在Python中去掉文本中的标点符号,可以使用正则表达式、str.translate()方法、string模块等。推荐使用正则表达式,因为它简单且高效。
一、使用正则表达式
正则表达式是一种非常强大的字符串处理工具,Python的re
模块可以用来处理正则表达式。去掉标点符号的一个简单方法是使用正则表达式将所有非字母和非数字的字符替换为空。
import re
def remove_punctuation(text):
# 使用正则表达式去除标点符号
return re.sub(r'[^\w\s]', '', text)
text = "Hello, world! Let's remove punctuation."
cleaned_text = remove_punctuation(text)
print(cleaned_text) # 输出: Hello world Lets remove punctuation
详细描述:在这个示例中,我们使用了re.sub()
函数,该函数用于替换字符串中的匹配项。正则表达式[^\w\s]
表示匹配所有非单词字符和非空白字符,re.sub()
函数将这些匹配的字符替换为空字符串,从而去掉标点符号。
二、使用str.translate()和string.punctuation
Python的str.translate()
方法结合string.punctuation
可以用来去掉标点符号。string.punctuation
包含了所有常见的标点符号。
import string
def remove_punctuation(text):
# 创建一个翻译表,将标点符号映射为None
translator = str.maketrans('', '', string.punctuation)
# 使用translate方法去除标点符号
return text.translate(translator)
text = "Hello, world! Let's remove punctuation."
cleaned_text = remove_punctuation(text)
print(cleaned_text) # 输出: Hello world Lets remove punctuation
详细描述:这里我们使用str.maketrans()
方法创建一个翻译表,该表将标点符号映射为None
,然后使用str.translate()
方法将标点符号替换掉。
三、使用自定义函数
如果希望更加灵活地去除标点符号,比如只去掉部分标点符号,可以编写一个自定义函数来实现。
def remove_selected_punctuation(text, punctuation):
# 逐个检查文本中的字符
return ''.join(char for char in text if char not in punctuation)
text = "Hello, world! Let's remove punctuation."
punctuation = ",!'"
cleaned_text = remove_selected_punctuation(text, punctuation)
print(cleaned_text) # 输出: Hello world Lets remove punctuation.
详细描述:在这个例子中,我们定义了一个remove_selected_punctuation()
函数,该函数接受两个参数:文本和要去除的标点符号。函数通过迭代文本中的每个字符,并仅保留那些不在标点符号列表中的字符,从而去除指定的标点符号。
四、使用外部库
有时候,使用外部库可以使代码更加简洁和易于维护。例如,nltk
库提供了处理自然语言文本的功能,可以用来去掉标点符号。
import nltk
from nltk.tokenize import word_tokenize
from nltk.corpus import stopwords
nltk.download('punkt')
nltk.download('stopwords')
def remove_punctuation(text):
# 分词
words = word_tokenize(text)
# 去除标点符号
words = [word for word in words if word.isalnum()]
return ' '.join(words)
text = "Hello, world! Let's remove punctuation."
