在Python中启动多个线程的方法有:使用threading
模块、使用concurrent.futures.ThreadPoolExecutor
、使用multiprocessing
模块。 其中,使用threading
模块是最常见的方法,因为它提供了一个简单的接口来创建和管理线程。concurrent.futures.ThreadPoolExecutor
可以方便地管理线程池,对于需要大量线程的任务非常有用。multiprocessing
模块提供了启动独立进程的能力,适用于CPU密集型任务,因为Python的全局解释器锁(GIL)限制了多线程的性能。在使用threading
模块时,需要创建一个线程类,继承自threading.Thread
,然后重载run
方法。在concurrent.futures.ThreadPoolExecutor
中,可以使用submit
方法提交多个任务。接下来我们将详细介绍这几种方法。
一、使用threading
模块
threading
模块是Python标准库的一部分,提供了创建和管理线程的功能。要启动多个线程,通常有两种方法:直接创建Thread
对象,或定义一个继承自Thread
类的自定义线程类。
-
直接创建
Thread
对象直接创建
Thread
对象的方式最简单,只需为每个线程创建一个Thread
实例并调用start
方法即可。下面是一个简单的示例,展示如何启动多个线程来执行相同的函数:import threading
def worker(num):
"""线程要执行的任务"""
print(f"Thread {num} is starting")
# 模拟一些工作
import time
time.sleep(1)
print(f"Thread {num} is finished")
threads = []
for i in range(5): # 创建5个线程
thread = threading.Thread(target=worker, args=(i,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join() # 等待所有线程完成
在这个示例中,
worker
函数是每个线程执行的任务。使用threading.Thread
的target
参数指定要执行的函数,args
参数传递给函数的参数。start
方法启动线程,join
方法等待线程完成。 -
定义自定义线程类
定义一个自定义线程类可以使线程的代码更模块化和可重用。继承
threading.Thread
类并重载run
方法是实现自定义线程类的常见方式。import threading
class MyThread(threading.Thread):
def __init__(self, num):
super().__init__()
self.num = num
def run(self):
print(f"Thread {self.num} is starting")
import time
time.sleep(1)
print(f"Thread {self.num} is finished")
threads = []
for i in range(5):
thread = MyThread(i)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
在这个示例中,
MyThread
类继承自threading.Thread
,并重载了run
方法。在run
方法中定义线程要执行的任务。通过创建MyThread
对象并调用start
方法启动线程。
二、使用concurrent.futures.ThreadPoolExecutor
concurrent.futures
模块提供了一个高级接口,用于启动和管理线程池。在某些情况下,使用线程池可以简化多线程编程,尤其是当你需要大量线程来执行类似任务时。
-
使用
ThreadPoolExecutor
ThreadPoolExecutor
类提供了一个上下文管理器,便于启动和管理线程池。使用submit
方法提交任务,并使用result
方法获取任务结果。from concurrent.futures import ThreadPoolExecutor
def worker(num):
print(f"Thread {num} is starting")
import time
time.sleep(1)
print(f"Thread {num} is finished")
return num
with ThreadPoolExecutor(max_workers=5) as executor:
futures = [executor.submit(worker, i) for i in range(5)]
for future in futures:
result = future.result()
print(f"Thread {result} has completed")
在这个示例中,
