在Python中实现语音模块可以通过以下几种方式:使用speech_recognition
库进行语音识别、使用gTTS
库进行文本转语音、集成第三方API如Google Cloud Speech-to-Text和Text-to-Speech。下面将详细介绍如何使用这些方法实现语音模块。
一、使用speech_recognition
进行语音识别
speech_recognition
是一个功能强大且易于使用的Python库,可以用来将语音转换为文本。它支持多个语音识别引擎和API,包括Google Web Speech API、CMU Sphinx等。
- 安装并导入库
首先,你需要安装speech_recognition
库:
pip install SpeechRecognition
然后在你的Python脚本中导入它:
import speech_recognition as sr
- 语音识别实现步骤
- 创建识别器实例:创建一个
Recognizer
对象,它将用于处理音频数据。
recognizer = sr.Recognizer()
- 加载音频数据:可以从麦克风或音频文件中获取音频数据。
with sr.Microphone() as source:
print("Please wait. Calibrating microphone...")
# 侦听背景噪音并调整
recognizer.adjust_for_ambient_noise(source, duration=5)
print("Microphone calibrated, start speaking.")
audio = recognizer.listen(source)
- 识别语音:使用识别器对象将音频转换为文本。
try:
print("You said: " + recognizer.recognize_google(audio))
except sr.UnknownValueError:
print("Google Speech Recognition could not understand audio")
except sr.RequestError as e:
print(f"Could not request results from Google Speech Recognition service; {e}")
二、使用gTTS
进行文本转语音
gTTS
(Google Text-to-Speech)是一个Python库,允许你使用Google的TTS API将文本转换为语音。
- 安装并导入库
首先,你需要安装gTTS
库:
pip install gTTS
然后在你的Python脚本中导入它:
from gtts import gTTS
import os
- 实现文本转语音
- 创建TTS对象:将文本转换为语音对象。
tts = gTTS(text='Hello, world!', lang='en')
- 保存音频文件:将生成的语音保存为音频文件。
tts.save("hello.mp3")
- 播放音频文件:使用系统的音频播放器播放生成的音频文件。
os.system("mpg321 hello.mp3")
三、使用Google Cloud Speech-to-Text和Text-to-Speech API
Google Cloud提供了强大的语音识别和生成服务,可以处理复杂的语音任务。
- 配置Google Cloud环境
首先,你需要在Google Cloud平台上启用Speech-to-Text和Text-to-Speech API,并下载服务账户的JSON密钥文件。
- 安装Google Cloud客户端库
pip install google-cloud-speech
pip install google-cloud-texttospeech
- 使用Speech-to-Text API
- 导入库并设置环境变量
import os
from google.cloud import speech
os.environ["GOOGLE_APPLICATION_CREDENTIALS"] = "path/to/your/credentials.json"
- 创建客户端并识别音频
client = speech.SpeechClient()
with open("path/to/audio.wav", "rb") as audio_file:
content = audio_file.read()
audio = speech.RecognitionAudio(content=content)
config = speech.RecognitionConfig(
encoding=speech.RecognitionConfig.AudioEncoding.LINEAR16,
sample_rate_hertz=16000,
language_code="en-US",
)
response = client.recognize(config=config, audio=audio)
for result in response.results:
print(f"Transcript: {result.alternatives[0].transcript}")
- 使用Text-to-Speech API
- 导入库并创建客户端
from google.cloud import texttospeech
tts_client = texttospeech.TextToSpeechClient()
- 合成语音
synthesis_input = texttospeech.SynthesisInput(text="Hello, world!")
voice = texttospeech.VoiceSelectionParams(
language_code="en-US",
ssml_gender=texttospeech.SsmlVoiceGender.NEUTRAL,
)
audio_config = texttospeech.AudioConfig(
audio_encoding=texttospeech.AudioEncoding.MP3
)
response = tts_client.synthesize_speech(
input=synthesis_input, voice=voice, audio_config=audio_config
)
with open("output.mp3", "wb") as out:
out.write(response.audio_content)
print('Audio content written to file "output.mp3"')
四、集成第三方API
除了Google Cloud,其他云服务提供商如Amazon AWS、Microsoft Azure等也提供语音识别和生成服务。你可以根据需求选择合适的API,并通过其官方文档进行集成。
- Amazon AWS Polly和Transcribe
AWS提供了Polly(文本转语音)和Transcribe(语音转文本)服务。你需要通过Boto3库进行集成。
- Microsoft Azure Speech Services
Azure提供了强大的语音服务,通过Azure SDK可以轻松集成语音识别和合成。
五、总结
通过以上几种方法,Python可以实现功能丰富的语音模块。根据实际需求,你可以选择本地开源库或云服务API来实现语音识别和合成功能。在选择工具和服务时,需要考虑准确性、响应速度、支持的语言和成本等因素。
相关问答FAQs:
如何在Python中实现语音识别功能?
在Python中,可以使用库如SpeechRecognition来实现语音识别功能。用户可以通过安装该库并结合PyAudio进行音频输入,轻松地将语音转换为文本。此外,SpeechRecognition支持多种语音识别引擎,包括Google Web Speech API,用户只需简单配置即可开始使用。
有哪些常用的Python库可以实现语音合成?
Python中有几个常用的库可以实现语音合成,比如gTTS(Google Text-to-Speech)和pyttsx3。gTTS可以将文本转换为音频文件,并支持多种语言,而pyttsx3则是一个离线库,支持多种语音选项,用户可以根据自己的需求选择合适的库进行语音合成。
如何处理语音数据以提高识别准确性?
为了提高语音识别的准确性,用户可以对音频数据进行预处理。这包括去噪、调整音量以及清晰化语音信号等方法。此外,使用高质量的麦克风录音和适当的环境设置也能有效提高识别效果。可以考虑使用librosa库来处理和分析音频数据,以便更好地适应语音识别的需求。
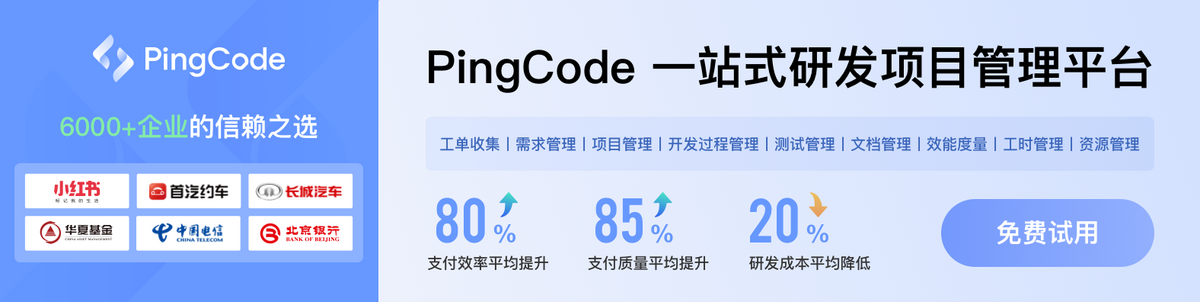