在Python中,使用def
关键字来定义函数。通过def
关键字,你可以创建一个可重用的代码块,接收参数并返回结果、定义一个函数的基本步骤包括使用def
关键词、命名函数、指定参数列表和编写函数体。函数的使用可以提高代码的复用性和可读性。以下是详细的解释和示例:
函数定义和调用
在Python中,函数是通过def
关键字来定义的。定义一个函数的基本格式如下:
def function_name(parameters):
"""docstring"""
# Function body
return expression
- function_name 是函数的名称,用于标识函数。
- parameters 是一个可选的列表,用于定义函数接受的参数。
- docstring 是一个可选的字符串,用于描述函数的功能。
- Function body 是函数执行的代码块。
- return 是一个可选的语句,用于返回函数的结果。
一、函数的基本定义和调用
函数的定义和调用是Python编程的基础,通过定义函数,可以将常用的操作封装成一个独立的代码块,从而提高代码的复用性和可维护性。
1. 定义和调用无参数函数
一个简单的函数定义示例如下:
def greet():
"""This function greets the user."""
print("Hello, welcome to Python programming!")
在这个例子中,函数greet
没有参数,调用时只需使用函数名即可:
greet()
输出:
Hello, welcome to Python programming!
2. 定义和调用有参数函数
如果需要传递参数到函数中,可以在定义函数时指定参数列表:
def greet_user(name):
"""This function greets the user with their name."""
print(f"Hello, {name}, welcome to Python programming!")
调用时需要传递参数:
greet_user("Alice")
输出:
Hello, Alice, welcome to Python programming!
二、函数参数详解
Python函数参数提供了许多灵活的功能,包括默认参数、可变参数和关键字参数,使得函数调用更加灵活和可扩展。
1. 默认参数
在函数定义中,可以为参数指定默认值,这样在调用函数时可以不传递该参数:
def greet_user(name="Guest"):
"""This function greets the user with their name."""
print(f"Hello, {name}, welcome to Python programming!")
调用时可以省略参数:
greet_user()
输出:
Hello, Guest, welcome to Python programming!
2. 关键字参数
使用关键字参数调用函数,可以在调用时显式地指定参数的名称:
def greet_user(first_name, last_name):
"""This function greets the user with their full name."""
print(f"Hello, {first_name} {last_name}, welcome to Python programming!")
greet_user(last_name="Smith", first_name="John")
输出:
Hello, John Smith, welcome to Python programming!
3. 可变参数
Python允许定义可变数量的参数,使用*args
和kwargs
:
*args
:用于接收不定数量的位置参数。kwargs
:用于接收不定数量的关键字参数。
def print_fruits(*args):
"""This function prints all the fruits passed as arguments."""
for fruit in args:
print(fruit)
print_fruits("Apple", "Banana", "Cherry")
输出:
Apple
Banana
Cherry
def print_user_info(kwargs):
"""This function prints user information."""
for key, value in kwargs.items():
print(f"{key}: {value}")
print_user_info(name="Alice", age=30, city="New York")
输出:
name: Alice
age: 30
city: New York
三、函数的返回值
函数可以通过return
语句返回一个或多个值。返回值可以是任何类型的数据,包括数字、字符串、列表、元组、字典等。
1. 返回单个值
def add(a, b):
"""This function returns the sum of two numbers."""
return a + b
result = add(5, 3)
print(result)
输出:
8
2. 返回多个值
函数可以返回多个值,这些值会被打包成一个元组:
def get_user_info():
"""This function returns user information."""
name = "Alice"
age = 30
return name, age
user_name, user_age = get_user_info()
print(f"Name: {user_name}, Age: {user_age}")
输出:
Name: Alice, Age: 30
四、函数的作用域
在Python中,变量的作用域决定了变量的可见性和生命周期。函数内部定义的变量称为局部变量,函数外部定义的变量称为全局变量。
1. 局部变量
局部变量是在函数内部定义的变量,仅在函数内部可见:
def greet():
"""This function demonstrates local variables."""
message = "Hello, world!"
print(message)
greet()
输出:
Hello, world!
