使用Python计算词频的方法主要有:使用collections.Counter、使用字典、使用pandas、使用nltk库。在实际应用中,collections.Counter是最为简洁和高效的方法,因为它专为计数设计,能快速统计词频。下面我将详细介绍如何使用collections.Counter来计算词频。
使用collections.Counter进行词频统计非常简单,只需将文本转换为词列表,然后使用Counter统计即可。首先,你需要将文本进行预处理,比如去除标点符号、将文本转为小写等。然后,利用split()
方法将文本按空格分割成词列表,最后使用Counter进行统计。例如:
from collections import Counter
import re
def calculate_word_frequency(text):
# 文本预处理
text = text.lower() # 转换为小写
text = re.sub(r'[^\w\s]', '', text) # 去除标点符号
words = text.split() # 分割成词列表
# 使用Counter计算词频
word_freq = Counter(words)
return word_freq
text = "Python is great. Python is dynamic. Python is easy to learn."
word_frequency = calculate_word_frequency(text)
print(word_frequency)
一、使用COLLECTIONS.COUNTER进行词频统计
collections模块中的Counter类是专门为计数设计的工具,它是一个字典的子类,专门用来计数可哈希对象。使用Counter进行词频统计的步骤如下:
-
文本预处理:文本预处理是词频统计的第一步。在这一步中,我们需要将文本转换为小写,并去除所有的标点符号。这是因为在大多数情况下,我们不希望将相同的单词由于大小写不同或因标点符号而被视为不同的词。
import re
def preprocess_text(text):
text = text.lower() # 转换为小写
text = re.sub(r'[^\w\s]', '', text) # 去除标点符号
return text
-
分割文本:文本预处理完成后,我们需要将文本分割成一个个单词。通常,我们可以使用Python的
split()
方法来完成这一步。split()
方法会将字符串按空格分割成一个列表,其中每个元素就是一个单词。def split_text(text):
return text.split()
-
使用Counter进行计数:将预处理后的文本分割成单词列表后,就可以使用Counter进行计数了。Counter会返回一个字典,其中键是词,值是词出现的次数。
from collections import Counter
def calculate_word_frequency(words):
return Counter(words)
-
完整代码实现:将以上步骤整合在一起,我们可以实现一个完整的词频统计函数。
from collections import Counter
import re
def calculate_word_frequency(text):
# 文本预处理
text = preprocess_text(text)
# 分割文本
words = split_text(text)
# 使用Counter计算词频
word_freq = calculate_word_frequency(words)
return word_freq
def preprocess_text(text):
text = text.lower()
text = re.sub(r'[^\w\s]', '', text)
return text
def split_text(text):
return text.split()
text = "Python is great. Python is dynamic. Python is easy to learn."
word_frequency = calculate_word_frequency(text)
print(word_frequency)
二、使用字典计算词频
虽然collections.Counter是计算词频的简便方法,但我们也可以使用普通的字典来实现这一功能。这种方法可以帮助我们更好地理解词频统计的基本原理。
-
初始化字典:首先,我们需要创建一个空字典来存储词频信息。
def calculate_word_frequency(text):
word_freq = {}
return word_freq
-
遍历词列表并更新字典:对于文本中的每个词,我们检查它是否已经在字典中。如果在,则将其对应的值加一;如果不在,则将其添加到字典中,并将值设置为1。
def calculate_word_frequency(text):
word_freq = {}
for word in words:
if word in word_freq:
word_freq[word] += 1
else:
word_freq[word] = 1
return word_freq
-
完整代码实现:将以上步骤整合到一个完整的函数中。
import re
def calculate_word_frequency(text):
word_freq = {}
text = preprocess_text(text)
words = split_text(text)
for word in words:
if word in word_freq:
word_freq[word] += 1
else:
word_freq[word] = 1
return word_freq
def preprocess_text(text):
text = text.lower()
text = re.sub(r'[^\w\s]', '', text)
return text
def split_text(text):
return text.split()
text = "Python is great. Python is dynamic. Python is easy to learn."
word_frequency = calculate_word_frequency(text)
print(word_frequency)
三、使用PANDAS计算词频
Pandas是一个功能强大的数据分析库,它提供了许多方便的数据操作功能。虽然Pandas不是专为词频统计设计的,但我们可以使用它的DataFrame来实现这一功能。
-
导入Pandas库并创建DataFrame:首先,我们需要导入Pandas库,并将词列表转换为一个DataFrame。
import pandas as pd
def calculate_word_frequency(words):
df = pd.DataFrame(words, columns=['word'])
return df
-
使用value_counts()计算词频:DataFrame的
value_counts()
方法可以直接用于计算词频。def calculate_word_frequency(words):
df = pd.DataFrame(words, columns=['word'])
word_freq = df['word'].value_counts()
return word_freq
-
完整代码实现:将以上步骤整合到一个完整的函数中。
import pandas as pd
import re
def calculate_word_frequency(text):
text = preprocess_text(text)
words = split_text(text)
df = pd.DataFrame(words, columns=['word'])
word_freq = df['word'].value_counts()
return word_freq
def preprocess_text(text):
text = text.lower()
text = re.sub(r'[^\w\s]', '', text)
return text
def split_text(text):
return text.split()
text = "Python is great. Python is dynamic. Python is easy to learn."
