在Python中,记录print
函数的输出内容有多种方法,包括重定向标准输出、使用日志模块、将输出写入文件。这些方法各有优缺点,可以根据具体需求选择适合的方法。下面将详细介绍一种常用的方法:重定向标准输出。
重定向标准输出是通过将sys.stdout
重定向到文件或其他对象,实现对print
输出内容的捕获和记录。这样做的好处是可以在不修改大量代码的情况下,捕获并保存所有print
输出,尤其在调试和记录程序运行状态时非常有用。
一、重定向标准输出
1、使用sys.stdout
重定向
在Python中,sys.stdout
是一个文件对象,默认情况下指向控制台。我们可以将它重定向到其他文件对象来捕获print
输出。以下是具体步骤:
import sys
保存当前标准输出
original_stdout = sys.stdout
打开一个文件用于记录输出
with open('output.txt', 'w') as f:
# 重定向标准输出到文件
sys.stdout = f
print('This will be written to the file.')
print('Another line for the file.')
恢复标准输出到控制台
sys.stdout = original_stdout
print('This will be displayed on the console.')
在这个例子中,print
函数的输出被重定向到output.txt
文件中。通过在with
语句块结束后恢复sys.stdout
,确保后续的print
输出重新回到控制台。
2、重定向到io.StringIO
如果不想将输出写入文件,而是需要在内存中捕获输出,可以使用io.StringIO
:
import sys
import io
创建一个StringIO对象
output = io.StringIO()
重定向标准输出到StringIO对象
sys.stdout = output
print('This will be captured in the StringIO object.')
print('Another captured line.')
获取捕获的内容
captured_output = output.getvalue()
恢复标准输出
sys.stdout = sys.__stdout__
print('Captured:', captured_output)
io.StringIO
对象在内存中模拟文件操作,适合用于短时间捕获和处理输出内容。
二、使用日志模块
1、Python的logging
模块
Python的logging
模块提供了强大的日志功能,可用于记录程序运行时的信息。相比直接使用print
,logging
模块更灵活和可扩展。
import logging
配置日志记录
logging.basicConfig(filename='app.log', level=logging.INFO, format='%(asctime)s - %(message)s')
logging.info('This message will be logged to a file.')
logging.error('This is an error message.')
还可以将日志输出到控制台
console = logging.StreamHandler()
console.setLevel(logging.INFO)
formatter = logging.Formatter('%(name)s - %(levelname)s - %(message)s')
console.setFormatter(formatter)
logging.getLogger('').addHandler(console)
logging.info('This message will be logged both to the file and console.')
使用logging
模块,可以设置不同的日志级别(如DEBUG, INFO, WARNING, ERROR, CRITICAL),并选择将日志输出到文件、控制台或其他目标。
2、自定义日志处理器
如果需要更复杂的日志记录需求,可以通过自定义日志处理器实现。例如,可以创建一个处理器将日志发送到远程服务器,或者记录到数据库中。
import logging
class CustomHandler(logging.Handler):
def emit(self, record):
log_entry = self.format(record)
# 这里可以添加自定义的日志处理逻辑
print('Custom log entry:', log_entry)
使用自定义处理器
logger = logging.getLogger('custom_logger')
logger.setLevel(logging.INFO)
custom_handler = CustomHandler()
formatter = logging.Formatter('%(asctime)s - %(name)s - %(levelname)s - %(message)s')
custom_handler.setFormatter(formatter)
logger.addHandler(custom_handler)
logger.info('This message will be processed by the custom handler.')
通过自定义处理器,日志模块几乎可以满足任何复杂的日志记录需求。
三、将输出写入文件
1、直接将输出写入文件
在很多情况下,简单地将输出写入文件是最直接的方法:
with open('output.txt', 'w') as f:
f.write('This will be written directly to the file.\n')
f.write('Another line to the file.\n')
这种方式适合在需要精确控制输出格式和内容时使用,但不具备日志模块的灵活性。
2、使用contextlib.redirect_stdout
Python的contextlib
模块提供了一个便捷的工具,用于重定向输出流:
import contextlib
import io
f = io.StringIO()
with contextlib.redirect_stdout(f):
print('This will be captured in the StringIO object.')
print('Another captured line.')
