使用Python查看WiFi信息可以通过多种方法实现,如使用os
模块调用系统命令、使用subprocess
模块执行命令行指令、使用第三方库如pywifi
等。推荐使用pywifi
库,因为它提供了更高层次的接口来处理WiFi操作、支持跨平台使用。接下来,我将详细介绍如何使用这些方法中的一种来查看WiFi信息。
一、使用PYWIFI库查看WIFI信息
pywifi
是一个专门用于处理WiFi操作的Python库,支持扫描WiFi、连接WiFi、断开连接等操作。首先需要安装这个库,可以通过pip进行安装:
pip install pywifi
- 扫描WiFi
扫描WiFi是查看周围可用WiFi网络的第一步。可以使用pywifi
库中的scan
功能来获取周围的WiFi信息。以下是一个基本的例子:
from pywifi import PyWiFi, const
def scan_wifi():
wifi = PyWiFi()
iface = wifi.interfaces()[0]
iface.scan()
results = iface.scan_results()
for network in results:
print(f"SSID: {network.ssid}, Signal: {network.signal}, BSSID: {network.bssid}")
scan_wifi()
在这个例子中,我们首先创建一个PyWiFi
对象,然后获取第一个网络接口。调用scan
方法开始扫描,然后使用scan_results
方法获取扫描结果。每个结果包含SSID、信号强度和BSSID等信息。
- 连接WiFi
连接到指定WiFi网络需要知道该网络的SSID和密码。以下是如何使用pywifi
库连接到WiFi的示例:
from pywifi import PyWiFi, const, Profile
def connect_wifi(ssid, password):
wifi = PyWiFi()
iface = wifi.interfaces()[0]
profile = Profile()
profile.ssid = ssid
profile.auth = const.AUTH_ALG_OPEN
profile.akm.append(const.AKM_TYPE_WPA2PSK)
profile.cipher = const.CIPHER_TYPE_CCMP
profile.key = password
iface.remove_all_network_profiles()
tmp_profile = iface.add_network_profile(profile)
iface.connect(tmp_profile)
# 等待连接
import time
time.sleep(5)
if iface.status() == const.IFACE_CONNECTED:
print("Connected successfully.")
else:
print("Failed to connect.")
connect_wifi("YourSSID", "YourPassword")
在这个示例中,我们创建了一个Profile
对象,设置了SSID、认证方式、加密类型和密码等,然后将这个配置文件添加到网络接口,最后尝试连接。
二、使用SUBPROCESS模块查看WIFI信息
subprocess
模块可以用来执行系统命令,并获取执行结果。对于Windows用户,可以使用netsh
命令来获取WiFi信息。
- 获取WiFi配置
可以使用subprocess
模块执行netsh wlan show profiles
命令来获取所有已保存的WiFi配置。
import subprocess
def get_wifi_profiles():
command = "netsh wlan show profiles"
networks = subprocess.check_output(command, shell=True).decode('utf-8', errors='ignore')
profiles = [line.split(":")[1][1:-1] for line in networks.split("\n") if "All User Profile" in line]
return profiles
print(get_wifi_profiles())
- 查看WiFi密码
要查看某个WiFi的密码,可以使用netsh wlan show profile name="SSID" key=clear
命令,其中SSID
为WiFi的名称。
def get_wifi_password(ssid):
command = f'netsh wlan show profile name="{ssid}" key=clear'
result = subprocess.check_output(command, shell=True).decode('utf-8', errors='ignore')
for line in result.split("\n"):
if "Key Content" in line:
return line.split(":")[1][1:-1]
return None
ssid = "YourSSID"
password = get_wifi_password(ssid)
print(f"SSID: {ssid}, Password: {password}")
三、使用OS模块查看WIFI信息
os
模块可以用来执行简单的系统命令,但通常不如subprocess
模块灵活和强大。不过在某些简单情况下,os
模块也可以用来查看WiFi信息。
- 执行命令
使用os.system()
来执行WiFi相关的系统命令:
import os
os.system("netsh wlan show networks")
这种方法虽然简单,但无法捕获命令的输出,通常用于不需要处理输出的情况。
四、使用平台特定的工具
- Windows
在Windows系统上,可以使用netsh
命令获取WiFi信息,通过Python调用这些命令可以实现WiFi信息的查看和管理。
- Linux
在Linux系统上,可以使用iwconfig
和nmcli
等命令获取WiFi信息,Python可以通过subprocess
模块调用这些命令。
import subprocess
def linux_wifi_scan():
command = "nmcli dev wifi list"
networks = subprocess.check_output(command, shell=True).decode('utf-8', errors='ignore')
print(networks)
linux_wifi_scan()
- MacOS
在MacOS系统上,可以使用airport
命令来获取WiFi信息。
import subprocess
def macos_wifi_scan():
command = "/System/Library/PrivateFrameworks/Apple80211.framework/Versions/Current/Resources/airport -s"
networks = subprocess.check_output(command, shell=True).decode('utf-8', errors='ignore')
print(networks)
macos_wifi_scan()
总结而言,Python提供了多种方式来查看WiFi信息,具体选择哪种方法取决于用户的操作系统和具体需求。对于跨平台的解决方案,pywifi
库是一个不错的选择,而对于特定平台的实现,使用subprocess
模块调用系统命令是常见的做法。通过这些方法,用户可以方便地获取WiFi的相关信息,并进行进一步的操作。
相关问答FAQs:
如何使用Python查看当前连接的WiFi网络名称?
