在Python中实现跳转功能,可以通过多种方式来实现,具体包括使用函数、使用类和对象、使用装饰器等方法。最常见的实现方式是通过定义函数,然后在不同的条件下调用不同的函数来实现跳转功能。函数的使用是Python中最基础的代码结构之一,它能够封装一段代码逻辑,并在需要时调用,实现代码复用和逻辑跳转。下面将详细介绍这几种方法,并提供一些代码示例来帮助理解。
一、使用函数实现跳转
函数是Python中最基本的代码封装方式,通过定义函数并在需要的时候调用,可以实现逻辑上的跳转。
1、定义和调用函数
在Python中,函数的定义使用def
关键字。可以通过不同的条件来选择调用不同的函数,从而实现跳转。
def greet():
print("Hello, World!")
def farewell():
print("Goodbye, World!")
def main(condition):
if condition == 'greet':
greet()
elif condition == 'farewell':
farewell()
else:
print("No valid condition provided.")
Example usage
main('greet') # Outputs: Hello, World!
main('farewell') # Outputs: Goodbye, World!
2、使用返回值进行跳转
函数除了可以执行特定操作外,还可以返回值。根据返回值来决定后续调用哪个函数,这也是一种实现跳转的方式。
def get_user_input():
return input("Enter '1' for greeting or '2' for farewell: ")
def process_choice(choice):
if choice == '1':
greet()
elif choice == '2':
farewell()
else:
print("Invalid choice.")
def main():
choice = get_user_input()
process_choice(choice)
Example usage
main()
二、使用类和对象实现跳转
类和对象提供了一种结构化的方式来管理状态和行为,通过定义类,可以将不同的功能封装在方法中,根据需要来调用不同的方法实现跳转。
1、定义类和方法
可以通过定义类,将相关的功能封装为类的方法,并通过创建对象来调用这些方法。
class Greeter:
def greet(self):
print("Hello, World!")
def farewell(self):
print("Goodbye, World!")
def perform_action(self, action):
if action == 'greet':
self.greet()
elif action == 'farewell':
self.farewell()
else:
print("Invalid action.")
Example usage
greeter = Greeter()
greeter.perform_action('greet')
greeter.perform_action('farewell')
2、使用继承和多态
继承和多态是面向对象编程的两个重要概念,通过继承可以创建一系列相关的类,通过多态可以在运行时决定调用哪个方法。
class BaseAction:
def execute(self):
pass
class GreetAction(BaseAction):
def execute(self):
print("Hello, World!")
class FarewellAction(BaseAction):
def execute(self):
print("Goodbye, World!")
def perform_action(action: BaseAction):
action.execute()
Example usage
greet_action = GreetAction()
farewell_action = FarewellAction()
perform_action(greet_action)
perform_action(farewell_action)
三、使用装饰器实现跳转
装饰器是Python中的一种高级特性,它允许在函数的定义和调用之间插入额外的逻辑。可以使用装饰器来改变函数的行为,实现跳转功能。
1、定义装饰器
装饰器本质上是一个函数,它接受一个函数作为参数,并返回一个新的函数。可以利用装饰器在函数调用前后插入逻辑。
def action_decorator(func):
def wrapper(*args, kwargs):
print(f"Executing {func.__name__}...")
return func(*args, kwargs)
return wrapper
@action_decorator
def greet():
print("Hello, World!")
@action_decorator
def farewell():
print("Goodbye, World!")
Example usage
greet()
farewell()
2、条件装饰器
可以根据条件动态地决定是否应用某个装饰器,从而实现跳转功能。
def conditional_decorator(condition):
def decorator(func):
if condition:
def wrapper(*args, kwargs):
print(f"Condition met, executing {func.__name__}...")
return func(*args, kwargs)
return wrapper
else:
return func
return decorator
condition = True
@conditional_decorator(condition)
def greet():
print("Hello, World!")
@conditional_decorator(not condition)
def farewell():
print("Goodbye, World!")
Example usage
greet()
farewell()
通过以上几种方式,可以在Python中实现跳转功能。根据具体的应用场景和需求选择合适的方法,是开发者需要考虑的重要问题。在实际开发中,通常会结合使用多种方法,以实现更灵活和高效的代码结构。
相关问答FAQs:
如何在Python中实现网页跳转?
在Python中,可以使用Flask或Django等Web框架来实现网页跳转。以Flask为例,使用redirect
函数和url_for
方法,可以方便地在路由之间进行跳转。示例代码如下:
from flask import Flask, redirect, url_for
app = Flask(__name__)
@app.route('/old-page')
def old_page():
return redirect(url_for('new_page'))
@app.route('/new-page')
def new_page():
return "Welcome to the new page!"
if __name__ == '__main__':
app.run()
通过访问/old-page
,用户将自动跳转到/new-page
。
Python中的条件跳转是如何实现的?
在Python中,可以使用if
语句来实现条件跳转。根据不同的条件,可以通过函数调用或返回值来引导程序流向不同的部分。例如:
def check_condition(x):
if x > 10:
return "Value is greater than 10"
else:
return "Value is 10 or less"
result = check_condition(15)
print(result) # 输出: Value is greater than 10
这种方式可以根据不同条件进行灵活的跳转。
如何在命令行界面中实现跳转功能?
在命令行界面中,可以通过调用不同的函数或模块来实现跳转。例如,可以创建一个简单的菜单系统,根据用户输入选择不同的功能:
def menu():
print("1. Option 1")
print("2. Option 2")
choice = input("Choose an option: ")
if choice == '1':
option_one()
elif choice == '2':
option_two()
else:
print("Invalid choice")
def option_one():
print("You chose option 1")
def option_two():
print("You chose option 2")
menu()
这种设计允许用户在不同的功能之间进行选择和跳转。
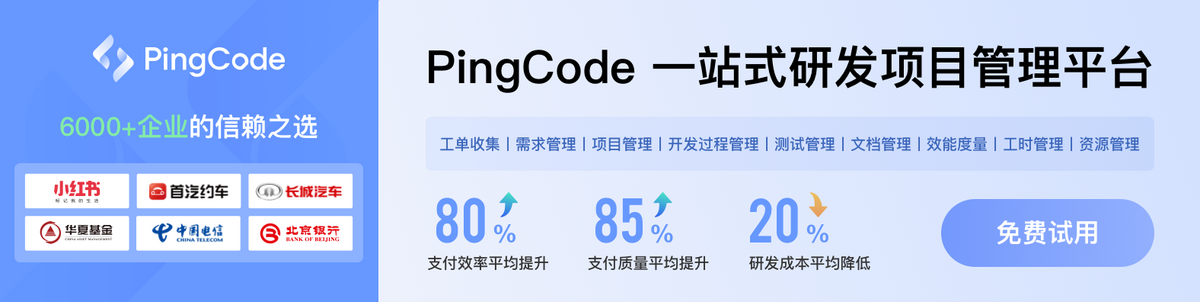