要在Python的Tkinter库中删除组件或元素,可以使用destroy()
方法、pack_forget()
、grid_forget()
方法。这些方法可以删除或隐藏Tkinter窗口中的控件。destroy()
方法用于永久删除控件,而pack_forget()
和grid_forget()
方法用于临时隐藏控件。下面我将详细介绍这三种方法的使用。
在Tkinter中,控件是通过布局管理器(如pack
、grid
或place
)来放置的。根据布局方式的不同,删除或隐藏控件的方法也有所不同。以下是详细的说明和使用示例:
一、使用 destroy()
方法删除控件
destroy()
方法用于彻底删除控件。当您调用该方法时,控件及其所有子控件都会被永久移除,无法恢复。如果需要删除一个控件,这是最常用的方法。
示例:
import tkinter as tk
def delete_label():
label.destroy()
root = tk.Tk()
label = tk.Label(root, text="This is a label")
label.pack()
button = tk.Button(root, text="Delete the label", command=delete_label)
button.pack()
root.mainloop()
在这个示例中,当点击按钮时,delete_label
函数会被调用,label
控件会被删除。
二、使用 pack_forget()
和 grid_forget()
方法隐藏控件
1. pack_forget()
方法
pack_forget()
方法用于隐藏通过pack
管理器放置的控件。控件会从窗口中消失,但并没有被销毁,您可以通过再次调用pack()
将其恢复。
示例:
import tkinter as tk
def hide_label():
label.pack_forget()
def show_label():
label.pack()
root = tk.Tk()
label = tk.Label(root, text="This is a label")
label.pack()
hide_button = tk.Button(root, text="Hide the label", command=hide_label)
hide_button.pack()
show_button = tk.Button(root, text="Show the label", command=show_label)
show_button.pack()
root.mainloop()
通过点击“Hide the label”按钮,标签会被隐藏;点击“Show the label”按钮,标签会重新出现。
2. grid_forget()
方法
grid_forget()
方法用于隐藏通过grid
管理器放置的控件。与pack_forget()
类似,控件被隐藏但没有被销毁。
示例:
import tkinter as tk
def hide_label():
label.grid_forget()
def show_label():
label.grid(row=0, column=0)
root = tk.Tk()
label = tk.Label(root, text="This is a label")
label.grid(row=0, column=0)
hide_button = tk.Button(root, text="Hide the label", command=hide_label)
hide_button.grid(row=1, column=0)
show_button = tk.Button(root, text="Show the label", command=show_label)
show_button.grid(row=2, column=0)
root.mainloop()
在这个示例中,通过点击“Hide the label”按钮可以隐藏标签,而“Show the label”按钮可以使其重新出现。
三、选择合适的方法
在选择删除或隐藏控件的方法时,应该根据具体的需求来决定。如果您需要永久删除控件,destroy()
方法是最佳选择;如果只需要临时隐藏控件并可能在以后恢复,pack_forget()
或grid_forget()
方法则更为合适。
1. 使用场景分析
destroy()
: 适用于不再需要的控件,释放资源。pack_forget()
/grid_forget()
: 适用于需要暂时隐藏控件的场景,如切换视图或动态更新界面。
2. 注意事项
- 使用
destroy()
后,控件将不再存在,无法通过简单的方法恢复。 - 使用
pack_forget()
或grid_forget()
后,控件仍然存在于内存中,可以通过适当的方法恢复显示。
四、综合示例
下面是一个综合示例,展示了如何在一个应用程序中使用这三种方法。
import tkinter as tk
def destroy_label():
label.destroy()
def hide_label():
label.pack_forget()
def show_label():
label.pack()
root = tk.Tk()
label = tk.Label(root, text="This is a label")
label.pack()
destroy_button = tk.Button(root, text="Destroy the label", command=destroy_label)
destroy_button.pack()
hide_button = tk.Button(root, text="Hide the label", command=hide_label)
hide_button.pack()
show_button = tk.Button(root, text="Show the label", command=show_label)
show_button.pack()
root.mainloop()
通过这个示例,您可以体验到不同的控件管理方法带来的效果差异。选择适合您需求的方法,以实现最佳的用户体验和界面管理。
通过合理使用destroy()
、pack_forget()
和grid_forget()
方法,您可以更好地管理Tkinter应用程序的界面布局,提高程序的灵活性和用户体验。希望本文对您在处理Tkinter控件时有所帮助。
相关问答FAQs:
如何在Python Tkinter中删除一个组件?
在Tkinter中,删除一个组件可以使用destroy()
方法。调用这个方法会将指定的组件从界面中移除。例如,如果你有一个按钮想要删除,可以使用button.destroy()
。确保在删除组件时,组件的引用仍然可用,以便调用这个方法。
有没有办法在Tkinter中隐藏组件而不是删除?
是的,Tkinter提供了pack_forget()
、grid_forget()
和place_forget()
等方法,可以将组件隐藏而不删除。这些方法会将组件从显示中移除,但保留其状态和属性,方便之后重新显示。使用这些方法时,可以根据组件的布局管理器选择相应的方法。
如何在Tkinter中删除多个组件?
若要同时删除多个组件,可以将它们的引用存储在一个列表中,然后遍历该列表,逐个调用destroy()
方法。这样可以有效地管理和删除多个组件,保持代码整洁。例如:
widgets = [button1, button2, label1]
for widget in widgets:
widget.destroy()
这样可以确保所有指定的组件都被删除。
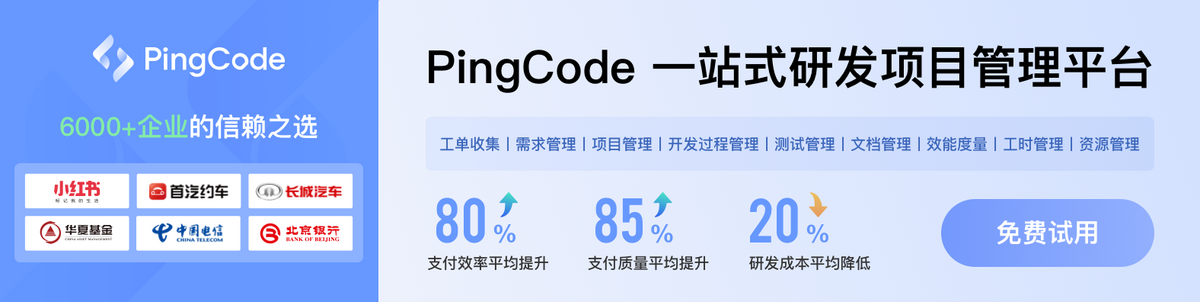