在Python中实现批量替换的主要方法包括使用字符串的replace方法、正则表达式模块re以及利用字典进行替换。其中,利用字典进行替换是一种高效且灵活的方法,尤其适用于多对替换需求。下面将详细展开如何使用字典进行批量替换。
使用字典进行批量替换可以通过构建一个包含所有待替换键值对的字典,并利用正则表达式模块re
的sub
函数来实现。首先,构建一个字典,其中键为待替换的字符串,值为替换后的字符串。然后,将字典的键构造成一个正则表达式模式,利用re.sub
方法对目标文本进行替换。这样的方法不仅可以实现单个字符或字符串的替换,还可以处理多个不同字符或字符串的批量替换。这种方法的优势在于其灵活性和可扩展性,可以轻松地添加或修改替换规则。
接下来,我们将详细探讨Python中实现批量替换的各种方法和技巧。
一、字符串的replace方法
Python内置的字符串方法replace
是进行字符串替换的最直接方式。虽然replace
方法不能直接进行批量替换,但通过循环可以实现对多个字符串的替换。
- 单个字符串替换
replace
方法可以直接用于替换一个字符串中的某一部分。其基本语法是str.replace(old, new[, maxreplace])
,其中old
是要被替换的子字符串,new
是替换后的字符串,maxreplace
是可选参数,指定替换的最大次数。
text = "Hello World! Welcome to the world of Python."
text = text.replace("world", "universe")
print(text)
- 多个字符串替换
要进行多个字符串替换,可以使用循环配合replace
方法。比如:
replacements = {"Hello": "Hi", "World": "Earth", "Python": "Programming"}
text = "Hello World! Welcome to the world of Python."
for old, new in replacements.items():
text = text.replace(old, new)
print(text)
二、正则表达式模块re
使用正则表达式模块re
可以更灵活地进行字符串替换,尤其适用于复杂的替换规则。
- 基本用法
re.sub(pattern, repl, string, count=0, flags=0)
用于替换字符串中符合正则表达式模式的部分。pattern
是正则表达式模式,repl
是替换后的字符串。
import re
text = "The rain in Spain"
new_text = re.sub(r"ain", "xyz", text)
print(new_text)
- 批量替换
通过构建一个合适的正则表达式模式,可以实现多个字符串的批量替换。
import re
def multiple_replace(replacement_dict, text):
pattern = re.compile("|".join(map(re.escape, replacement_dict.keys())))
return pattern.sub(lambda match: replacement_dict[match.group(0)], text)
replacements = {"rain": "snow", "Spain": "France"}
text = "The rain in Spain"
new_text = multiple_replace(replacements, text)
print(new_text)
三、利用字典进行批量替换
使用字典进行批量替换是一个高效且灵活的方法。通过正则表达式模块re
的sub
方法,可以实现复杂的批量替换。
- 构建字典
首先,构建一个包含所有待替换键值对的字典。
replacements = {"rain": "snow", "Spain": "France", "The": "A"}
- 正则表达式模式
将字典的键构造成一个正则表达式模式,并利用re.sub
方法对目标文本进行替换。
import re
def multiple_replace(replacement_dict, text):
# 构建正则表达式模式
pattern = re.compile("|".join(map(re.escape, replacement_dict.keys())))
return pattern.sub(lambda match: replacement_dict[match.group(0)], text)
text = "The rain in Spain"
new_text = multiple_replace(replacements, text)
print(new_text)
四、使用pandas进行数据替换
当处理数据表格时,pandas
库提供了便捷的方法进行批量替换。
- 替换单列中的值
pandas
中的replace
方法可以对DataFrame中的某一列进行替换。
import pandas as pd
data = {'Country': ['Spain', 'USA', 'Italy'], 'Capital': ['Madrid', 'Washington', 'Rome']}
df = pd.DataFrame(data)
replacements = {"Spain": "France", "USA": "Canada"}
df['Country'] = df['Country'].replace(replacements)
print(df)
- 替换整个DataFrame中的值
如果需要替换整个DataFrame中的值,也可以使用replace
方法。
replacements = {"Spain": "France", "USA": "Canada", "Rome": "Paris"}
df = df.replace(replacements)
print(df)
五、结合函数与数据结构进行高级替换
在某些复杂场景下,可以结合函数与数据结构(如字典、列表)进行高级替换。
- 使用函数进行条件替换
可以定义一个函数,根据特定的条件来替换字符串。
def custom_replace(word):
if word == "Spain":
return "France"
elif word == "USA":
return "Canada"
return word
text = "The rain in Spain and the USA"
words = text.split()
new_words = [custom_replace(word) for word in words]
new_text = " ".join(new_words)
print(new_text)
- 利用数据结构的灵活性
通过结合字典、列表等数据结构,可以实现更加灵活的替换规则。
def replace_using_dict(text, replacement_dict):
for old, new in replacement_dict.items():
text = text.replace(old, new)
return text
replacements = {"rain": "snow", "Spain": "France"}
text = "The rain in Spain"
new_text = replace_using_dict(text, replacements)
print(new_text)
综上所述,Python提供了多种实现批量替换的方法,从简单的字符串替换到复杂的正则表达式替换,再到结合数据结构的高级替换。选择合适的方法可以提高代码的效率和可读性,满足不同场景下的需求。
相关问答FAQs:
如何在Python中使用正则表达式进行批量替换?
在Python中,可以通过re
模块来实现正则表达式的批量替换。使用re.sub()
函数可以指定要匹配的模式和替换的字符串。例如,使用re.sub(r'old_text', 'new_text', original_string)
可以将字符串中的所有old_text
替换为new_text
。通过这种方式,可以灵活地处理更复杂的文本替换需求。
有哪些常用的库可以帮助实现批量替换?
除了内置的re
模块外,还有其他一些库可以帮助实现批量替换。比如,pandas
库非常适合处理表格数据,可以通过DataFrame.replace()
方法进行批量替换。在处理大规模文本数据时,fileinput
库也能方便地替换文件中的内容,尤其是处理多行文本时。
在处理大文件时,如何优化Python的批量替换性能?
对于大文件的批量替换,采用逐行读取和写入的方式可以显著提升性能。使用with open()
语句逐行读取文件内容,并在内存中执行替换操作,最后将结果写入新文件或覆盖原文件。这样可以避免一次性将整个文件加载到内存中,从而提高效率并减少内存占用。
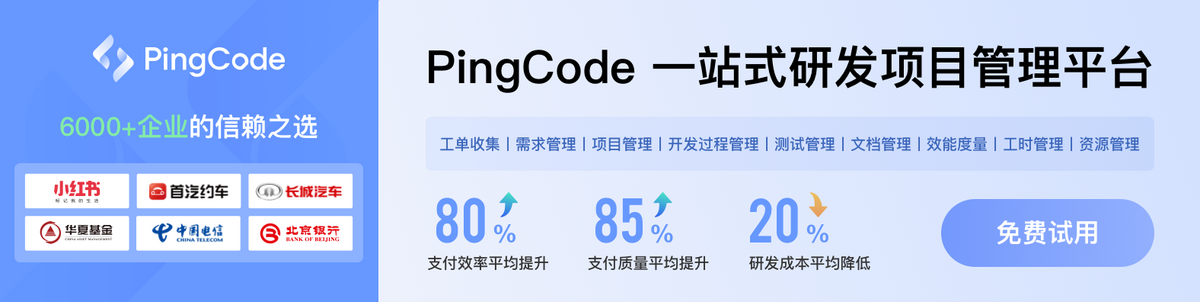