在Python中,互相调用方法的实现可以通过创建类和实例、使用模块和包、以及通过函数传递对象等方式实现。 通过类和实例的方法调用是最常见的方式。可以通过在同一个类中定义多个方法,并在这些方法中相互调用。例如,方法A可以在方法B中被调用,反之亦然。通过模块和包,可以在一个文件中定义函数,并在另一个文件中导入这些函数进行调用。函数传递对象的方式则可以通过将一个函数作为参数传递给另一个函数来实现。下面将详细介绍这几种方法。
一、类与实例中的方法互相调用
在Python中,类是对象的蓝图,而实例是类的具体对象。通过在类中定义方法,实例可以调用这些方法,并且方法可以在类内部互相调用。
1. 定义类与方法
定义一个类,并在类中添加多个方法。以下是一个示例:
class ExampleClass:
def method_a(self):
print("Method A called")
self.method_b() # 调用同一类中的另一个方法
def method_b(self):
print("Method B called")
self.method_c() # 调用同一类中的另一个方法
def method_c(self):
print("Method C called")
在上面的代码中,method_a
调用了method_b
,而method_b
又调用了method_c
。这展示了如何在同一个类中实现方法之间的互相调用。
2. 创建实例并调用方法
创建类的实例,并调用其中的方法:
example = ExampleClass()
example.method_a()
输出结果将是:
Method A called
Method B called
Method C called
这表明method_a
成功调用了method_b
,而method_b
又调用了method_c
。
二、模块与包中的方法互相调用
在Python中,模块是一个包含Python代码的文件,包是一个包含模块的目录。通过模块和包,可以实现跨文件的方法调用。
1. 创建模块与方法
创建两个模块module1.py
和module2.py
,分别定义方法:
module1.py
:
def function_one():
print("Function One called")
from module2 import function_two # 导入另一个模块中的函数
function_two()
module2.py
:
def function_two():
print("Function Two called")
2. 导入模块并调用方法
在主程序中导入模块并调用函数:
import module1
module1.function_one()
输出结果将是:
Function One called
Function Two called
这说明在module1
中,function_one
成功调用了module2
中的function_two
。
三、通过函数传递对象实现互相调用
函数可以作为参数传递给其他函数,从而实现函数间的互相调用。
1. 定义函数与参数
定义两个函数,其中一个函数接受另一个函数作为参数:
def function_a(callback):
print("Function A called")
callback()
def function_b():
print("Function B called")
2. 传递函数作为参数
调用function_a
并将function_b
作为参数传递:
function_a(function_b)
输出结果将是:
Function A called
Function B called
这说明function_a
成功调用了作为参数传递的function_b
。
四、使用闭包实现互相调用
闭包是一种可以捕获并记住其创建环境的函数。通过闭包,可以实现互相调用的功能。
1. 定义闭包
定义一个外部函数,该函数返回一个内部函数:
def outer_function():
def inner_function():
print("Inner Function called")
return inner_function
def call_closure(closure):
print("Outer Function called")
closure()
2. 使用闭包
创建闭包并调用:
closure = outer_function()
call_closure(closure)
输出结果将是:
Outer Function called
Inner Function called
这说明call_closure
成功调用了通过闭包传递的inner_function
。
五、总结
通过以上几种方式,可以实现Python中方法的互相调用。无论是通过类、模块、函数传递,还是使用闭包,每种方法都有其独特的应用场景和优势。 在实际开发中,应根据具体需求选择合适的实现方式,确保代码的可读性和可维护性。
相关问答FAQs:
Python 中如何实现不同类之间的方法调用?
在 Python 中,可以通过创建类的实例来实现不同类之间的方法调用。首先,确保你有两个类,并在一个类中创建另一个类的实例。然后,可以通过实例对象直接调用对方的方法。例如:
class ClassA:
def method_a(self):
print("Method A")
class ClassB:
def __init__(self):
self.class_a_instance = ClassA()
def method_b(self):
print("Method B")
self.class_a_instance.method_a()
b = ClassB()
b.method_b()
这样,ClassB
中的方法 method_b
可以成功调用 ClassA
中的 method_a
。
在同一类中,如何相互调用实例方法?
如果你想在同一个类的不同实例间调用方法,可以通过传递其他实例作为参数来实现。例如:
class MyClass:
def method_one(self):
print("This is method one.")
def method_two(self, another_instance):
print("This is method two.")
another_instance.method_one()
instance_a = MyClass()
instance_b = MyClass()
instance_b.method_two(instance_a)
在这个例子中,instance_b
通过 method_two
调用了 instance_a
的 method_one
。
如何在 Python 中使用装饰器实现方法的互相调用?
装饰器可以用来增强方法的功能,同时也可以实现方法之间的互相调用。你可以定义一个装饰器,然后将其应用于类的方法。例如:
def call_another_method(method):
def wrapper(self):
print("Calling another method...")
method(self)
self.method_c()
return wrapper
class MyClass:
@call_another_method
def method_a(self):
print("This is method A.")
def method_c(self):
print("This is method C.")
instance = MyClass()
instance.method_a()
在这个示例中,method_a
被装饰器包裹,从而在调用后也执行了 method_c
。这种方式使得方法之间的调用更加灵活。
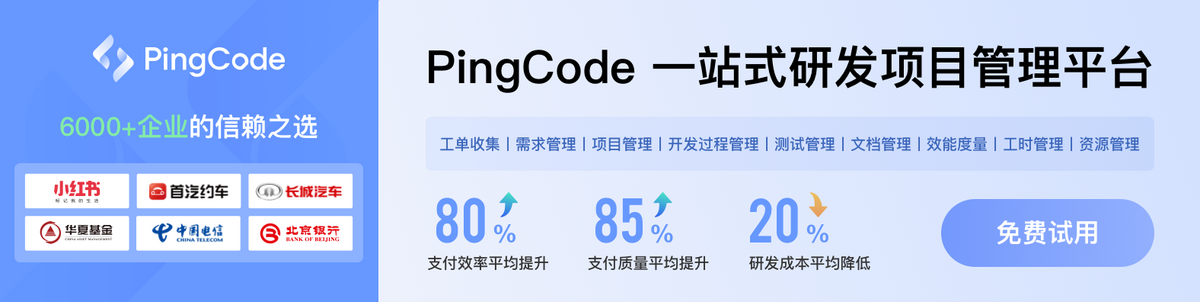