在Python中调节字体粗细主要通过使用图形库来实现,常用的库包括Matplotlib、Tkinter和Pygame等。使用Matplotlib、Tkinter和Pygame等库,可以通过设置字体的权重或样式来调节字体粗细。通过属性设置、选项调整等方式实现不同的字体效果。其中,Matplotlib和Tkinter通过设置字体的权重选项(如'normal'、'bold')来改变字体粗细;而Pygame则通过指定字体文件和大小来实现更灵活的字体样式控制。接下来,我们将详细介绍如何使用这些库来调节字体粗细。
一、MATPLOTLIB中字体粗细的调节
Matplotlib是一个广泛用于数据可视化的库,提供了强大的绘图功能。调节字体粗细通常通过设置字体属性来实现。
- 设置字体属性
Matplotlib中的字体粗细可以通过fontweight
属性来设置。常用的选项包括'normal'、'bold'、'light'等。例如,使用plt.title
或plt.xlabel
等函数时,可以传递fontweight
参数来控制标题或标签的字体粗细。
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [10, 20, 25, 30])
plt.title('Example Plot', fontweight='bold')
plt.xlabel('X-axis', fontweight='light')
plt.ylabel('Y-axis', fontweight='normal')
plt.show()
- 全局设置字体粗细
除了单独设置某个文本元素的字体粗细,还可以通过rcParams
全局设置字体的默认粗细。这样,所有的文本元素都会应用相同的字体粗细。
import matplotlib.pyplot as plt
plt.rcParams['font.weight'] = 'bold'
plt.plot([1, 2, 3, 4], [10, 20, 25, 30])
plt.title('Example Plot')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.show()
二、TKINTER中字体粗细的调节
Tkinter是Python的标准GUI库,常用于创建桌面应用程序。调节字体粗细可以通过font
模块中的Font
类来实现。
- 使用Font类设置字体粗细
Tkinter的Font
类允许我们定义字体的样式,包括字体名称、大小和粗细。粗细通过weight
参数设置。
import tkinter as tk
from tkinter import font
root = tk.Tk()
bold_font = font.Font(family='Helvetica', size=12, weight='bold')
label = tk.Label(root, text="Bold Text", font=bold_font)
label.pack()
normal_font = font.Font(family='Helvetica', size=12, weight='normal')
label2 = tk.Label(root, text="Normal Text", font=normal_font)
label2.pack()
root.mainloop()
- 动态改变字体粗细
Tkinter允许动态改变字体的属性,包括粗细。可以通过修改Font
对象的属性来实现。
import tkinter as tk
from tkinter import font
def toggle_weight():
current_weight = bold_font.cget('weight')
new_weight = 'normal' if current_weight == 'bold' else 'bold'
bold_font.config(weight=new_weight)
root = tk.Tk()
bold_font = font.Font(family='Helvetica', size=12, weight='bold')
label = tk.Label(root, text="Toggle Bold Text", font=bold_font)
label.pack()
button = tk.Button(root, text="Toggle Weight", command=toggle_weight)
button.pack()
root.mainloop()
三、PYGAME中字体粗细的调节
Pygame是一个用于制作2D游戏的库,提供了丰富的多媒体功能。虽然Pygame没有直接的字体粗细选项,但可以通过选择不同的字体文件来实现。
- 加载字体文件
Pygame允许加载自定义字体文件,并通过设置字体大小来间接调整字体的粗细。粗字体通常需要提前准备好对应的字体文件。
import pygame
pygame.init()
screen = pygame.display.set_mode((400, 300))
pygame.display.set_caption("Font Weight Example")
Load a bold font
bold_font = pygame.font.Font('freesansbold.ttf', 32)
Render the text
text_surface = bold_font.render('Bold Text', True, (255, 255, 255))
screen.blit(text_surface, (50, 100))
pygame.display.flip()
Wait for a while to view the result
pygame.time.wait(2000)
pygame.quit()
- 使用系统字体
Pygame还可以使用系统字体,通过pygame.font.SysFont
指定字体样式和大小,选择系统中可用的粗体字体。
import pygame
pygame.init()
screen = pygame.display.set_mode((400, 300))
pygame.display.set_caption("System Font Example")
Use a system font with bold style
bold_font = pygame.font.SysFont('Arial', 32, bold=True)
Render the text
text_surface = bold_font.render('Bold Text', True, (255, 255, 255))
screen.blit(text_surface, (50, 100))
pygame.display.flip()
Wait for a while to view the result
pygame.time.wait(2000)
pygame.quit()
四、总结
通过上述介绍,我们了解了在Python中如何使用不同的库来调节字体的粗细。每种库都有其特定的方式和适用场景,开发者可以根据实际需求选择合适的方案:
- Matplotlib适合数据可视化中需要控制图表文本的样式。
- Tkinter适合桌面应用程序中需要动态调整文本的场合。
- Pygame适合游戏开发中需要多媒体展示的场景。
掌握这些技巧将有助于在不同的Python应用中实现更丰富的文本样式效果。
相关问答FAQs:
如何在Python中改变文本的字体样式?
在Python中,可以使用不同的库来改变文本的字体样式。例如,使用Matplotlib库,可以通过设置fontweight
参数来调节字体粗细。此外,Tkinter也允许通过font
参数来定义字体的粗细。了解这些库的文档能够帮助您更好地掌握字体样式的调整。
使用哪些库可以在Python中设置字体粗细?
Python中有几个常用的库可以用来设置字体粗细,如Matplotlib、PIL(Pillow)、Tkinter等。Matplotlib专注于图形绘制,而PIL则用于图像处理,Tkinter适合构建图形用户界面。根据您的需求选择合适的库将使字体样式的调整更加便捷。
如何在Matplotlib中实现不同的字体粗细效果?
在Matplotlib中,可以通过在绘图函数中添加fontdict
参数来控制字体的粗细。例如,可以设置fontdict={'weight': 'bold'}
来实现粗体效果。使用其他相关属性,如size
和family
,可以进一步调整字体的外观,以达到您想要的效果。
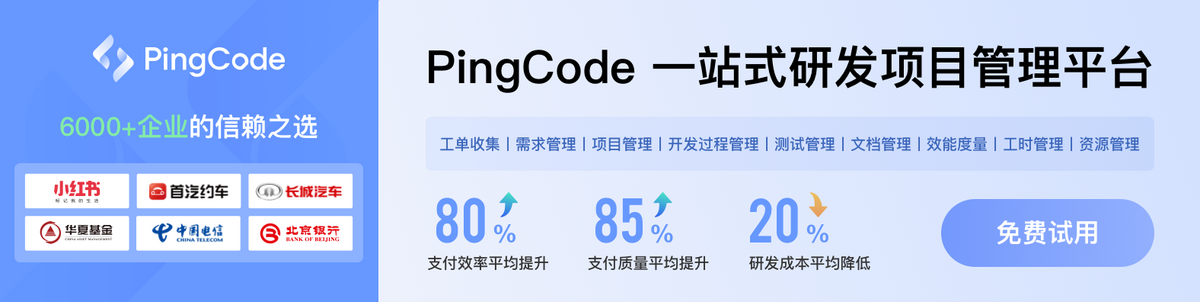