获取捕获的内容
captured_output = f.getvalue()
print('Captured:', captured_output)
contextlib.redirect_stdout
简化了输出重定向操作,非常适合在临时需要重定向输出的场景下使用。
四、使用第三方库
1、使用loguru
loguru
是一个第三方库,提供了比标准logging
模块更简单易用的接口:
from loguru import logger
配置日志文件
logger.add('file_{time}.log')
logger.info('This message will be logged to a file.')
logger.error('This is an error message.')
使用装饰器记录函数执行
@logger.catch
def dangerous_function(x, y):
return x / y
dangerous_function(10, 0)
loguru
提供了更直观的API,并且支持异步日志、日志轮换等高级功能,是现代Python项目中日志记录的不错选择。
2、使用structlog
structlog
是一个面向结构化日志的库,适用于需要生成机器可读日志的场景:
import structlog
配置structlog
structlog.configure(
processors=[
structlog.processors.JSONRenderer()
]
)
log = structlog.get_logger()
log.info('message', key='value', another_key='another_value')
structlog
生成的日志是结构化的,便于分析和处理,尤其在分布式系统和云环境中使用优势显著。
通过这些方法,可以在Python中有效地记录print
输出。根据不同的需求,选择合适的方法可以提高程序的可维护性和调试效率。无论是简单的文件记录还是复杂的日志系统,Python都提供了灵活的解决方案。
相关问答FAQs:
如何在Python中将print输出保存到文件中?
在Python中,可以使用重定向的方法将print输出保存到文件中。可以通过将sys.stdout重定向到一个文件对象来实现这一点。以下是一个简单的示例:
import sys
# 打开文件以写入
with open('output.txt', 'w') as f:
# 将stdout重定向到文件
sys.stdout = f
print("这条信息将被写入到文件中")
# 恢复stdout
sys.stdout = sys.__stdout__
print("这条信息将在控制台显示")
这样,文件output.txt中就会包含你在重定向期间的所有print输出。
Python中如何捕获print输出到变量?
如果你希望将print的内容捕获到一个变量中,可以使用io.StringIO模块。通过创建一个StringIO对象并将其作为stdout重定向,可以轻松实现这一点:
import io
import sys
# 创建一个StringIO对象
output = io.StringIO()
# 重定向stdout
sys.stdout = output
print("这条信息将被保存到变量中")
# 获取输出内容
captured_output = output.getvalue()
# 恢复stdout
sys.stdout = sys.__stdout__
print("捕获的内容是:", captured_output)
这种方法特别适合于在测试或调试时捕获输出。
如何在Python中同时打印到控制台和文件?
有时需要同时在控制台和文件中显示输出。可以通过自定义一个类来实现这一点。以下是一个示例:
class DualWriter:
def __init__(self, *writers):
self.writers = writers
def write(self, message):
for writer in self.writers:
writer.write(message)
def flush(self):
for writer in self.writers:
writer.flush()
# 将sys.stdout重定向到控制台和文件
with open('output.txt', 'w') as f:
dual_writer = DualWriter(sys.stdout, f)
sys.stdout = dual_writer
print("这条信息将同时显示在控制台和文件中")
# 恢复stdout
sys.stdout = sys.__stdout__
使用这种方法,您可以同时在多个输出目标中查看打印内容,便于调试和记录。
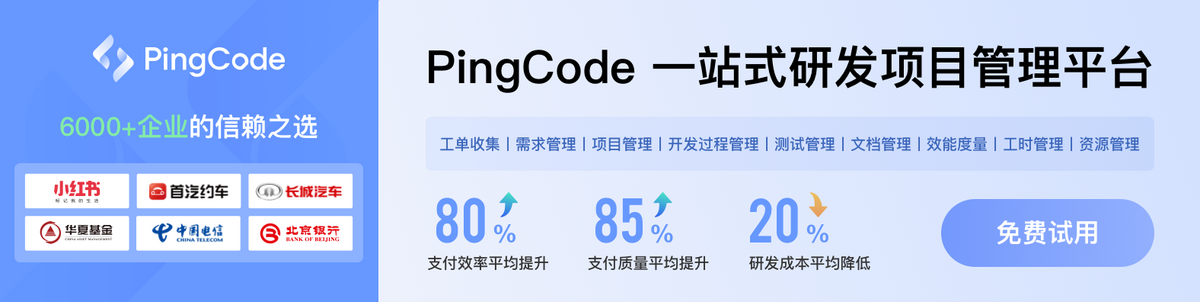