一、直接使用Python枚举所有磁盘的方法
在Python中枚举所有磁盘的方法主要有以下几种:使用os
库、使用psutil
库、使用platform
库。其中,最常用的方法是利用psutil
库,因为它提供了更多的系统和进程信息。通过psutil.disk_partitions()
函数,我们可以获取系统上所有挂载的磁盘分区并返回详细的信息。
psutil
是一个跨平台库,支持在Windows、Linux和macOS上使用。通过它,我们可以轻松获取磁盘的设备名称、挂载点、文件系统类型等信息。下面将详细介绍如何使用psutil
库来枚举所有磁盘。
import psutil
def list_disks():
partitions = psutil.disk_partitions()
for partition in partitions:
print(f"Device: {partition.device}, Mountpoint: {partition.mountpoint}, Fstype: {partition.fstype}")
list_disks()
上述代码通过调用psutil.disk_partitions()
函数获取所有磁盘分区的信息,然后遍历每个分区,输出设备名称、挂载点和文件系统类型。
二、通过os
库枚举磁盘
os
库提供的接口可以用于获取磁盘信息,但在跨平台支持方面不如psutil
库全面。在Windows系统中,我们可以使用os.popen()
来调用Windows命令行命令wmic logicaldisk get name
来列出磁盘。
import os
def list_disks_os():
if os.name == 'nt': # For Windows
disks = os.popen('wmic logicaldisk get name').read().strip().split('\n')[1:]
disks = [disk.strip() for disk in disks if disk.strip()]
for disk in disks:
print(f"Disk: {disk}")
else:
print("This method is specific to Windows.")
list_disks_os()
在Linux或macOS上,os
库不提供直接的方法来列出磁盘,因此需要结合其他命令,例如df
命令来获取挂载的磁盘信息。
三、使用platform
库结合系统命令
platform
库可以帮助我们识别操作系统类型,并结合相应的系统命令获取磁盘信息。这是一种更灵活的方式,但需要对不同操作系统的命令有一定的了解。
import platform
import os
def list_disks_platform():
os_type = platform.system()
if os_type == "Windows":
disks = os.popen('wmic logicaldisk get name').read().strip().split('\n')[1:]
disks = [disk.strip() for disk in disks if disk.strip()]
for disk in disks:
print(f"Disk: {disk}")
elif os_type == "Linux" or os_type == "Darwin": # Darwin is macOS
disks = os.popen('df -h').read().strip().split('\n')[1:]
for disk in disks:
columns = disk.split()
print(f"Device: {columns[0]}, Mounted on: {columns[5]}")
else:
print("Unsupported OS")
list_disks_platform()
四、获取磁盘的更多信息
除了获取磁盘的基本信息外,我们还可以使用psutil
库获取磁盘的使用情况和IO信息。这对于需要监控系统资源的应用程序非常有用。
import psutil
def get_disk_usage():
partitions = psutil.disk_partitions()
for partition in partitions:
try:
usage = psutil.disk_usage(partition.mountpoint)
print(f"Device: {partition.device}")
print(f" Total: {usage.total} bytes")
print(f" Used: {usage.used} bytes")
print(f" Free: {usage.free} bytes")
print(f" Percent Used: {usage.percent}%")
except PermissionError:
# This can happen on some systems if we don't have permission to access the disk
print(f"Permission denied for {partition.device}")
get_disk_usage()
五、使用psutil
获取磁盘IO信息
磁盘的IO信息包括读写操作的次数和字节数,这在性能调优和系统监控中非常重要。psutil.disk_io_counters()
函数可以帮助我们获取这些信息。
import psutil
def get_disk_io():
io_counters = psutil.disk_io_counters(perdisk=True)
for device, counters in io_counters.items():
print(f"Device: {device}")
print(f" Reads: {counters.read_count} times, {counters.read_bytes} bytes")
print(f" Writes: {counters.write_count} times, {counters.write_bytes} bytes")
get_disk_io()
总结来说,Python提供了多种方法来枚举和获取磁盘信息,其中psutil
库是最为全面和跨平台的选择。通过结合不同的库和系统命令,我们可以灵活地获取各种磁盘相关的信息,以满足不同的需求。
相关问答FAQs:
如何使用Python识别系统中的所有磁盘?
使用Python识别系统中的所有磁盘可以通过多个库实现,例如os
和psutil
。psutil
库是一个强大的工具,可以轻松获取系统的信息。可以安装psutil
库并使用它的disk_partitions()
函数来列出所有可用的磁盘和分区信息。
在Python中如何获取磁盘的详细信息?
获取磁盘的详细信息可以利用psutil
库中的disk_usage()
函数。通过传入特定的分区路径,可以获取该分区的使用情况,包括总空间、已用空间和可用空间等。此外,disk_io_counters()
函数可以提供磁盘的读写统计信息,帮助你了解磁盘的性能。
是否可以使用Python自动挂载新磁盘?
虽然Python本身没有直接的挂载磁盘功能,但可以通过调用系统命令实现此功能。使用subprocess
模块,可以在Python脚本中执行Linux或Windows的挂载命令。不过,执行此操作需要有适当的权限,确保在执行前了解相关的系统命令和参数,以避免潜在的系统问题。
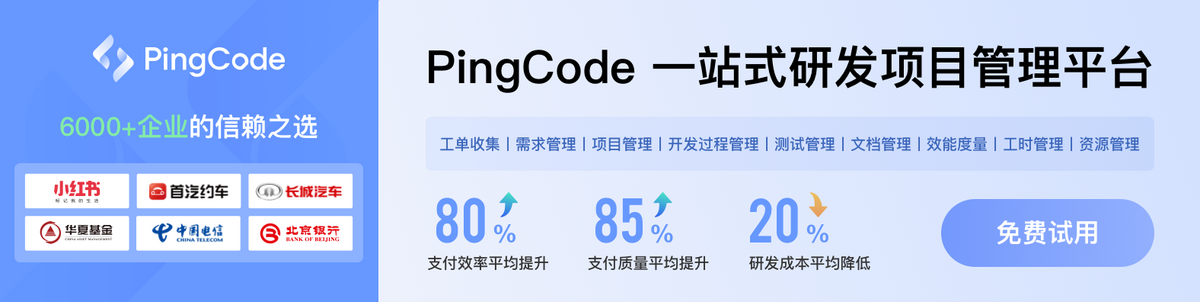