在Python中判断文件是否更新,可以通过检查文件的修改时间、使用文件的哈希值、监听文件系统事件来实现。其中,检查文件的修改时间是最常用的方法,适合大多数简单的更新检测需求。通过使用Python的os.path
模块,我们可以很容易地获取文件的最后修改时间并与之前的记录进行比较,以确定文件是否已更新。
通过文件的修改时间来判断文件是否更新非常直观且高效。每个文件系统都维护一个文件的元数据,其中就包括最后修改时间。Python提供了os.path.getmtime()
函数,可以方便地获取文件的修改时间。通过定期检查文件的修改时间并与之前记录的时间进行比较,可以轻松判断文件是否发生了更新。例如,如果我们在某个时间点记录了文件的修改时间,并在之后的某个时刻再次获取修改时间,如果两者不同,则可以认为文件已经更新。
接下来,我们将详细探讨如何在Python中实现文件更新的判断。
一、通过修改时间判断文件更新
通过文件的修改时间来判断文件是否更新是最简单的方法。我们可以使用Python的标准库os
和time
来实现这一功能。
- 获取文件的修改时间
要获取文件的修改时间,我们可以使用os.path.getmtime()
函数,该函数返回文件的最后修改时间,以秒为单位的浮点数表示。
import os
def get_file_modification_time(file_path):
return os.path.getmtime(file_path)
- 判断文件是否更新
通过比较文件的当前修改时间和之前记录的修改时间,可以判断文件是否更新。
import time
def is_file_updated(file_path, last_mod_time):
current_mod_time = get_file_modification_time(file_path)
return current_mod_time != last_mod_time
- 示例代码
结合上述函数,我们可以编写一个简单的示例程序来监测文件的更新状态。
import time
import os
def get_file_modification_time(file_path):
return os.path.getmtime(file_path)
def is_file_updated(file_path, last_mod_time):
current_mod_time = get_file_modification_time(file_path)
return current_mod_time != last_mod_time
file_path = 'example.txt'
last_mod_time = get_file_modification_time(file_path)
while True:
if is_file_updated(file_path, last_mod_time):
print(f"{file_path} has been updated.")
last_mod_time = get_file_modification_time(file_path)
time.sleep(5)
二、通过文件哈希值判断文件更新
通过文件的哈希值来判断文件是否更新是一种更为精确的方法。这种方法适用于需要检测文件内容变化的场景。
- 计算文件的哈希值
我们可以使用Python的hashlib
库来计算文件的哈希值。例如,使用SHA-256算法。
import hashlib
def calculate_file_hash(file_path):
sha256 = hashlib.sha256()
with open(file_path, 'rb') as f:
while chunk := f.read(8192):
sha256.update(chunk)
return sha256.hexdigest()
- 判断文件是否更新
通过比较文件的当前哈希值和之前记录的哈希值,可以判断文件是否更新。
def is_file_content_updated(file_path, last_hash):
current_hash = calculate_file_hash(file_path)
return current_hash != last_hash
- 示例代码
结合上述函数,我们可以编写一个简单的示例程序来监测文件的更新状态。
import time
import hashlib
def calculate_file_hash(file_path):
sha256 = hashlib.sha256()
with open(file_path, 'rb') as f:
while chunk := f.read(8192):
sha256.update(chunk)
return sha256.hexdigest()
def is_file_content_updated(file_path, last_hash):
current_hash = calculate_file_hash(file_path)
return current_hash != last_hash
file_path = 'example.txt'
last_hash = calculate_file_hash(file_path)
while True:
if is_file_content_updated(file_path, last_hash):
print(f"{file_path} content has been updated.")
last_hash = calculate_file_hash(file_path)
time.sleep(5)
三、通过监听文件系统事件判断文件更新
使用文件系统事件监听是一种实时、高效的文件更新检测方法。Python可以通过第三方库watchdog
实现这一功能。
- 安装
watchdog
库
首先,通过pip安装watchdog
库。
pip install watchdog
- 使用
watchdog
监听文件更新
通过继承watchdog.events.FileSystemEventHandler
,可以实现自定义的事件处理逻辑。
from watchdog.observers import Observer
from watchdog.events import FileSystemEventHandler
import time
class FileUpdateHandler(FileSystemEventHandler):
def __init__(self, file_path):
self.file_path = file_path
def on_modified(self, event):
if event.src_path == self.file_path:
print(f"{self.file_path} has been updated.")
file_path = 'example.txt'
event_handler = FileUpdateHandler(file_path)
observer = Observer()
observer.schedule(event_handler, path='.', recursive=False)
observer.start()
try:
while True:
time.sleep(1)
except KeyboardInterrupt:
observer.stop()
observer.join()
- 示例代码
上述代码演示了如何使用watchdog
库监听文件更新事件。程序会在文件被修改时输出提示信息。
四、总结
在Python中判断文件更新的方法有多种,包括通过文件修改时间、文件哈希值、监听文件系统事件等。每种方法都有其适用的场景和优缺点。通过文件修改时间判断文件更新最简单,但可能无法检测到内容不变的情况下的更新。通过文件哈希值判断文件更新更精确,但计算哈希值会增加一定的开销。使用文件系统事件监听则提供了一种实时检测文件更新的高效方法,适用于需要即时响应文件变化的场景。
无论选择哪种方法,都应根据具体的应用场景和需求来权衡优缺点,从而选择最合适的解决方案。
相关问答FAQs:
如何使用Python检测文件的修改时间?
可以使用os.path.getmtime()
函数获取文件的最后修改时间。通过比较两次获取的时间戳,可以判断文件是否被更新。例如,先记录文件的修改时间,再定期检查该时间戳是否发生变化。
Python可以监控文件变化吗?
是的,Python可以通过使用watchdog
库来监控文件的变化。该库允许你创建一个观察者,实时检测文件或目录的变化,包括创建、修改和删除操作。
如何在Python中判断文件的内容是否发生变化?
一种常见的方法是计算文件的哈希值。使用hashlib
库中的md5
或sha256
函数,可以生成文件内容的唯一标识符。通过比较文件的哈希值,可以判断文件内容是否发生了变化。
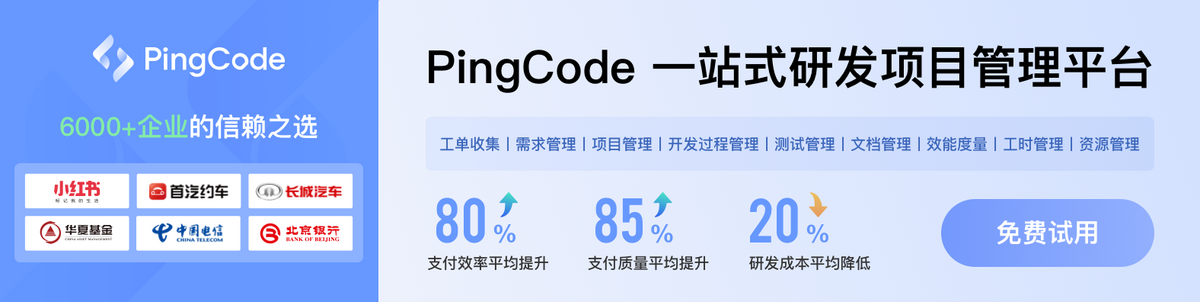