使用Python显示词频可以通过以下几种方法:使用collections模块中的Counter类、使用字典数据结构、使用正则表达式处理文本。在这里,我将详细介绍如何使用collections模块中的Counter类实现词频统计。Counter是一个专门用于计数的工具,它可以帮助我们方便地统计每个单词出现的次数。下面我将详细介绍该方法。
Counter类是collections模块中的一个子类,用于计数可哈希对象。它是一个无序的集合,元素存储为字典键,计数存储为字典值。Counter类提供了许多有用的方法,例如most_common(),可以用来获取出现频率最高的元素。通过这种方式,我们可以轻松地统计出文本中每个单词的出现频率,并按频率进行排序。
接下来,我将从多个方面详细介绍如何使用Python实现词频统计。
一、使用collections.Counter统计词频
collections模块中的Counter类是统计词频的一个非常便捷的工具。它可以用来统计可迭代对象中每个元素出现的次数。
1.1、导入模块并读取文本
首先,我们需要导入必要的模块并读取需要分析的文本数据。可以通过文件读取或者直接从字符串中获取文本。
from collections import Counter
假设我们有一个文本文件
with open('sample.txt', 'r') as file:
text = file.read()
1.2、文本预处理
在统计词频之前,我们需要对文本进行一些预处理步骤,例如将文本转换为小写、去除标点符号、分词等。
import re
将文本转换为小写
text = text.lower()
去除标点符号
text = re.sub(r'[^\w\s]', '', text)
分词
words = text.split()
1.3、统计词频
使用Counter类统计每个单词的出现次数。
word_counts = Counter(words)
1.4、获取最高频的单词
通过most_common()方法,我们可以获取出现频率最高的单词及其频率。
most_common_words = word_counts.most_common(10)
print(most_common_words)
二、使用字典统计词频
除了使用Counter类,我们也可以手动使用字典数据结构来统计词频。
2.1、初始化字典
首先,我们需要初始化一个空字典,用于存储单词及其出现次数。
word_counts = {}
2.2、遍历单词并计数
遍历分词后的单词列表,并更新字典中的计数。
for word in words:
if word in word_counts:
word_counts[word] += 1
else:
word_counts[word] = 1
2.3、排序并获取最高频的单词
我们可以使用sorted()函数对字典进行排序,以获取出现频率最高的单词。
sorted_word_counts = sorted(word_counts.items(), key=lambda kv: kv[1], reverse=True)
print(sorted_word_counts[:10])
三、使用正则表达式处理文本
正则表达式是处理文本数据时的强大工具,特别是在分词和去除非单词字符方面。
3.1、使用正则表达式分词
我们可以使用正则表达式直接从文本中提取出所有单词。
import re
words = re.findall(r'\b\w+\b', text.lower())
3.2、结合Counter统计词频
使用正则表达式提取单词后,可以结合Counter类进行词频统计。
word_counts = Counter(words)
most_common_words = word_counts.most_common(10)
print(most_common_words)
四、可视化词频数据
为了更好地理解词频数据,我们可以使用matplotlib等可视化库将结果绘制成图表。
4.1、安装matplotlib
如果您还没有安装matplotlib库,可以通过以下命令进行安装:
pip install matplotlib
4.2、绘制词频直方图
我们可以使用matplotlib绘制词频的直方图,以便更直观地展示结果。
import matplotlib.pyplot as plt
获取单词和频率
words, frequencies = zip(*most_common_words)
绘制直方图
plt.bar(words, frequencies)
plt.xlabel('Words')
plt.ylabel('Frequencies')
plt.title('Word Frequencies')
plt.show()
通过以上几种方法,我们可以使用Python高效地统计和展示文本数据中的词频信息。无论是使用collections.Counter、字典还是正则表达式,每种方法都有其独特的优势,可以根据具体需求选择最合适的方法。
相关问答FAQs:
如何使用Python计算文本中的词频?
在Python中,计算词频通常可以借助内置的collections
模块中的Counter
类。首先,您需要读取文本数据,将其分割成单词,然后使用Counter来统计每个单词的出现次数。示例代码如下:
from collections import Counter
import re
# 读取文本
with open('your_text_file.txt', 'r', encoding='utf-8') as file:
text = file.read()
# 使用正则表达式清理文本并分割成单词
words = re.findall(r'\w+', text.lower())
# 计算词频
word_counts = Counter(words)
# 打印词频
for word, count in word_counts.items():
print(f"{word}: {count}")
这段代码将计算您指定文本文件中每个单词的频率。
有哪些Python库可以帮助我更方便地显示词频?
有多个Python库可以简化词频计算和可视化的过程。nltk
(自然语言工具包)提供了丰富的文本处理功能,pandas
可以用来处理数据并生成数据框。此外,matplotlib
或seaborn
可以用于绘制词频图表。这些库结合使用,可以让您更直观地分析文本数据。以下是一个简单的示例,使用nltk
和matplotlib
来显示词频条形图:
import nltk
import matplotlib.pyplot as plt
from nltk.corpus import stopwords
from collections import Counter
nltk.download('stopwords')
# 清理文本并计算词频
words = [word for word in words if word not in stopwords.words('english')]
word_counts = Counter(words)
# 绘制词频图
plt.bar(word_counts.keys(), word_counts.values())
plt.xticks(rotation=90)
plt.show()
如何处理文本中的停用词,以便更准确地计算词频?
停用词是指在文本中频繁出现但对内容分析帮助不大的词语,如“的”、“是”、“在”等。在计算词频时,您可以选择排除这些词,以便突出更有意义的单词。使用nltk
库的停用词列表,您可以轻松地从您的文本数据中移除这些词。示例代码如下:
from nltk.corpus import stopwords
nltk.download('stopwords')
stop_words = set(stopwords.words('english'))
# 过滤停用词
filtered_words = [word for word in words if word not in stop_words]
word_counts = Counter(filtered_words)
通过这种方式,您可以更准确地分析文本中的重要词汇。
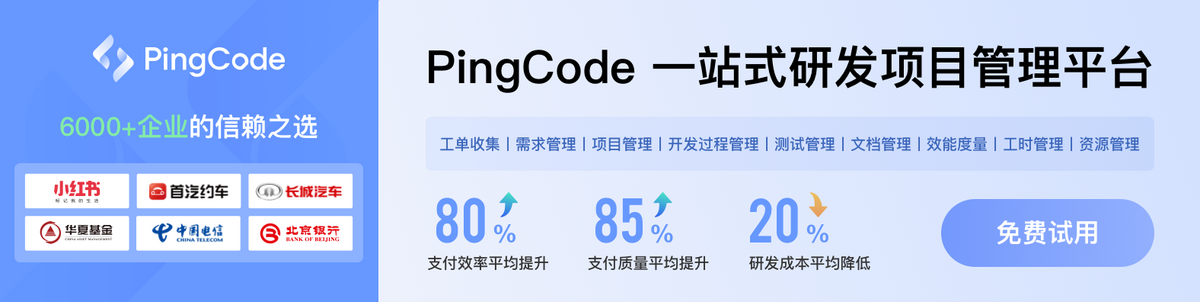