可以使用subprocess
模块结合系统命令来获取当前连接的WiFi名称。在Windows上,可以通过运行netsh wlan show interfaces
命令来获取信息;而在Linux上,可以使用iwgetid -r
命令。以下是一个简单的示例代码:
import subprocess
def get_current_wifi():
try:
# Windows
result = subprocess.check_output(["netsh", "wlan", "show", "interfaces"])
for line in result.decode().split('\n'):
if "SSID" in line:
return line.split(':')[1].strip()
except Exception as e:
print(f"Error: {e}")
return None
print(get_current_wifi())
Python可以获取WiFi信号强度吗?
是的,Python可以通过调用系统命令来获取WiFi信号强度。在Windows上,同样可以使用netsh wlan show interfaces
命令,而在Linux系统中,可以使用iwconfig
命令。解析命令输出中的信号强度信息,能够帮助用户了解网络的稳定性和连接质量。示例代码:
import subprocess
def get_wifi_signal_strength():
try:
result = subprocess.check_output(["netsh", "wlan", "show", "interfaces"])
for line in result.decode().split('\n'):
if "Signal" in line:
return line.split(':')[1].strip()
except Exception as e:
print(f"Error: {e}")
return None
print(get_wifi_signal_strength())
如何使用Python扫描附近的WiFi网络?
可以使用Python的subprocess
模块来执行系统命令,获取周围可用的WiFi网络列表。在Windows上,使用netsh wlan show networks
命令;在Linux上,可以通过nmcli dev wifi
命令。解析输出结果后,可以显示所有可用网络的名称和信号强度。以下是一个示例:
import subprocess
def scan_wifi_networks():
networks = []
try:
result = subprocess.check_output(["netsh", "wlan", "show", "network"])
for line in result.decode().split('\n'):
if "SSID" in line and "BSSID" not in line:
networks.append(line.split(':')[1].strip())
except Exception as e:
print(f"Error: {e}")
return networks
print(scan_wifi_networks())
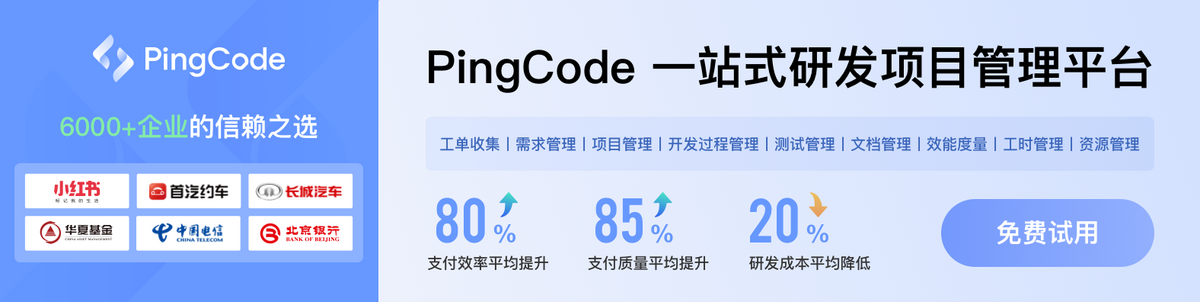