当Python运行卡住时,可以通过设置超时、使用多线程或多进程、监控程序状态等方法来跳过卡住的部分。其中,设置超时是最直接的方法,具体可以使用signal
模块或者concurrent.futures
模块来实现。通过设置超时,程序在等待某个操作完成时,如果超出了指定的时间,就会抛出异常,从而避免程序卡住。下面将详细介绍这些方法。
一、设置超时
- 使用signal模块设置超时
在Python中,signal
模块可以用于设置超时信号,特别适用于在Linux系统下的同步代码中。
import signal
def handler(signum, frame):
raise TimeoutError("Operation timed out")
设置信号处理函数
signal.signal(signal.SIGALRM, handler)
设置超时时间为5秒
signal.alarm(5)
try:
# 可能会卡住的操作
result = long_running_function()
finally:
# 取消信号
signal.alarm(0)
在这个代码中,signal.alarm(5)
设定了一个5秒的超时,如果在这段时间内函数没有返回结果,则会引发TimeoutError
,从而避免程序卡死。
- 使用concurrent.futures模块
concurrent.futures
模块可以在多线程或多进程中设置超时,更加跨平台。
from concurrent.futures import ThreadPoolExecutor, TimeoutError
def long_running_function():
# 模拟长时间运行的函数
pass
executor = ThreadPoolExecutor(max_workers=1)
future = executor.submit(long_running_function)
try:
# 设置超时时间
result = future.result(timeout=5)
except TimeoutError:
print("Operation timed out")
通过ThreadPoolExecutor
或ProcessPoolExecutor
,可以在5秒后自动抛出TimeoutError
。
二、使用多线程或多进程
- 多线程
使用多线程可以将容易卡住的操作放到单独的线程中执行,当主线程发现任务卡住时,可以选择终止该线程。
import threading
def long_running_function():
# 模拟长时间运行的函数
pass
thread = threading.Thread(target=long_running_function)
thread.start()
thread.join(timeout=5) # 等待5秒
if thread.is_alive():
print("Operation is still running, consider terminating it.")
- 多进程
与多线程类似,多进程可以在一个进程卡住时终止它。
from multiprocessing import Process
def long_running_function():
# 模拟长时间运行的函数
pass
process = Process(target=long_running_function)
process.start()
process.join(timeout=5) # 等待5秒
if process.is_alive():
process.terminate()
print("Process terminated due to timeout.")
三、监控程序状态
- 日志记录
通过日志记录可以监控程序的执行流程,并在程序卡住时查看日志以定位问题。
import logging
logging.basicConfig(level=logging.INFO)
def long_running_function():
logging.info("Starting long running function")
# 模拟长时间运行的函数
logging.info("Finished long running function")
long_running_function()
- 健康检查
在长期运行的程序中,可以定期进行健康检查,确保程序在正常运行。
import time
def health_check():
while True:
# 检查程序的健康状况
time.sleep(5)
check_thread = threading.Thread(target=health_check)
check_thread.start()
四、优化代码和算法
- 分析瓶颈
使用分析工具如cProfile或line_profiler可以帮助定位代码中的瓶颈部分。
import cProfile
def long_running_function():
# 模拟长时间运行的函数
pass
cProfile.run('long_running_function()')
- 优化算法
如果某段代码经常卡住,可以考虑优化算法以提高效率。例如,使用更高效的数据结构或算法。
五、使用外部工具
- 使用守护进程
守护进程可以监控主程序的状态,并在其卡住时进行重启。
- 使用第三方库
一些第三方库如timeout-decorator可以方便地为函数设置超时。
from timeout_decorator import timeout, TimeoutError
@timeout(5)
def long_running_function():
# 模拟长时间运行的函数
pass
try:
long_running_function()
except TimeoutError:
print("Function timed out")
通过上述方法,可以有效避免Python程序在运行时卡住,并确保其可以继续执行其他任务。根据具体情况选择合适的方法进行实现,可以提高程序的鲁棒性和稳定性。
相关问答FAQs:
在Python程序运行时,如何判断是卡住还是正常执行?
在Python程序中,如果发现程序运行时间超过预期,可以通过添加日志输出或使用调试工具来判断程序是否卡住。使用print()
语句在关键步骤打印状态信息,可以帮助追踪程序的执行进度。此外,利用Python的trace
模块或集成开发环境(IDE)的调试功能,能够更清晰地查看代码运行的状态。
遇到卡住的情况,如何安全终止Python进程?
如果Python程序卡住,可以通过键盘快捷键Ctrl + C
在终端中安全终止程序。这会引发KeyboardInterrupt
异常,允许你在捕获异常后进行必要的清理操作。如果是在图形用户界面(GUI)中运行,通常可以通过关闭窗口或使用任务管理器来结束进程。
如何优化Python代码以减少运行卡住的可能性?
优化Python代码可以有效降低卡住的几率。可以考虑使用高效的数据结构和算法,避免不必要的循环和复杂的嵌套结构。同时,使用multiprocessing
或asyncio
等模块可以实现并行处理,从而提高程序的执行效率。此外,定期进行代码重构和性能测试也有助于识别和解决潜在的性能瓶颈。
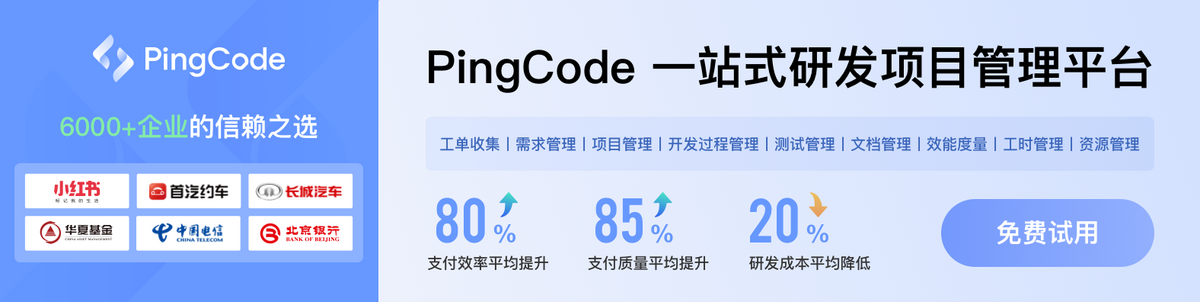