在JSP中调用Python的方式有多种,主要包括使用ProcessBuilder、通过HTTP请求调用Python Web服务、以及使用Jython等方法。 其中,使用ProcessBuilder 是一种简单直接的方式,它允许Java程序直接运行外部的Python脚本。通过HTTP请求调用Python Web服务 则适用于需要更复杂的交互的情况,比如需要频繁调用或需要与Python服务进行数据交换。使用Jython 是一种较为复杂的方案,它允许在Java中直接运行Python代码,但需要对项目环境进行一定的配置。
一、使用ProcessBuilder调用Python脚本
使用ProcessBuilder来调用Python脚本是一种简单直接的方法,适合于不需要频繁调用或与Python进行复杂数据交换的场景。
1.1、ProcessBuilder的基础用法
ProcessBuilder是Java中用于启动和管理操作系统进程的类。通过ProcessBuilder,我们可以直接运行Python脚本并获取其输出。
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class PythonExecutor {
public static void main(String[] args) {
try {
// 创建ProcessBuilder实例,指定要运行的Python脚本和参数
ProcessBuilder pb = new ProcessBuilder("python", "script.py", "arg1", "arg2");
// 启动进程
Process process = pb.start();
// 获取Python脚本的输出
BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
// 等待进程结束
int exitCode = process.waitFor();
System.out.println("Exited with code: " + exitCode);
} catch (Exception e) {
e.printStackTrace();
}
}
}
1.2、处理输入和错误流
在使用ProcessBuilder时,我们不仅可以读取标准输出流,还可以处理标准错误流,以便更好地调试和处理异常。
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class PythonExecutor {
public static void main(String[] args) {
try {
ProcessBuilder pb = new ProcessBuilder("python", "script.py", "arg1", "arg2");
Process process = pb.start();
// 获取标准输出和错误输出
BufferedReader stdInput = new BufferedReader(new InputStreamReader(process.getInputStream()));
BufferedReader stdError = new BufferedReader(new InputStreamReader(process.getErrorStream()));
// 读取标准输出
String s;
while ((s = stdInput.readLine()) != null) {
System.out.println("Output: " + s);
}
// 读取错误输出
while ((s = stdError.readLine()) != null) {
System.err.println("Error: " + s);
}
int exitCode = process.waitFor();
System.out.println("Exited with code: " + exitCode);
} catch (Exception e) {
e.printStackTrace();
}
}
}
二、通过HTTP请求调用Python Web服务
这种方法适合于需要与Python进行频繁交互和数据交换的场景,特别是在微服务架构中。
2.1、搭建简单的Python Web服务
我们可以使用Flask或Django等框架在Python中快速搭建一个Web服务。
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route('/process', methods=['POST'])
def process_data():
data = request.json
result = {'processed_data': data['key'] * 2} # 简单的数据处理
return jsonify(result)
if __name__ == '__main__':
app.run(debug=True)
2.2、在JSP/Java中发送HTTP请求
我们可以使用Java中的HttpURLConnection或第三方库(如Apache HttpClient)来发送HTTP请求。
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
public class HttpRequestExample {
public static void main(String[] args) {
try {
URL url = new URL("http://localhost:5000/process");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("POST");
conn.setRequestProperty("Content-Type", "application/json; utf-8");
conn.setRequestProperty("Accept", "application/json");
conn.setDoOutput(true);
String jsonInputString = "{\"key\": 5}";
try (OutputStream os = conn.getOutputStream()) {
byte[] input = jsonInputString.getBytes("utf-8");
os.write(input, 0, input.length);
}
// 读取响应
try (BufferedReader br = new BufferedReader(
new InputStreamReader(conn.getInputStream(), "utf-8"))) {
StringBuilder response = new StringBuilder();
String responseLine = null;
while ((responseLine = br.readLine()) != null) {
response.append(responseLine.trim());
}
System.out.println(response.toString());
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
三、使用Jython
Jython是一种可以在Java中运行Python代码的实现,但它对Python版本的支持有限(通常支持Python 2.x)。
3.1、Jython的安装与配置
首先,需要下载并安装Jython。安装后,在Java项目中引入Jython的jar包。
3.2、在Java中运行Python代码
Jython允许我们在Java中直接嵌入和运行Python代码。
import org.python.util.PythonInterpreter;
public class JythonExample {
public static void main(String[] args) {
PythonInterpreter interpreter = new PythonInterpreter();
// 执行Python代码
interpreter.exec("import sys");
interpreter.exec("print(sys.version)");
// 传递参数并调用Python函数
interpreter.set("a", 10);
interpreter.set("b", 20);
interpreter.exec("result = a + b");
int result = interpreter.get("result", Integer.class);
System.out.println("Result: " + result);
}
}
四、选择合适的方法
在选择如何在JSP中调用Python时,需要根据项目的具体需求做出选择:
- 对于简单的、一次性的任务,可以选择使用ProcessBuilder。
- 对于需要频繁交互的场景,推荐使用HTTP请求调用Python Web服务。
- 对于需要在Java中嵌入Python代码的场景,可以考虑使用Jython,但要注意其对Python版本的支持限制。
通过这些方法,我们可以在Java和Python之间实现良好的互操作性,充分利用两种语言的优势。
相关问答FAQs:
如何在JSP中集成Python脚本?
在JSP中集成Python脚本可以通过多种方式实现,例如使用Java Runtime类调用Python解释器,或使用web服务进行交互。可以通过Java代码执行Python脚本并获取输出,确保Python环境已安装并配置正确。将Python脚本的路径传递给Runtime类的exec方法,并处理返回的结果。
使用JSP调用Python时需要注意哪些安全性问题?
在使用JSP调用Python脚本时,确保输入的参数已经过严格验证,以防止注入攻击。同时,避免在服务器上直接执行来自用户输入的命令,最好采用预定义的命令和参数。此外,确保Python脚本的权限设置合理,以减少潜在的安全漏洞。
有哪些常见的场景适合使用JSP调用Python?
JSP调用Python适合处理数据分析、机器学习模型推理或复杂计算等场景。尤其是当应用需要利用Python丰富的库和工具(如Pandas、NumPy或TensorFlow)时,结合JSP的动态网页生成能力,可以实现强大的功能。此外,Python的图像处理和自然语言处理模块也可以被JSP调用,以增强网站的交互性和用户体验。
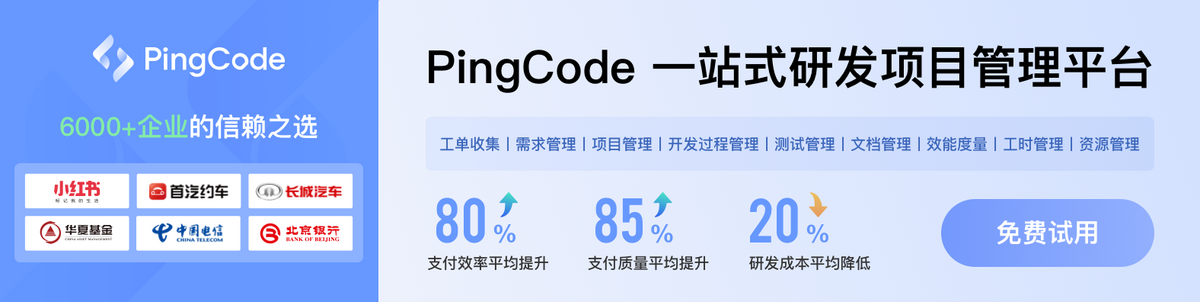