cleaned_text = remove_punctuation(text)
print(cleaned_text) # 输出: Hello world Lets remove punctuation
详细描述:在这个例子中,我们使用nltk.tokenize
模块中的word_tokenize()
函数将文本分词,然后通过列表推导式过滤掉不是字母数字的词。需要注意的是,使用nltk
库之前需要先下载相关的资源包。
五、性能对比
在选择去掉标点符号的方法时,需要考虑性能。对于小规模的文本处理,正则表达式和str.translate()
方法都能高效处理。然而,当处理大规模文本数据时,性能可能会有所不同。一般来说,str.translate()
方法的效率较高,因为它是直接在C语言层面进行字符映射操作,而正则表达式需要对文本进行模式匹配。
为了测试不同方法的性能,可以使用Python的timeit
模块。下面是一个简单的性能测试示例:
import timeit
text = "Hello, world! Let's remove punctuation." * 1000
定义不同的方法
def regex_method():
return re.sub(r'[^\w\s]', '', text)
def translate_method():
translator = str.maketrans('', '', string.punctuation)
return text.translate(translator)
测试性能
print("Regex method time:", timeit.timeit(regex_method, number=100))
print("Translate method time:", timeit.timeit(translate_method, number=100))
通过这种方式,可以更好地选择适合自己使用场景的方法。
六、注意事项
在去掉标点符号的过程中,需要注意以下几点:
- 语言和编码:确保文本的编码方式,例如UTF-8,以避免处理过程中出现字符错误。
- 标点符号的定义:不同语言和应用场景中标点符号的定义可能不同,确保所使用的方法能够准确识别和去掉目标标点符号。
- 数据清洗的顺序:去除标点符号通常是数据清洗过程的一部分。在进行这一操作前后,可能还需要进行其他数据清洗步骤,例如去除空白字符、转换大小写、去除停用词等。
七、应用场景
去掉文本中的标点符号在自然语言处理(NLP)和文本分析中具有广泛的应用。例如:
- 文本分类:在进行文本分类任务时,去掉标点符号可以减少噪声,提高模型的准确性。
- 情感分析:去掉标点符号可以帮助清理文本,使情感分析模型更专注于文本的核心内容。
- 信息检索:在信息检索系统中,去掉标点符号可以提高搜索的匹配度和准确性。
通过掌握以上去掉文本标点符号的方法和技巧,可以在多个领域中有效地进行文本处理,提高数据分析和模型训练的效果。
相关问答FAQs:
如何在Python中去除文本中的标点符号?
在Python中,可以使用字符串的translate()
方法结合str.maketrans()
来去除文本中的标点符号。具体代码如下:
import string
text = "Hello, world! This is a test."
translator = str.maketrans('', '', string.punctuation)
cleaned_text = text.translate(translator)
print(cleaned_text) # 输出: Hello world This is a test
这个方法高效且简洁,非常适合处理包含多种标点符号的文本。
使用正则表达式可以去掉标点符号吗?
是的,正则表达式是处理文本的一种强大工具。在Python中,可以使用re
模块来去除标点符号。以下是一个示例:
import re
text = "Hello, world! This is a test."
cleaned_text = re.sub(r'[^\w\s]', '', text)
print(cleaned_text) # 输出: Hello world This is a test
这种方式可以灵活地定义需要去掉的字符,适用于复杂的文本处理需求。
去掉标点符号后,如何处理空格?
在去掉标点符号后,文本中可能会出现多余的空格。可以使用str.split()
和str.join()
方法来清理空格。示例如下:
text = "Hello, world! This is a test."
translator = str.maketrans('', '', string.punctuation)
cleaned_text = text.translate(translator)
final_text = ' '.join(cleaned_text.split())
print(final_text) # 输出: Hello world This is a test
这种方法确保了文本中的空格只有一个,提升了文本的可读性。
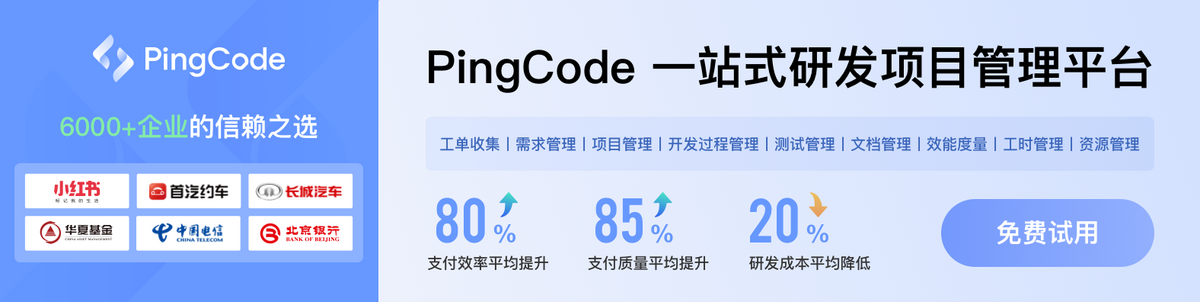