在Python中隐藏TXT文件的方法主要有:修改文件属性、使用加密技术、将文件扩展名更改为其他格式、存储在隐藏目录中。其中,修改文件属性是最常用的方法。通过Python的os模块,可以直接修改文件的属性,使其在操作系统的文件资源管理器中不可见。接下来,我将详细介绍这一方法。
一、修改文件属性
修改文件属性是隐藏TXT文件最简单的方法之一。通过使用Python的os模块,你可以改变文件的属性使其在资源管理器中隐藏。
1. 使用os模块修改文件属性
在Windows系统中,可以利用attrib
命令来修改文件属性。通过Python的os模块,你可以使用os.system()
来执行该命令。例如:
import os
def hide_file(file_path):
os.system(f'attrib +h {file_path}')
file_path = 'example.txt'
hide_file(file_path)
上述代码中,attrib +h
命令将指定文件的隐藏属性设置为True,使其在资源管理器中不可见。相反地,若要取消隐藏,可以使用attrib -h
命令。
2. 跨平台隐藏文件
在Linux和macOS中,隐藏文件的方式与Windows不同。通常,通过在文件名开头加上一个点(.)即可使文件隐藏:
import os
def hide_file(file_path):
# 对于Linux和macOS系统,将文件名前加一个点
if not file_path.startswith('.'):
dir_name = os.path.dirname(file_path)
base_name = os.path.basename(file_path)
hidden_file_path = os.path.join(dir_name, f'.{base_name}')
os.rename(file_path, hidden_file_path)
return hidden_file_path
return file_path
file_path = 'example.txt'
hidden_file_path = hide_file(file_path)
print(f'Hidden file path: {hidden_file_path}')
以上代码会将文件名从example.txt
更改为.example.txt
,从而在Linux和macOS系统中将其隐藏。
二、使用加密技术
加密技术是保护文件内容的一种有效方法。通过加密文件,即使文件被找到,也无法直接读取其中的内容。Python提供了多种加密模块,如cryptography
、PyCrypto
等,可以用于加密文件。
1. 使用cryptography模块加密文件
cryptography是Python中一个强大的加密库,支持多种加密算法。以下是使用cryptography模块加密文件的示例:
from cryptography.fernet import Fernet
def generate_key():
return Fernet.generate_key()
def encrypt_file(file_path, key):
with open(file_path, 'rb') as file:
file_data = file.read()
fernet = Fernet(key)
encrypted_data = fernet.encrypt(file_data)
with open(file_path, 'wb') as file:
file.write(encrypted_data)
def decrypt_file(file_path, key):
with open(file_path, 'rb') as file:
encrypted_data = file.read()
fernet = Fernet(key)
decrypted_data = fernet.decrypt(encrypted_data)
with open(file_path, 'wb') as file:
file.write(decrypted_data)
key = generate_key()
file_path = 'example.txt'
Encrypt the file
encrypt_file(file_path, key)
Decrypt the file
decrypt_file(file_path, key)
上述代码展示了如何生成加密密钥,以及如何加密和解密文件。通过使用Fernet加密算法,文件内容被加密后存储在同一文件中。
三、将文件扩展名更改为其他格式
更改文件扩展名是隐藏文件的一种简单方法。通过更改文件扩展名,可以使文件在通常的文件浏览中不那么显眼,从而增加文件的隐藏性。
1. 更改文件扩展名
可以通过os模块轻松更改文件扩展名:
import os
def change_file_extension(file_path, new_extension):
base = os.path.splitext(file_path)[0]
new_file_path = f'{base}.{new_extension}'
os.rename(file_path, new_file_path)
return new_file_path
file_path = 'example.txt'
new_extension = 'hidden'
new_file_path = change_file_extension(file_path, new_extension)
print(f'File with new extension: {new_file_path}')
以上代码将文件example.txt
的扩展名更改为hidden
,使其不再被识别为普通文本文件。
四、存储在隐藏目录中
将文件存储在隐藏目录中也是一种常见的隐藏方法。在Windows中,可以通过attrib
命令将目录设置为隐藏。在Linux和macOS中,可以在目录名前加一个点来隐藏目录。
1. 在Windows中创建隐藏目录
import os
def create_hidden_directory(dir_path):
os.makedirs(dir_path, exist_ok=True)
os.system(f'attrib +h {dir_path}')
hidden_dir_path = 'hidden_folder'
create_hidden_directory(hidden_dir_path)
上述代码创建一个名为hidden_folder
的目录,并将其设置为隐藏。
2. 在Linux和macOS中创建隐藏目录
import os
def create_hidden_directory(dir_path):
if not dir_path.startswith('.'):
hidden_dir_path = f'.{dir_path}'
os.makedirs(hidden_dir_path, exist_ok=True)
return hidden_dir_path
return dir_path
hidden_dir_path = 'hidden_folder'
hidden_dir_path = create_hidden_directory(hidden_dir_path)
在此示例中,目录名被修改为以点开头,从而在Linux和macOS系统中隐藏。
通过以上几种方法,你可以在Python中实现对TXT文件的隐藏,选择适合你的需求的方法可以有效保护文件的隐私和安全。
相关问答FAQs:
如何在Python中隐藏txt文件?
在Python中,可以通过更改文件的属性来隐藏txt文件。在Windows系统中,可以使用os
模块中的system
方法调用命令行命令来设置文件为隐藏状态。示例代码如下:
import os
file_path = 'your_file.txt'
os.system(f'attrib +h {file_path}')
在Linux系统中,可以通过重命名文件的方式来实现隐藏,通常以点(.)开头的文件会被视为隐藏文件。
隐藏txt文件后,如何恢复显示?
要恢复txt文件的可见性,您可以使用类似的方法。在Windows中,使用attrib -h
命令来去除隐藏属性。示例代码如下:
import os
file_path = 'your_file.txt'
os.system(f'attrib -h {file_path}')
在Linux中,可以将文件重命名为不以点开头的名称,或者使用命令ls -a
来查看所有文件,包括隐藏文件。
在Python中隐藏文件是否会影响文件的内容?
隐藏文件的操作仅仅是更改文件的属性或名称,不会对文件的内容产生任何影响。您仍然可以通过相应的代码或命令访问和编辑文件内容。无论文件是否被隐藏,Python都能够读取和写入这些文件。
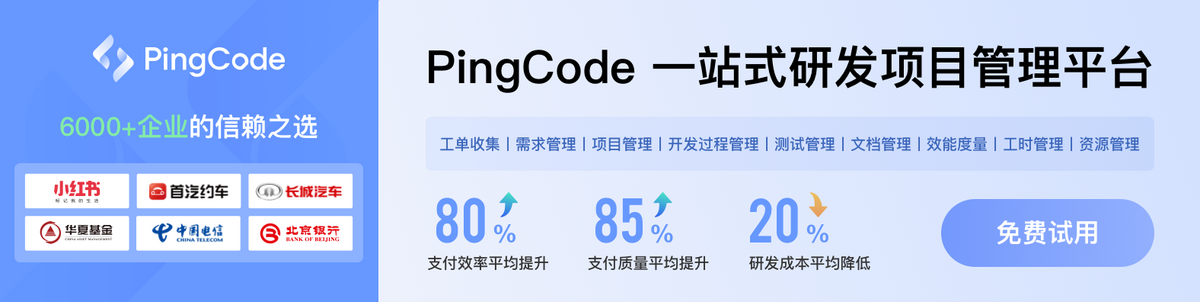