在Python中编写帮助文档可以通过以下几种方式:使用docstring、利用help()函数、生成外部文档。docstring是最常用的方法,可以为模块、类、函数和方法提供说明,直接在代码中编写。我们可以通过help()函数来查看这些文档。对于更复杂的项目,可以使用工具如Sphinx来生成外部文档。docstring不仅有助于代码的可读性,还能通过工具自动生成详细文档。
一、DOCSTRING编写
在Python中,docstring是一种用三重引号括起来的字符串,通常被放置在模块、类、方法或函数的开头。它用来描述代码的用途和功能。docstring的使用不仅有助于代码的可读性,还可以通过工具自动生成详细的文档。
-
函数docstring
编写docstring时,建议在函数定义下方使用三重引号,并简要描述函数的功能、参数和返回值。
def add(a, b):
"""
Add two numbers.
Parameters:
a (int): The first number.
b (int): The second number.
Returns:
int: The sum of a and b.
"""
return a + b
-
类和模块docstring
类和模块的docstring应放置在类定义或模块文件的顶部,描述其用途和功能。
class Calculator:
"""
A simple calculator class to perform basic operations like add, subtract, etc.
"""
def subtract(self, a, b):
"""
Subtracts one number from another.
Parameters:
a (int): The number to be subtracted from.
b (int): The number to subtract.
Returns:
int: The result of the subtraction.
"""
return a - b
二、利用HELP()函数
Python内置的help()函数可以用来查看docstring。只需将模块、类或函数作为参数传递给help()函数,即可查看其详细的docstring。
help(add)
help(Calculator)
三、生成外部文档
对于大型项目,使用工具生成外部文档是很有必要的。Sphinx是一个流行的Python文档生成工具,它可以从docstring中提取信息并生成HTML、PDF等格式的文档。
-
安装Sphinx
使用pip安装Sphinx:
pip install sphinx
-
初始化Sphinx项目
在项目目录中运行以下命令,初始化一个Sphinx项目:
sphinx-quickstart
按照提示进行配置,生成conf.py和index.rst文件。
-
编写rst文件
使用reStructuredText格式编写文档,将docstring内容添加到相应的rst文件中。
-
生成文档
运行以下命令生成HTML文档:
make html
生成的文档将位于_build/html目录中,可以在浏览器中查看。
四、DOCSTRING格式规范
为了确保docstring的清晰和一致,通常遵循一定的格式规范。最常用的格式规范包括Google风格、NumPy风格和reStructuredText风格。
-
Google风格
Google风格的docstring格式简洁明了,适合较短的文档。
def multiply(a, b):
"""Multiply two numbers.
Args:
a (int): The first number.
b (int): The second number.
Returns:
int: The product of a and b.
"""
return a * b
-
NumPy风格
NumPy风格的docstring格式较为详细,适合长文档。
def divide(a, b):
"""
Divide two numbers.
Parameters
----------
a : int
The numerator.
b : int
The denominator.
Returns
-------
float
The quotient of a and b.
"""
return a / b
-
reStructuredText风格
reStructuredText风格的docstring格式与Sphinx兼容,适合自动生成文档。
def power(base, exp):
"""
Raises a number to a power.
:param base: The base number.
:type base: int
:param exp: The exponent.
:type exp: int
:return: The result of base raised to the power of exp.
:rtype: int
"""
return base exp
五、使用注释提高代码可读性
除了docstring,良好的注释习惯也能提高代码的可读性和维护性。注释应当简洁明了,放置在必要的地方,解释复杂的逻辑或算法。
-
代码块注释
在复杂的代码块上方添加注释,解释代码的功能和目的。
# This function calculates the factorial of a number.
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
-
行内注释
在代码行尾部添加注释,解释代码的具体作用。
result = factorial(5) # Calculate the factorial of 5
六、利用文档字符串测试
Python的doctest模块允许在docstring中编写测试用例,并自动运行这些测试。通过这种方式,可以确保代码功能的正确性。
-
编写测试用例
在docstring中编写测试用例,使用>>>表示输入,后跟预期输出。
def square(x):
"""
Calculate the square of a number.
>>> square(2)
4
>>> square(-3)
9
"""
return x * x
-
运行doctest
使用doctest模块运行测试用例:
import doctest
doctest.testmod()
通过以上几种方法,可以在Python中有效地编写和使用帮助文档,提高代码的可读性和维护性。同时,利用工具生成外部文档,可以为大型项目提供更全面的文档支持。
相关问答FAQs:
如何在Python中创建帮助文档?
在Python中,可以使用文档字符串(docstrings)为函数、类和模块提供帮助文档。通过在定义时使用三重引号,可以为代码添加详细的说明。用户可以使用help()
函数查看这些文档,或者直接通过IDE的提示获取帮助信息。
在Python中如何使用注释提高代码可读性?
注释是解释代码的重要部分。使用井号(#)可以在代码中添加注释,提供关于特定代码块的进一步解释。这有助于其他开发者或未来的自己更好地理解代码的意图和逻辑,特别是在复杂的算法或数据处理时。
如何有效利用Python的内置帮助系统?
Python提供了内置的help()
函数,可以用来获取模块、类、方法或函数的详细信息。只需在交互式解释器中输入help(对象)
,就能看到相关的帮助文档。这样的功能非常适合快速查找用法和参数说明,提升编码效率。
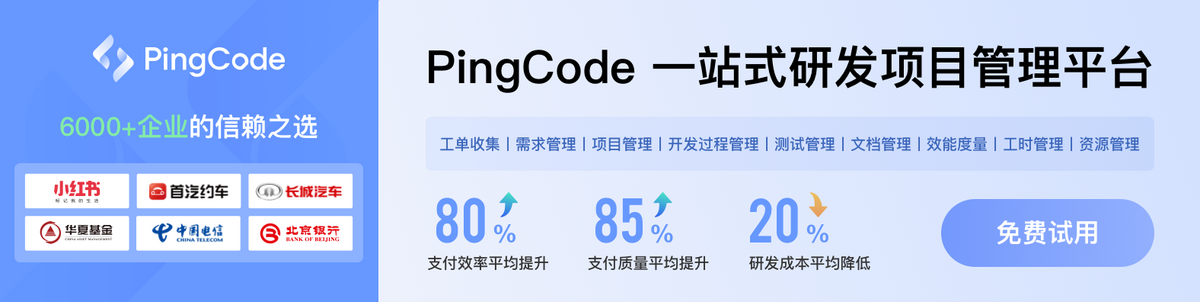