ThreadPoolExecutor
被用作上下文管理器,自动管理线程池的生命周期。max_workers
参数指定线程池的最大线程数。submit
方法提交任务,返回一个Future
对象,result
方法阻塞直到任务完成并返回结果。
三、使用multiprocessing
模块
虽然multiprocessing
模块主要用于多进程编程,但它也可以用于创建线程。这个模块的优势在于可以绕过Python的GIL,适用于CPU密集型任务。
-
使用
Process
类multiprocessing
模块的Process
类用于启动独立进程,类似于threading.Thread
。from multiprocessing import Process
def worker(num):
print(f"Process {num} is starting")
import time
time.sleep(1)
print(f"Process {num} is finished")
processes = []
for i in range(5):
process = Process(target=worker, args=(i,))
processes.append(process)
process.start()
for process in processes:
process.join()
在这个示例中,每个
Process
对象创建一个新进程,执行worker
函数。start
方法启动进程,join
方法等待进程完成。 -
使用
Pool
类Pool
类提供了一个进程池接口,用于管理多个进程。from multiprocessing import Pool
def worker(num):
print(f"Process {num} is starting")
import time
time.sleep(1)
print(f"Process {num} is finished")
return num
with Pool(5) as pool:
results = pool.map(worker, range(5))
for result in results:
print(f"Process {result} has completed")
在这个示例中,
Pool
对象管理一个进程池,map
方法用于将任务分配给进程池中的进程,类似于内置的map
函数。Pool
类的优势在于能够高效管理进程资源,简化多进程编程。
四、选择合适的方法
在选择启动多个线程的方法时,需要考虑任务的性质和Python的GIL限制。对于I/O密集型任务,使用threading
模块或concurrent.futures.ThreadPoolExecutor
是合适的选择,因为这些任务不会受到GIL的限制。对于CPU密集型任务,multiprocessing
模块更为合适,因为它能够充分利用多核CPU的性能。
此外,线程池和进程池的使用能够简化线程或进程的管理,尤其是在需要启动大量线程或进程时。选择合适的线程或进程池大小需要根据任务的性质和系统资源进行权衡。
总之,Python提供了多种启动和管理线程的方法,能够满足不同类型任务的需求。在实际应用中,应根据具体的任务特性选择最合适的方法,确保程序的高效和稳定运行。
相关问答FAQs:
如何在Python中实现多线程编程?
在Python中实现多线程编程可以使用threading
模块。通过创建Thread
类的实例并调用start()
方法,可以启动多个线程。每个线程可以执行独立的任务,例如处理I/O操作、执行计算等。示例代码如下:
import threading
def worker():
print("线程正在执行任务")
threads = []
for i in range(5): # 启动5个线程
thread = threading.Thread(target=worker)
threads.append(thread)
thread.start()
for thread in threads:
thread.join() # 等待所有线程完成
使用多线程时需要注意哪些问题?
在使用多线程时,有几个关键问题需要关注。首先是线程安全性,多个线程可能会同时访问共享数据,导致数据不一致。可以使用锁(Lock)来解决这个问题。其次,Python的全局解释器锁(GIL)限制了同一时刻只能有一个线程执行Python字节码,这意味着在CPU密集型任务中,多线程的性能提升可能并不明显。最后,合理管理线程生命周期,确保没有线程泄露或阻塞。
Python的多线程和多进程有什么区别?
多线程和多进程都是实现并发的方式,但它们有显著区别。多线程适合I/O密集型任务,由于线程共享同一进程的内存空间,线程间的通信相对简单。多进程则适合CPU密集型任务,因为每个进程都有独立的内存空间,可以充分利用多核CPU的优势。需要使用multiprocessing
模块来实现多进程。选择哪种方式取决于具体的应用场景和需求。
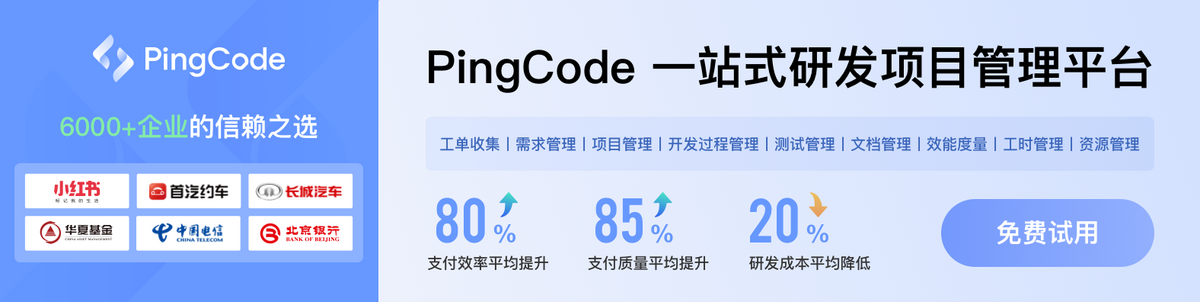