word_frequency = calculate_word_frequency(text)
print(word_frequency)
四、使用NLTK库计算词频
NLTK(Natural Language Toolkit)是一个用于自然语言处理的Python库,它提供了许多有用的功能来处理和分析文本数据。我们可以使用NLTK库中的FreqDist
类来计算词频。
-
安装和导入NLTK库:首先,你需要安装NLTK库,并导入它。
pip install nltk
import nltk
-
使用FreqDist计算词频:NLTK库中的
FreqDist
类可以用于计算词频。from nltk.probability import FreqDist
def calculate_word_frequency(words):
freq_dist = FreqDist(words)
return freq_dist
-
完整代码实现:将以上步骤整合到一个完整的函数中。
import nltk
from nltk.probability import FreqDist
import re
def calculate_word_frequency(text):
text = preprocess_text(text)
words = split_text(text)
freq_dist = FreqDist(words)
return freq_dist
def preprocess_text(text):
text = text.lower()
text = re.sub(r'[^\w\s]', '', text)
return text
def split_text(text):
return text.split()
text = "Python is great. Python is dynamic. Python is easy to learn."
word_frequency = calculate_word_frequency(text)
print(word_frequency)
在这篇文章中,我们详细介绍了如何使用Python进行词频统计。我们探讨了使用collections.Counter、字典、pandas和nltk库的方法。每种方法都有其优缺点,选择哪种方法取决于你的具体需求和使用场景。collections.Counter是最为简洁和高效的方法,适合大多数常见的词频统计任务。如果需要进行更复杂的文本分析,nltk库将是一个非常有用的工具。希望这篇文章能帮助你更好地理解和应用词频统计。
相关问答FAQs:
如何使用Python计算文本中的词频?
要计算文本中的词频,可以使用Python的内置功能和一些流行的库,如collections
模块中的Counter
类。首先,读取文本数据,接着将文本分割成单词,最后使用Counter
来计算每个单词出现的次数。以下是一个简单的示例代码:
from collections import Counter
text = "这是一个示例文本。示例文本用于计算词频。"
words = text.split() # 将文本分割为单词
word_count = Counter(words) # 计算词频
print(word_count) # 输出词频统计结果
是否可以在Python中排除常见词(停用词)进行词频计算?
绝对可以。停用词通常是指在文本处理中被忽略的高频词,如“的”、“是”、“在”等。在计算词频时,可以先定义一个停用词列表,然后在分词时过滤掉这些词。例如:
stop_words = set(["的", "是", "在"]) # 定义停用词
filtered_words = [word for word in words if word not in stop_words] # 过滤停用词
word_count = Counter(filtered_words) # 计算词频
如何处理大文件中的词频计算?
对于大型文本文件,建议逐行读取文件而不是一次性加载到内存中。这可以避免内存溢出的问题。可以使用open()
函数逐行读取文件,并在处理每一行时更新词频统计。以下是示例代码:
from collections import Counter
word_count = Counter()
with open('large_text_file.txt', 'r', encoding='utf-8') as file:
for line in file:
words = line.split()
word_count.update(words) # 更新词频统计
print(word_count) # 输出结果
这些方法和示例将帮助您利用Python有效地计算文本中的词频。
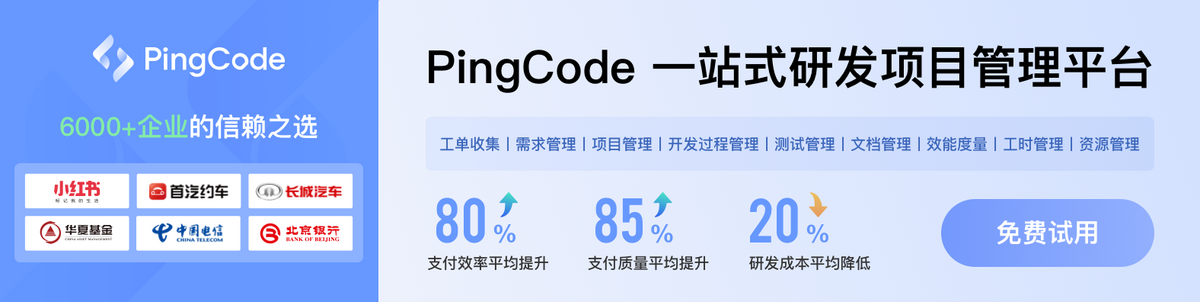