要在Python中修改本地时间,可以使用os
模块调用系统命令、使用subprocess
模块执行系统命令、或者使用第三方库pywin32
(仅限Windows系统)、ctypes
库(跨平台)等方法。最常见的方法是直接通过系统命令进行设置、需要管理员权限。
接下来,我们将详细介绍如何通过多种方法修改本地时间。
一、使用os
模块修改本地时间
在许多操作系统中,可以通过命令行命令来修改系统时间。在Python中,我们可以使用os.system()
来执行这些命令。
1、在Windows系统上
在Windows上,可以使用date
和time
命令来修改日期和时间:
import os
def set_windows_time(date_str, time_str):
os.system(f'date {date_str}')
os.system(f'time {time_str}')
示例:设置日期为2023年12月1日,时间为12:30:00
set_windows_time('12-01-23', '12:30:00')
2、在Linux或macOS系统上
在Linux或macOS系统上,可以使用date
命令来设置时间:
import os
def set_unix_time(date_time_str):
os.system(f'sudo date -s "{date_time_str}"')
示例:设置日期和时间为2023年12月1日12:30:00
set_unix_time('2023-12-01 12:30:00')
注意:在Linux和macOS上,由于需要管理员权限来修改系统时间,因此需要使用sudo
命令,并且运行脚本的用户需要有相应的权限。
二、使用subprocess
模块修改本地时间
subprocess
模块比os.system()
提供了更强大的功能和更好的安全性。我们可以使用subprocess.run()
来执行系统命令。
1、在Windows系统上
import subprocess
def set_windows_time(date_str, time_str):
subprocess.run(['date', date_str], shell=True)
subprocess.run(['time', time_str], shell=True)
示例
set_windows_time('12-01-23', '12:30:00')
2、在Linux或macOS系统上
import subprocess
def set_unix_time(date_time_str):
subprocess.run(['sudo', 'date', '-s', date_time_str], shell=True)
示例
set_unix_time('2023-12-01 12:30:00')
三、使用pywin32
库修改本地时间(仅限Windows)
pywin32
是一个专门用于Windows系统的Python库,可以通过调用Windows API来修改系统时间。
安装pywin32
首先,确保安装了pywin32
库:
pip install pywin32
修改时间
import pywin32.win32api as win32api
import pywin32.win32timezone as win32timezone
from datetime import datetime
def set_windows_time(year, month, day, hour, minute, second):
dt = datetime(year, month, day, hour, minute, second)
win32api.SetSystemTime(dt.year, dt.month, dt.weekday(), dt.day, dt.hour, dt.minute, dt.second, 0)
示例:设置日期和时间为2023年12月1日12:30:00
set_windows_time(2023, 12, 1, 12, 30, 0)
注意:在使用pywin32
修改时间时,需要以管理员权限运行Python脚本。
四、使用ctypes
库修改本地时间(跨平台)
ctypes
是Python的一个外部函数库,可以用来调用C语言编写的动态链接库。通过ctypes
,可以直接调用系统API来修改系统时间。
在Windows系统上
import ctypes
from datetime import datetime
def set_windows_time(year, month, day, hour, minute, second):
class SYSTEMTIME(ctypes.Structure):
_fields_ = [("wYear", ctypes.c_ushort),
("wMonth", ctypes.c_ushort),
("wDayOfWeek", ctypes.c_ushort),
("wDay", ctypes.c_ushort),
("wHour", ctypes.c_ushort),
("wMinute", ctypes.c_ushort),
("wSecond", ctypes.c_ushort),
("wMilliseconds", ctypes.c_ushort)]
system_time = SYSTEMTIME(year, month, 0, day, hour, minute, second, 0)
ctypes.windll.kernel32.SetSystemTime(ctypes.byref(system_time))
示例
set_windows_time(2023, 12, 1, 12, 30, 0)
在Linux系统上
import ctypes
import ctypes.util
from datetime import datetime
class timespec(ctypes.Structure):
_fields_ = [("tv_sec", ctypes.c_long),
("tv_nsec", ctypes.c_long)]
def set_unix_time(year, month, day, hour, minute, second):
libc = ctypes.CDLL(ctypes.util.find_library("c"))
tm = datetime(year, month, day, hour, minute, second)
ts = timespec(int(tm.timestamp()), 0)
libc.clock_settime(0, ctypes.byref(ts))
示例
set_unix_time(2023, 12, 1, 12, 30, 0)
注意:在Linux系统上,使用ctypes
修改时间时,同样需要管理员权限。
五、注意事项
-
权限要求:修改系统时间通常需要管理员权限。确保以管理员身份运行脚本。
-
时间同步:许多系统会自动与网络时间协议(NTP)服务器同步时间,手动修改时间可能会在下次同步时被覆盖。
-
系统影响:修改系统时间会影响到操作系统和应用程序的时间戳、计划任务等功能。在生产环境中谨慎操作。
-
调试与测试:在测试环境中进行充分的测试,确保修改时间不会对系统产生负面影响。
通过以上多种方法,Python可以实现修改本地时间的功能。根据具体使用环境和需求,选择合适的方法来进行操作。
相关问答FAQs:
如何在Python中获取本地时间?
可以使用datetime
模块来获取和显示本地时间。以下是一个简单的示例代码:
from datetime import datetime
local_time = datetime.now()
print("当前本地时间:", local_time)
这段代码将输出当前的本地时间。
Python中是否可以直接修改系统时间?
Python本身并没有直接修改系统时间的权限。不过,可以通过调用系统命令来实现这一功能。例如,在Windows系统上,可以使用os
模块结合命令行工具来设置时间,但需要管理员权限。在Linux系统中,可以使用sudo
命令来修改时间。
如何在Python中格式化时间输出?
使用strftime
方法可以很方便地格式化时间输出。比如,想要将时间格式化为“年-月-日 时:分:秒”的形式,可以这样写:
formatted_time = local_time.strftime("%Y-%m-%d %H:%M:%S")
print("格式化后的时间:", formatted_time)
通过这种方式,可以根据需要自定义时间的输出格式。
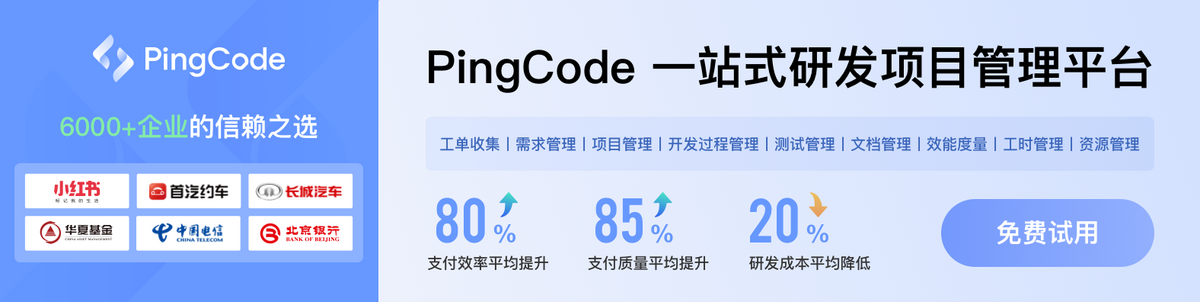