Python中,替换字符串或部分内容的操作可以通过多种方式实现。使用replace()方法、正则表达式(re模块)、结合其他字符串操作方法是常用的手段。下面将详细介绍这些方法中的一种:replace()方法。
replace()方法是Python中字符串对象自带的一个方法,它用于替换字符串中的某些部分。这个方法非常简单,只需要指定要替换的旧子串和新的子串即可。replace()方法的基本语法是str.replace(old, new, count)
,其中old
是要被替换的旧子串,new
是替换后的新子串,count
是一个可选参数,表示最多替换的次数。
举例来说,假设我们有一个字符串text = "Hello, World!"
,我们想把其中的"World"替换为"Python",可以这样使用replace()方法:
text = "Hello, World!"
new_text = text.replace("World", "Python")
print(new_text) # 输出: Hello, Python!
接下来,我们将详细探讨Python中替换字符串的几种方法。
一、REPLACE()方法
1、基本用法
replace()方法是Python字符串对象的一个内建方法,用于替换字符串中的子串。其基本形式为:
str.replace(old, new[, count])
old
:要被替换的子串。new
:用于替换的子串。count
:可选参数,表示最多替换的次数。
例如,替换字符串中的某个单词:
sentence = "The sky is blue."
new_sentence = sentence.replace("blue", "clear")
print(new_sentence) # 输出: The sky is clear.
2、指定替换次数
当你只想替换字符串中出现的前几个匹配项时,可以使用count
参数。例如:
text = "apple, banana, apple, cherry"
result = text.replace("apple", "orange", 1)
print(result) # 输出: orange, banana, apple, cherry
通过指定count=1
,只替换了第一个"apple"为"orange"。
3、替换多个字符
使用replace()方法无法同时替换多个不同的字符或子串,但可以通过链式调用多次replace()来实现:
text = "one apple, two apples, three apples"
new_text = text.replace("one", "1").replace("two", "2").replace("three", "3")
print(new_text) # 输出: 1 apple, 2 apples, 3 apples
二、使用RE模块
Python的re
模块提供了强大的正则表达式支持,可以用来进行复杂的替换操作。
1、re.sub()函数
re.sub()
函数用于在字符串中替换正则表达式匹配的子串,其基本语法为:
re.sub(pattern, repl, string, count=0, flags=0)
pattern
:正则表达式模式。repl
:替换的字符串。string
:要处理的原始字符串。count
:可选参数,表示替换的最大次数,默认是0(表示替换所有匹配)。flags
:正则表达式的匹配标志。
例如,将字符串中的数字替换为"#":
import re
text = "I have 2 apples and 3 bananas."
new_text = re.sub(r'\d+', '#', text)
print(new_text) # 输出: I have # apples and # bananas.
2、使用捕获组
使用捕获组可以在替换中引用匹配的内容:
import re
text = "John Smith"
new_text = re.sub(r'(\w+) (\w+)', r'\2, \1', text)
print(new_text) # 输出: Smith, John
在这个例子中,使用了捕获组(\w+) (\w+)
来匹配名字和姓氏,然后通过\2, \1
来重新排列。
三、结合其他字符串操作方法
1、使用str.translate()
str.translate()
方法与str.maketrans()
结合使用,可以替换单个字符。适合用于字符级别的替换:
text = "hello world"
translation_table = str.maketrans("lo", "12")
new_text = text.translate(translation_table)
print(new_text) # 输出: he112 w2r1d
2、结合split()和join()
在某些情况下,使用split()
和join()
方法可以实现复杂的替换操作:
text = "this is a test"
words = text.split()
new_text = '-'.join(words)
print(new_text) # 输出: this-is-a-test
四、替换的应用场景
1、数据清理
在数据分析和处理过程中,替换操作是数据清理的一部分。例如,替换掉无意义的字符或文本:
data = "N/A, 45, N/A, 78"
clean_data = data.replace("N/A", "0")
print(clean_data) # 输出: 0, 45, 0, 78
2、模板替换
在生成动态内容时,通常需要替换模板中的占位符:
template = "Hello, {name}!"
message = template.format(name="Alice")
print(message) # 输出: Hello, Alice!
通过以上多种方法,Python提供了灵活的字符串替换功能,满足不同场景的需求。根据具体的应用场景选择合适的方法,可以有效地提高代码的效率和可读性。
相关问答FAQs:
如何在Python中进行字符串替换?
在Python中,可以使用str.replace()
方法来替换字符串中的特定子串。这个方法接受两个参数,第一个是要被替换的子串,第二个是用于替换的新子串。可以选择指定替换的次数,如果不指定,则会替换所有出现的子串。例如:
original_string = "Hello, world!"
new_string = original_string.replace("world", "Python")
print(new_string) # 输出: Hello, Python!
Python中是否有其他方法可以替换字符串?
除了str.replace()
方法,Python还支持使用正则表达式进行更复杂的替换操作。可以使用re
模块中的re.sub()
函数,它允许使用模式匹配来替换字符串。以下是一个示例:
import re
original_string = "I have 2 apples and 3 oranges."
new_string = re.sub(r'\d+', 'many', original_string)
print(new_string) # 输出: I have many apples and many oranges.
在Python中如何处理大小写敏感的替换?
Python的字符串替换是大小写敏感的。如果需要进行不区分大小写的替换,可以结合使用re.sub()
和re.IGNORECASE
标志。这样可以确保所有匹配的大小写形式都会被替换。例如:
import re
original_string = "Python is great. python is fun."
new_string = re.sub(r'python', 'Java', original_string, flags=re.IGNORECASE)
print(new_string) # 输出: Java is great. Java is fun.
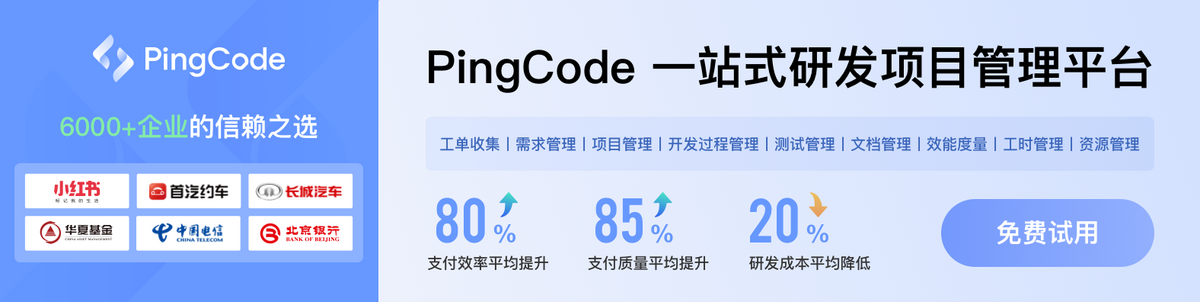