Python采集内容可以通过自动化脚本、API接口、Web框架等多种方式发布。在这里,我们将详细探讨如何利用Python进行内容采集和发布,包括使用不同的技术栈和工具来实现这一过程。Python的强大之处在于其丰富的库和社区支持,使得数据采集与发布变得相对简单和高效。
一、内容采集的基础
内容采集是自动化获取网站数据的过程。Python提供了多种工具来实现这一功能。
- 使用Requests和BeautifulSoup
Requests库用于发送HTTP请求,而BeautifulSoup则用于解析HTML文档。这两个库组合使用,可以轻松地从网页中提取信息。首先,使用Requests库发送请求并获取网页的HTML内容。接下来,使用BeautifulSoup解析HTML,提取所需的数据。
例如,假设我们要从一个新闻网站采集文章标题和链接:
import requests
from bs4 import BeautifulSoup
url = 'http://example.com/news'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
for article in soup.find_all('article'):
title = article.find('h2').get_text()
link = article.find('a')['href']
print(f'Title: {title}, Link: {link}')
- 使用Scrapy框架
Scrapy是一个用于网络爬虫的强大框架,适合于需要大规模数据采集的项目。Scrapy不仅支持数据采集,还提供了数据存储和处理的功能。
首先,安装Scrapy:
pip install scrapy
然后,创建一个Scrapy项目并定义爬虫:
scrapy startproject myproject
cd myproject
scrapy genspider myspider example.com
在爬虫文件中,定义解析逻辑:
import scrapy
class MySpider(scrapy.Spider):
name = 'myspider'
start_urls = ['http://example.com/news']
def parse(self, response):
for article in response.css('article'):
yield {
'title': article.css('h2::text').get(),
'link': article.css('a::attr(href)').get()
}
- 处理动态网页
对于需要JavaScript渲染的动态网页,Selenium是一个常用的选择。Selenium可以模拟浏览器行为,加载完整的网页内容。
首先,安装Selenium和WebDriver:
pip install selenium
然后,使用Selenium加载网页并进行解析:
from selenium import webdriver
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
driver.get('http://example.com/news')
soup = BeautifulSoup(driver.page_source, 'html.parser')
for article in soup.find_all('article'):
title = article.find('h2').get_text()
link = article.find('a')['href']
print(f'Title: {title}, Link: {link}')
driver.quit()
二、数据存储与处理
在采集到数据后,如何有效地存储和处理这些数据是一个关键问题。Python提供了多种方式来实现这一目标。
- 存储到文件系统
最简单的方式是将数据存储到本地文件系统中,例如CSV或JSON文件。
import csv
data = [
{'title': 'Title 1', 'link': 'http://example.com/1'},
{'title': 'Title 2', 'link': 'http://example.com/2'}
]
with open('data.csv', mode='w', newline='') as file:
writer = csv.DictWriter(file, fieldnames=['title', 'link'])
writer.writeheader()
for row in data:
writer.writerow(row)
- 存储到数据库
对于大量数据或需要频繁查询的数据,使用数据库是一个更好的选择。Python支持多种数据库,包括SQLite、MySQL、PostgreSQL等。
以SQLite为例,首先安装SQLite支持库:
pip install sqlite3
然后,创建数据库并插入数据:
import sqlite3
conn = sqlite3.connect('data.db')
c = conn.cursor()
c.execute('CREATE TABLE IF NOT EXISTS articles (title TEXT, link TEXT)')
data = [
('Title 1', 'http://example.com/1'),
('Title 2', 'http://example.com/2')
]
c.executemany('INSERT INTO articles VALUES (?,?)', data)
conn.commit()
conn.close()
三、内容发布的方式
有了采集和存储的数据,接下来就是如何有效地发布这些内容。Python同样提供了多种方式来实现这一目标。
- 使用API接口发布
许多网站和平台提供API接口,允许开发者发布内容。以WordPress为例,其REST API允许外部程序发布文章。
首先,安装Requests库:
pip install requests
然后,使用API接口发布文章:
import requests
import json
url = 'http://example.com/wp-json/wp/v2/posts'
headers = {'Content-Type': 'application/json'}
data = {
'title': 'My Article',
'content': 'This is the article content',
'status': 'publish'
}
response = requests.post(url, headers=headers, data=json.dumps(data), auth=('username', 'password'))
print(response.json())
- 使用Web框架
如果需要发布到自有网站,Python的Web框架如Flask和Django可以帮助构建和部署。
以Flask为例,首先安装Flask:
pip install flask
然后,创建一个简单的Web应用:
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def home():
data = [
{'title': 'Title 1', 'link': 'http://example.com/1'},
{'title': 'Title 2', 'link': 'http://example.com/2'}
]
return render_template('index.html', articles=data)
if __name__ == '__main__':
app.run(debug=True)
在templates/index.html
中,渲染数据:
<!doctype html>
<html>
<head><title>Articles</title></head>
<body>
<h1>Articles</h1>
<ul>
{% for article in articles %}
<li><a href="{{ article.link }}">{{ article.title }}</a></li>
{% endfor %}
</ul>
</body>
</html>
- 自动化发布
对于需要定期发布的内容,Python的调度库如APScheduler可以自动化这一过程。
首先,安装APScheduler:
pip install apscheduler
然后,创建一个定时任务:
from apscheduler.schedulers.blocking import BlockingScheduler
def publish_content():
print("Publishing content...")
