一、Python查找指定字符的方法
在Python中,查找指定字符的方法有多种,常用的包括使用字符串方法find()
、index()
、in
关键字、正则表达式。其中,find()
和index()
方法都用于查找子字符串在字符串中首次出现的位置,但它们在处理找不到字符的情况时有所不同。in
关键字用于检查字符是否存在于字符串中,而正则表达式则提供了更高级的查找功能。使用find()
方法时,如果字符未找到,将返回-1,这种情况常用于需要处理字符不存在的情况。
二、字符串方法find()
和index()
1、find()
方法
find()
方法用于查找子字符串在字符串中第一次出现的索引位置,如果未找到,则返回-1。它的使用较为简单,适合用于需要知道字符位置的场景。
text = "Hello, world!"
position = text.find('o')
print(position) # 输出4
在上述示例中,find()
方法返回字符'o'第一次出现的位置索引4。如果想要从特定位置开始查找,可以提供可选参数start
和end
指定查找范围。
2、index()
方法
index()
方法与find()
类似,但如果未找到子字符串,则会抛出ValueError
异常。这种方式适合用于确保子字符串一定存在的情况。
text = "Hello, world!"
try:
position = text.index('o')
print(position) # 输出4
except ValueError:
print("Character not found")
在上述示例中,使用index()
方法查找字符'o'时,因为字符存在于字符串中,所以输出为4。如果字符不存在,将会抛出异常并被捕获。
三、使用in
关键字进行查找
1、检查字符存在性
使用in
关键字可以快速判断一个字符或子字符串是否存在于字符串中,这种方式不返回位置索引,只用于存在性检查。
text = "Hello, world!"
if 'o' in text:
print("Character found")
else:
print("Character not found")
在上述示例中,'o' in text
用于检查字符'o'是否存在于字符串中,结果为True,因此输出"Character found"。
2、结合in
进行条件判断
in
关键字常用于条件判断中,与其他操作结合使用可以实现更复杂的逻辑。
text = "Hello, world!"
result = "Character found" if 'o' in text else "Character not found"
print(result)
上述示例通过条件表达式简化了代码逻辑,用于实现字符存在性的判断。
四、正则表达式查找
1、正则表达式基础
正则表达式是一种用于模式匹配和查找的强大工具。在Python中,可以使用re
模块中的search()
方法查找指定字符或模式。
import re
text = "Hello, world!"
match = re.search('o', text)
if match:
print(f"Character found at position {match.start()}")
else:
print("Character not found")
在上述示例中,re.search()
方法用于查找字符'o'在字符串中的位置,并返回一个匹配对象。如果匹配成功,可以使用start()
方法获取匹配的起始位置。
2、使用正则表达式匹配模式
正则表达式还可以用于匹配复杂的模式,例如查找数字、特定格式的字符串等。
import re
text = "My phone number is 123-456-7890."
pattern = r'\d{3}-\d{3}-\d{4}'
match = re.search(pattern, text)
if match:
print(f"Phone number found: {match.group()}")
else:
print("Phone number not found")
在上述示例中,正则表达式模式r'\d{3}-\d{3}-\d{4}'
用于匹配电话号码格式,并返回匹配的字符串。
五、使用自定义函数查找
1、编写自定义查找函数
在某些情况下,可能需要编写自定义函数来实现更复杂的查找逻辑。例如,查找字符出现的所有位置索引。
def find_all_occurrences(text, char):
positions = []
index = text.find(char)
while index != -1:
positions.append(index)
index = text.find(char, index + 1)
return positions
text = "Hello, world!"
positions = find_all_occurrences(text, 'o')
print(f"Character 'o' found at positions: {positions}")
在上述示例中,自定义函数find_all_occurrences()
用于查找字符'o'在字符串中出现的所有位置索引,并返回一个列表。
2、优化查找效率
对于长字符串或需要高效查找的场景,可以优化自定义查找函数的效率。例如,使用生成器来减少内存占用。
def find_all_occurrences_generator(text, char):
index = text.find(char)
while index != -1:
yield index
index = text.find(char, index + 1)
text = "Hello, world!"
for position in find_all_occurrences_generator(text, 'o'):
print(f"Character 'o' found at position: {position}")
在上述示例中,使用生成器find_all_occurrences_generator()
实现查找逻辑,逐个返回字符'o'的位置索引。
六、总结
在Python中,查找指定字符的方法有多种选择,具体使用哪种方法取决于需求和上下文。对于简单的存在性检查,可以使用in
关键字;对于需要知道位置的情况,可以使用find()
或index()
方法;而正则表达式则适用于复杂的模式匹配需求。此外,自定义函数提供了灵活的解决方案,适合用于特定场景下的查找逻辑。无论选择哪种方法,都需要根据具体需求进行权衡和选择,以实现最佳的查找效果。
相关问答FAQs:
在Python中,如何判断一个字符串是否包含指定字符?
要判断一个字符串中是否包含特定字符,可以使用in
运算符。例如,如果想检查字符串text
中是否包含字符char
,可以使用如下代码:
if char in text:
print("字符存在")
else:
print("字符不存在")
这种方法简单直观,适合快速检查字符是否存在。
如何在Python中查找指定字符的索引位置?
使用字符串的find()
方法可以轻松找到字符的索引位置。该方法返回字符首次出现的索引,如果字符不在字符串中,则返回-1。示例代码如下:
index = text.find(char)
if index != -1:
print(f"字符的索引位置是:{index}")
else:
print("字符不存在")
这种方式在需要知道字符位置时非常有用。
在Python中,如何查找所有指定字符的索引位置?
如果需要找出字符串中所有指定字符的索引,可以使用列表推导式配合enumerate()
函数来实现。以下是示例代码:
positions = [i for i, c in enumerate(text) if c == char]
print(f"字符的所有索引位置是:{positions}")
这种方法可以有效地获取字符在字符串中的所有出现位置,适合需要全面分析的情况。
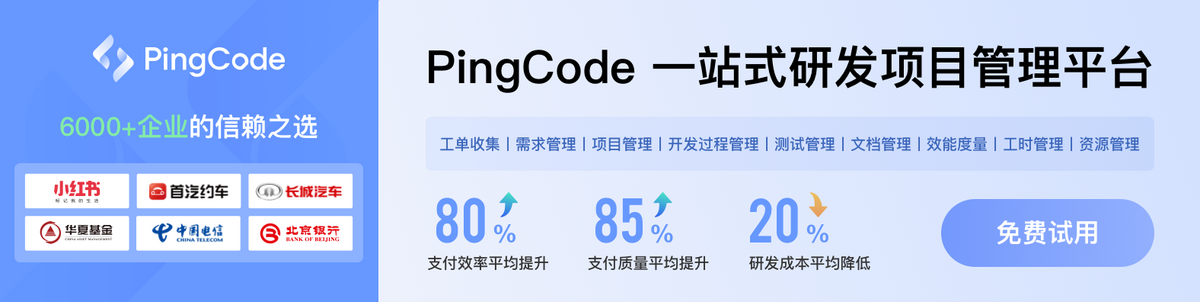