使用Python抓图的方法包括使用Pillow库进行图像处理、利用Selenium抓取网页截图、使用Matplotlib生成图表、运用OpenCV进行图像捕获和处理、以及通过PyAutoGUI进行屏幕截图。在这些方法中,Selenium抓取网页截图是一种非常常用的方法,因为它可以自动化浏览器操作并捕获网页的当前状态。下面将详细介绍如何使用Selenium进行网页截图。
一、使用Pillow进行图像处理
Pillow是Python Imaging Library(PIL)的一个分支,提供了强大的图像处理功能。通过Pillow,我们可以打开、操作和保存不同格式的图像。
1. 安装Pillow
首先,确保安装了Pillow库:
pip install pillow
2. 使用Pillow加载和保存图像
通过以下代码可以加载和保存图像:
from PIL import Image
打开图像
image = Image.open('example.jpg')
显示图像
image.show()
保存图像
image.save('example_copy.png')
3. 使用Pillow进行图像处理
Pillow还支持多种图像处理操作,例如裁剪、调整大小、旋转等:
# 裁剪图像
cropped_image = image.crop((100, 100, 400, 400))
cropped_image.show()
调整大小
resized_image = image.resize((200, 200))
resized_image.show()
旋转图像
rotated_image = image.rotate(45)
rotated_image.show()
二、利用Selenium抓取网页截图
Selenium是一个强大的工具,可以自动化Web浏览器操作。我们可以利用Selenium抓取网页的截图。
1. 安装Selenium和浏览器驱动
首先,安装Selenium库,并确保有相应的浏览器驱动(如ChromeDriver):
pip install selenium
下载ChromeDriver并将其路径添加到系统环境变量中。
2. 使用Selenium抓取网页截图
以下是使用Selenium抓取网页截图的示例代码:
from selenium import webdriver
设置浏览器驱动路径
driver_path = '/path/to/chromedriver'
创建浏览器实例
driver = webdriver.Chrome(executable_path=driver_path)
打开网页
driver.get('https://www.example.com')
设置窗口大小
driver.set_window_size(1920, 1080)
截取网页截图
driver.save_screenshot('webpage_screenshot.png')
关闭浏览器
driver.quit()
3. 处理动态内容
对于动态内容,我们可能需要等待内容加载完成后再截取截图。可以使用WebDriverWait实现:
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
等待元素加载
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.ID, 'some_element_id'))
)
截取截图
driver.save_screenshot('dynamic_content_screenshot.png')
三、使用Matplotlib生成图表
Matplotlib是一个用于生成图表的强大库。我们可以使用它生成并保存图表作为图像。
1. 安装Matplotlib
pip install matplotlib
2. 使用Matplotlib创建和保存图表
以下是一个简单的示例,展示如何使用Matplotlib创建并保存图表:
import matplotlib.pyplot as plt
创建数据
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
绘制图表
plt.plot(x, y)
添加标题和标签
plt.title('Simple Plot')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
保存图表
plt.savefig('plot.png')
显示图表
plt.show()
四、使用OpenCV进行图像捕获和处理
OpenCV是一个开源计算机视觉库,支持实时图像和视频处理。
1. 安装OpenCV
pip install opencv-python
2. 使用OpenCV捕获和处理图像
以下是使用OpenCV捕获和处理图像的示例:
import cv2
捕获视频流
cap = cv2.VideoCapture(0)
捕获图像帧
ret, frame = cap.read()
显示图像
cv2.imshow('Captured Image', frame)
保存图像
cv2.imwrite('captured_image.png', frame)
释放视频捕获对象
cap.release()
关闭所有OpenCV窗口
cv2.destroyAllWindows()
五、使用PyAutoGUI进行屏幕截图
PyAutoGUI是一个用于GUI自动化的库,可以实现屏幕截图功能。
1. 安装PyAutoGUI
pip install pyautogui
2. 使用PyAutoGUI截取屏幕
以下是使用PyAutoGUI截取屏幕的简单示例:
import pyautogui
截取屏幕截图
screenshot = pyautogui.screenshot()
保存截图
screenshot.save('screenshot.png')
综上所述,Python提供了多种方法来实现图像抓取和处理。选择哪种方法取决于具体的应用场景和需求。无论是处理本地图像文件、抓取网页截图、生成图表,还是进行实时图像处理,Python都提供了丰富的工具和库来满足不同的需求。
相关问答FAQs:
如何使用Python抓取网页上的图片?
抓取网页图片的常用方法是通过使用requests库获取网页内容,然后利用BeautifulSoup库解析HTML,找到图片的URL。接着,可以使用requests库下载这些图片。以下是一个简单的示例代码:
import requests
from bs4 import BeautifulSoup
url = '目标网页URL'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
for img in soup.find_all('img'):
img_url = img.get('src')
img_data = requests.get(img_url).content
with open('图片名称.jpg', 'wb') as handler:
handler.write(img_data)
确保在运行代码前安装了所需的库。
抓取图片时需要注意哪些法律和道德问题?
在抓取网页图片时,应遵循版权法和网站的使用条款。很多网站的图片受版权保护,未经授权的使用可能导致法律问题。在抓取之前,最好查看网站的robots.txt文件和相关的使用政策,确保遵循其抓取规则。
是否可以使用Python抓取动态加载的图片?
可以。对于动态加载的图片,通常需要使用Selenium等工具模拟浏览器行为。Selenium可以执行JavaScript代码并等待网页完全加载,从而抓取动态内容。示例代码如下:
from selenium import webdriver
driver = webdriver.Chrome()
driver.get('目标网页URL')
images = driver.find_elements_by_tag_name('img')
for img in images:
img_url = img.get_attribute('src')
img_data = requests.get(img_url).content
with open('图片名称.jpg', 'wb') as handler:
handler.write(img_data)
driver.quit()
确保安装了Selenium和相应的浏览器驱动。
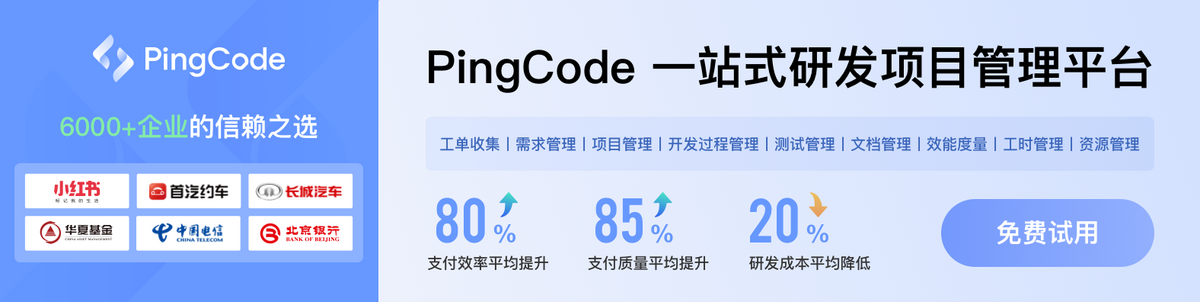