号处理
在某些情况下,您可能希望使用信号来取消长时间运行的进程。例如,在Linux系统中,可以使用signal
模块来处理信号。
import signal
import time
def handler(signum, frame):
print("Signal handler called with signal", signum)
signal.signal(signal.SIGINT, handler)
while True:
print("Running...")
time.sleep(2)
在这个例子中,当用户按下Ctrl+C
时,程序将捕获SIGINT
信号,并调用handler
函数。
以上是一些常见的取消操作的场景和解决方案。在实际应用中,具体的实现可能会因为应用程序的需求不同而有所变化。
相关问答FAQs:
如何在Python中取消一个变量的赋值?
在Python中,可以通过将变量赋值为None
来“取消”其原有的值。例如,若你有一个变量n
,可以使用n = None
来清除其值。这样在后续代码中,n
将不再指向原来的数据。
取消变量n的引用会有什么影响?
当你取消变量n
的引用,比如将其赋值为None
或重新赋值为其他类型的数据,原本存储在n
中的数据将不再可以通过n
访问。如果没有其他变量引用该数据,Python的垃圾回收机制会自动释放该数据所占用的内存。
在Python中,如何检查一个变量是否被取消或未定义?
可以使用is None
来检查一个变量是否被设置为None
。例如,执行if n is None:
可以用来判断变量n
是否已经取消或未被定义。此外,使用try-except
结构捕获NameError
也可以判断变量是否存在,例如:
try:
print(n)
except NameError:
print("变量n未定义")
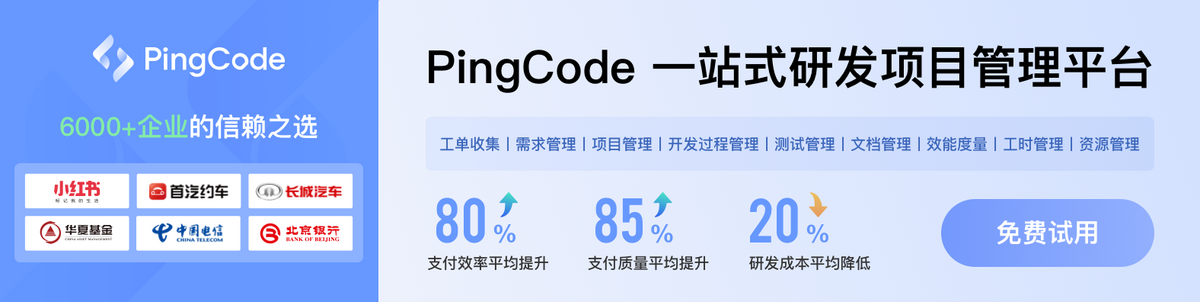