scheduler = BlockingScheduler()
scheduler.add_job(publish_content, 'interval', hours=1)
scheduler.start()
四、常见问题与解决方案
在内容采集和发布过程中,可能会遇到各种问题和挑战。
- 反爬虫机制
许多网站会使用反爬虫机制来阻止大量请求。解决方案包括使用随机User-Agent、设置请求间隔、使用代理IP等。
- 数据清洗
采集到的数据可能包含噪声,需要进行清洗和格式化。Python的pandas库提供了强大的数据处理功能。
import pandas as pd
data = pd.read_csv('data.csv')
cleaned_data = data.dropna().drop_duplicates()
- 性能优化
对于大规模数据采集,性能是一个关键问题。可以通过多线程、异步IO等方式提升性能。
import asyncio
import aiohttp
async def fetch(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
return await response.text()
urls = ['http://example.com/page1', 'http://example.com/page2']
loop = asyncio.get_event_loop()
tasks = [fetch(url) for url in urls]
loop.run_until_complete(asyncio.gather(*tasks))
五、总结
Python的灵活性和丰富的生态系统为内容采集和发布提供了强大的支持。通过结合使用多种工具和技术,可以高效地实现自动化内容采集和发布。然而,在实际操作中,需要根据具体需求和场景选择合适的工具和方法,并注意遵循相关法律法规,合理使用数据。无论是初学者还是有经验的开发者,都可以通过不断学习和实践,提升数据采集和发布的能力。
相关问答FAQs:
如何使用Python采集内容并进行发布?
Python提供了多种库和工具,可以方便地进行内容采集。常用的库包括Beautiful Soup、Scrapy和Requests等。采集完成后,可以通过多种方式发布内容,例如将数据存储到数据库中,或者通过API发送到网站上。具体步骤包括数据解析、格式化和发布等,选择合适的库和方法可提升效率。
在进行内容采集时需要注意哪些法律法规?
在进行内容采集时,用户应遵循相关的法律法规,包括但不限于版权法和数据保护法。确保采集的内容不侵犯他人的知识产权,并遵循网站的robots.txt文件中的规则。此外,尊重用户隐私,避免获取个人敏感信息,确保采集行为的合法性。
如何提高内容采集的效率和准确性?
提高内容采集效率可以通过使用多线程或异步编程实现,同时结合使用高效的解析库来加快数据处理速度。此外,合理设计爬虫策略,避免频繁请求同一网页,以减少被封禁的风险。数据清洗和去重也是提高准确性的重要步骤,确保采集到的信息真实有效。
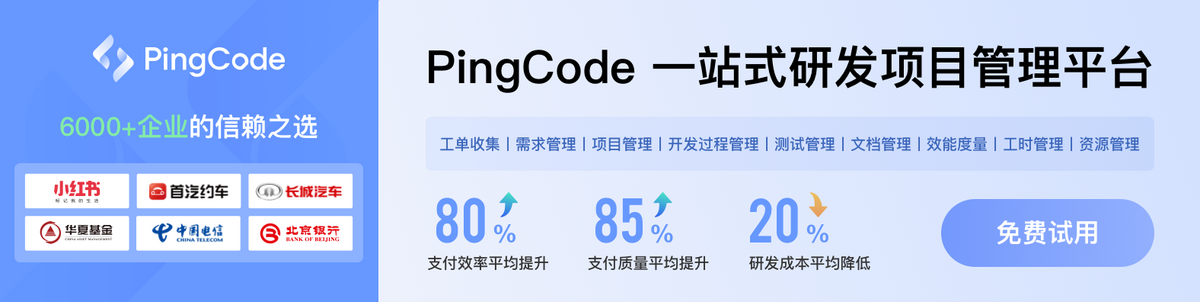