在Python中,复制文件的常用方法有使用shutil
模块、os
模块、以及subprocess
模块。 其中,shutil
模块是最常用也是最简单的方法。它提供了高层次的文件操作功能,能够轻松地复制文件和文件夹。下面将详细介绍这些方法,并结合具体代码示例进行说明。
一、使用SHUTIL模块
shutil
模块是Python标准库的一部分,专门用于文件和文件夹的高层次操作。
1.1 复制单个文件
使用shutil.copy()
函数可以复制单个文件。这个函数需要两个参数:源文件路径和目标文件路径。
import shutil
def copy_file(source, destination):
try:
shutil.copy(source, destination)
print(f"File {source} copied to {destination}")
except Exception as e:
print(f"Error occurred: {e}")
示例用法
copy_file('source.txt', 'destination.txt')
在这个例子中,source.txt
是要复制的文件,而destination.txt
是复制后的文件名。
1.2 复制文件并保留元数据
如果需要保留文件的元数据(如权限、最后访问时间等),可以使用shutil.copy2()
。
import shutil
def copy_file_with_metadata(source, destination):
try:
shutil.copy2(source, destination)
print(f"File {source} copied to {destination} with metadata")
except Exception as e:
print(f"Error occurred: {e}")
示例用法
copy_file_with_metadata('source.txt', 'destination.txt')
这个函数与shutil.copy()
的用法相似,但会保留更多的文件信息。
1.3 复制整个目录
使用shutil.copytree()
可以复制整个目录。它也需要两个参数:源目录路径和目标目录路径。
import shutil
def copy_directory(source_dir, destination_dir):
try:
shutil.copytree(source_dir, destination_dir)
print(f"Directory {source_dir} copied to {destination_dir}")
except Exception as e:
print(f"Error occurred: {e}")
示例用法
copy_directory('source_folder', 'destination_folder')
在这个例子中,整个source_folder
目录被复制到destination_folder
。
二、使用OS模块
os
模块可以提供更底层的文件操作功能。
2.1 使用OS模块复制文件
虽然os
模块没有直接的复制功能,但可以通过读取和写入文件实现复制。
import os
def copy_file_os(source, destination):
try:
with open(source, 'rb') as src_file:
with open(destination, 'wb') as dest_file:
dest_file.write(src_file.read())
print(f"File {source} copied to {destination} using os module")
except Exception as e:
print(f"Error occurred: {e}")
示例用法
copy_file_os('source.txt', 'destination.txt')
这种方法适用于简单的文件复制,但不处理元数据。
三、使用SUBPROCESS模块
subprocess
模块可以用来调用系统命令进行文件复制。
3.1 使用SUBPROCESS调用系统命令
在不同的操作系统中,命令可能不同。例如,在Windows中可以使用copy
命令,而在Linux/Unix中使用cp
命令。
import subprocess
def copy_file_subprocess(source, destination):
try:
if os.name == 'nt': # Windows
subprocess.run(['copy', source, destination], shell=True, check=True)
else: # Linux/Unix
subprocess.run(['cp', source, destination], check=True)
print(f"File {source} copied to {destination} using subprocess module")
except Exception as e:
print(f"Error occurred: {e}")
示例用法
copy_file_subprocess('source.txt', 'destination.txt')
这种方法的灵活性较高,但需要注意跨平台的兼容性。
四、错误处理与注意事项
在复制文件时,可能会遇到各种错误,如文件不存在、权限不足等。良好的错误处理可以提高程序的稳定性。
4.1 常见错误处理
文件不存在
在复制文件之前,首先检查源文件是否存在。
import os
def check_file_exists(file_path):
if not os.path.exists(file_path):
print(f"File {file_path} does not exist.")
return False
return True
权限不足
尝试在具有适当权限的环境中运行脚本,或者更改文件权限。
目标文件已存在
在复制文件之前,可以检查目标文件是否已存在,并选择是否覆盖。
import os
def check_file_overwrite(destination):
if os.path.exists(destination):
choice = input(f"File {destination} already exists. Overwrite? (y/n): ")
if choice.lower() != 'y':
print("Operation cancelled.")
return False
return True
通过结合以上的方法和注意事项,你可以在Python中有效地复制文件和目录。选择适合你的项目需求的复制方法,并确保处理可能出现的错误。
相关问答FAQs:
如何使用Python复制文件到指定目录?
使用Python复制文件可以通过内置的shutil
模块来实现。你可以使用shutil.copy(source, destination)
函数,其中source
是你要复制的文件路径,destination
是你希望将文件复制到的位置。确保目标目录存在,否则会抛出错误。
在Python中复制文件时如何处理文件权限?
在复制文件时,shutil.copy
只会复制文件内容和权限,如果需要复制更多的元数据(如创建时间、修改时间等),可以使用shutil.copy2(source, destination)
。这样可以确保复制文件的同时也保留了所有的文件元数据。
如果要复制一个文件夹及其所有内容,应该怎么做?
若要复制整个文件夹及其所有子文件和子文件夹,可以使用shutil.copytree(source_dir, destination_dir)
函数。这个函数会递归复制源目录中的所有内容到指定的目标目录。需要注意的是,目标目录必须不存在,否则会引发异常。
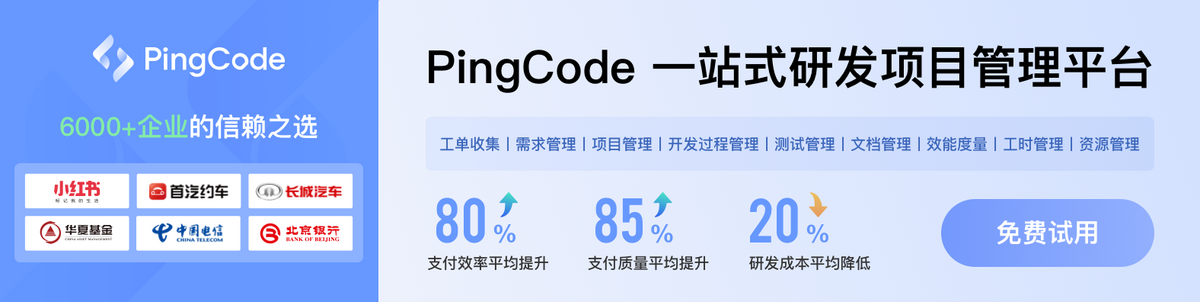