在Python中使用装饰器可以通过定义一个函数并将其应用于另一个函数来实现。装饰器是一种用于修改函数或方法行为的高级工具,它们通常用于日志记录、访问控制、缓存等。通过定义一个装饰器函数并将其应用于目标函数,开发者可以在不改变目标函数代码的情况下添加新的功能。装饰器通常通过在目标函数定义之前加上“@装饰器函数名”来应用。接下来,我们将详细探讨如何定义和使用Python装饰器。
一、装饰器的基本概念
装饰器本质上是一个返回函数的高阶函数。它接受一个函数作为参数,并返回一个增强或修改过的函数。装饰器主要用于在函数执行前后插入额外的代码,或者修改函数的行为,而无需直接修改函数的代码。
装饰器通常用于以下场景:
- 日志记录:在函数调用前后打印日志。
- 性能测试:测量函数执行时间。
- 访问控制和权限管理:在执行函数前检查权限。
- 缓存:缓存函数的返回结果,避免重复计算。
二、定义和应用装饰器
- 基本装饰器:一个基本的装饰器至少需要两个函数:一个外部函数用于接受被装饰的函数,一个内部函数用于执行增强操作。
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
在上述代码中,my_decorator
是一个装饰器,它在say_hello
函数执行的前后打印了一些信息。
- 带参数的装饰器:如果需要在装饰器中传递参数,可以使用一个额外的函数来实现。
def repeat(num_times):
def decorator_repeat(func):
def wrapper(*args, kwargs):
for _ in range(num_times):
result = func(*args, kwargs)
return result
return wrapper
return decorator_repeat
@repeat(num_times=3)
def greet(name):
print(f"Hello {name}")
greet("Alice")
repeat
装饰器可以让目标函数被执行多次,通过传递参数num_times
控制执行次数。
三、使用多个装饰器
Python允许将多个装饰器应用到一个函数上,装饰器的执行顺序是自上而下,而函数的执行顺序是自下而上。
def decorator_one(func):
def wrapper():
print("Decorator one")
func()
return wrapper
def decorator_two(func):
def wrapper():
print("Decorator two")
func()
return wrapper
@decorator_one
@decorator_two
def say_goodbye():
print("Goodbye!")
say_goodbye()
在上述代码中,say_goodbye
函数被两个装饰器修饰,decorator_two
首先执行,然后是decorator_one
。
四、类装饰器
类装饰器通过定义__call__
方法来实现,它们适用于需要在装饰器中维护状态的情况。
class CountCalls:
def __init__(self, func):
self.func = func
self.num_calls = 0
def __call__(self, *args, kwargs):
self.num_calls += 1
print(f"This is call number {self.num_calls}")
return self.func(*args, kwargs)
@CountCalls
def say_hi():
print("Hi!")
say_hi()
say_hi()
在这个例子中,CountCalls
类装饰器用于统计函数被调用的次数。
五、装饰器的实际应用
- 日志装饰器:在实际开发中,装饰器常用于记录函数的输入输出、执行时间等。
import time
def log_execution_time(func):
def wrapper(*args, kwargs):
start_time = time.time()
result = func(*args, kwargs)
end_time = time.time()
print(f"Executing {func.__name__} took {end_time - start_time:.4f} seconds")
return result
return wrapper
@log_execution_time
def compute():
time.sleep(1)
return "Done"
compute()
- 权限检查装饰器:可以用于检查用户权限。
def require_admin(func):
def wrapper(user, *args, kwargs):
if not user.is_admin:
raise PermissionError("You must be an admin to execute this function")
return func(user, *args, kwargs)
return wrapper
class User:
def __init__(self, is_admin):
self.is_admin = is_admin
@require_admin
def delete_user(user, user_to_delete):
print(f"{user_to_delete} has been deleted")
admin_user = User(is_admin=True)
normal_user = User(is_admin=False)
delete_user(admin_user, "Alice")
delete_user(normal_user, "Bob") # This will raise a PermissionError
通过使用装饰器,我们能够在代码中实现强大的功能扩展,而无需重复编写相同的逻辑。这不仅提高了代码的可读性,还增强了代码的可维护性。
相关问答FAQs:
装饰器在Python中有什么作用?
装饰器是一种设计模式,用于在不改变原有函数的情况下,增强或修改函数的功能。它允许程序员在运行时动态地添加新功能到已有对象上。通过使用装饰器,您可以实现功能的重用,如日志记录、权限验证、缓存等,增强代码的可读性和可维护性。
在Python中如何定义一个装饰器?
定义装饰器通常需要创建一个函数,该函数接受另一个函数作为参数,并在内部定义一个包装函数来执行原始函数的功能。包装函数可以在调用原始函数前后添加额外的行为。示例代码如下:
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
使用装饰器时需要注意什么?
使用装饰器时,应注意保持函数的元数据。装饰器可能会隐藏原函数的名称和文档字符串,这可能会影响调试和文档生成。为了解决这个问题,可以使用functools.wraps
来保留原函数的信息。例如:
from functools import wraps
def my_decorator(func):
@wraps(func)
def wrapper():
# 包装逻辑
return func()
return wrapper
这种方式确保了装饰后的函数仍然具有原始函数的名称和文档字符串,更加友好于开发者使用。
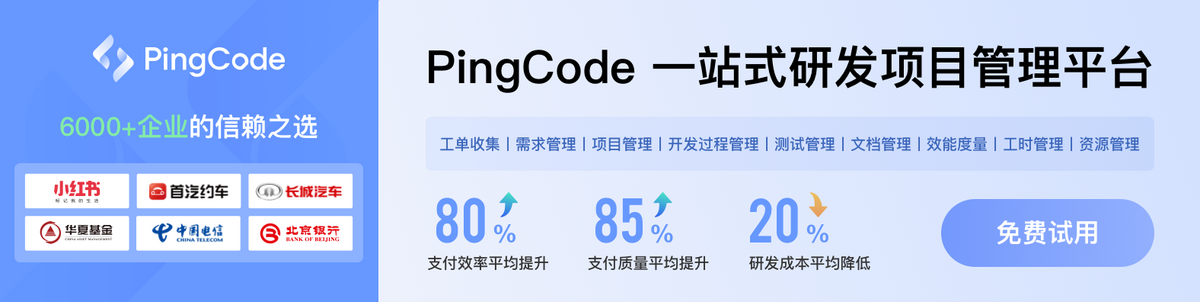