尝试在函数外部访问局部变量会导致错误:
print(message) # NameError: name 'message' is not defined
2. 全局变量
全局变量是在函数外部定义的变量,可以在函数内部访问和修改:
counter = 0
def increment():
"""This function increments the global counter."""
global counter
counter += 1
increment()
print(counter)
输出:
1
五、递归函数
递归函数是一个在其自身内部调用自身的函数。递归通常用于解决分治问题,如计算阶乘、斐波那契数列等。
1. 计算阶乘
def factorial(n):
"""This function calculates the factorial of a number."""
if n == 0:
return 1
else:
return n * factorial(n - 1)
print(factorial(5))
输出:
120
2. 斐波那契数列
def fibonacci(n):
"""This function returns the n-th Fibonacci number."""
if n <= 1:
return n
else:
return fibonacci(n - 1) + fibonacci(n - 2)
print(fibonacci(10))
输出:
55
六、匿名函数和高阶函数
Python支持匿名函数(lambda函数)和高阶函数(如map
、filter
、reduce
),这些函数提供了一种简洁的方法来处理函数式编程。
1. 匿名函数
匿名函数是一种没有名称的函数,使用lambda
关键字定义:
square = lambda x: x 2
print(square(4))
输出:
16
2. 高阶函数
高阶函数是可以接受函数作为参数或返回函数的函数:
def apply_function(func, value):
"""This function applies a given function to a value."""
return func(value)
result = apply_function(lambda x: x 2, 5)
print(result)
输出:
25
3. 使用map
、filter
、reduce
map
、filter
、reduce
是Python中常用的高阶函数:
from functools import reduce
map
numbers = [1, 2, 3, 4]
squared_numbers = list(map(lambda x: x 2, numbers))
print(squared_numbers)
filter
even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
print(even_numbers)
reduce
sum_of_numbers = reduce(lambda x, y: x + y, numbers)
print(sum_of_numbers)
输出:
[1, 4, 9, 16]
[2, 4]
10
通过以上内容,我们详细了解了Python中使用def
关键字定义和使用函数的各种方式。函数是Python编程中不可或缺的一部分,掌握函数的使用可以显著提高代码的质量和效率。
相关问答FAQs:
Python中的def关键字有什么作用?
def是Python中用于定义函数的关键字。使用def可以创建一个可重用的代码块,您可以通过函数名和参数调用它。函数可以接收输入参数并返回输出结果,这使得代码更加模块化,易于维护和理解。
在Python中如何定义一个简单的函数?
定义一个简单的函数可以使用以下格式:
def function_name(parameters):
# 执行的代码
return result
例如:
def add(a, b):
return a + b
这个函数接受两个参数a和b,返回它们的和。调用时只需使用add(2, 3)
,返回结果将是5。
如何在Python中使用def定义带有默认参数的函数?
在定义函数时,可以为参数设置默认值,这样即使调用时未提供某些参数,函数也能正常工作。格式如下:
def greet(name, message="Hello"):
return f"{message}, {name}!"
在这个例子中,如果只传递name参数,message将使用默认值“Hello”。例如,greet("Alice")
将返回“Hello, Alice!”而greet("Bob", "Hi")
将返回“Hi, Bob!”。
如何在Python中使用def定义带有可变参数的函数?
可以使用*args
和<strong>kwargs
来定义可接受任意数量参数的函数。*args
用于接收多个位置参数,而</strong>kwargs
用于接收多个关键字参数。示例代码如下:
def my_function(*args, **kwargs):
print("Positional arguments:", args)
print("Keyword arguments:", kwargs)
调用my_function(1, 2, 3, name="Alice", age=30)
将输出位置参数(1, 2, 3)
和关键字参数{'name': 'Alice', 'age': 30}
。
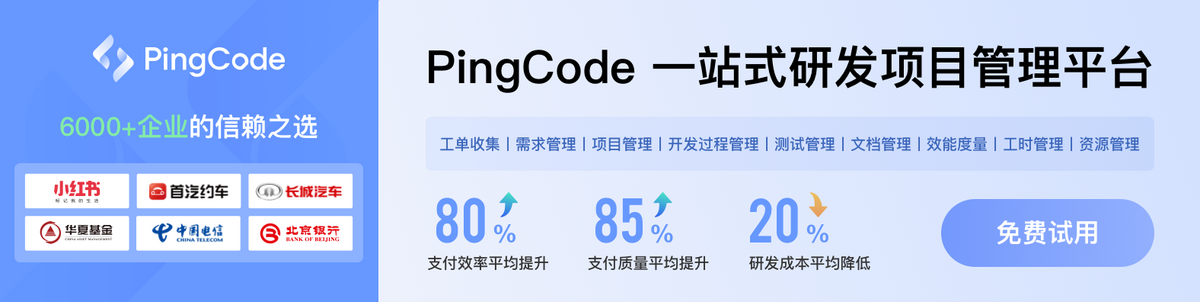