使用Python检测网络可以通过多种方式来实现,包括、ping命令、socket编程、使用第三方库(如scapy、requests)来检测网络连接的稳定性和性能。使用ping命令可以快速判断目标主机的可达性,socket编程可以通过建立TCP连接来检测网络服务的可用性,而第三方库可以提供更丰富的网络检测功能,如抓包、发送HTTP请求等。本文将详细介绍这些方法的实现细节。
一、PING命令检测
Ping命令是网络检测中最常用的工具之一,通过发送ICMP请求来判断目标主机是否在线。
- 使用os.system调用ping命令
import os
def ping_host(host):
response = os.system(f"ping -c 1 {host}")
if response == 0:
print(f"{host} is reachable.")
else:
print(f"{host} is not reachable.")
ping_host("google.com")
在上面的代码中,我们使用Python的os
模块调用系统的ping
命令。通过检测返回值来判断目标主机是否可达。
- 使用subprocess模块获取更详细的信息
import subprocess
def ping_host_subprocess(host):
try:
output = subprocess.check_output(["ping", "-c", "1", host])
print(output.decode())
except subprocess.CalledProcessError:
print(f"{host} is not reachable.")
ping_host_subprocess("google.com")
通过subprocess
模块,我们可以获取ping
命令的输出信息,这对于分析网络延迟和丢包率等详细信息非常有用。
二、SOCKET编程检测
Socket编程是网络编程的基础,通过创建Socket连接,可以检测网络服务的可用性。
- 检测TCP服务的可用性
import socket
def check_tcp_service(host, port):
try:
with socket.create_connection((host, port), timeout=5) as s:
print(f"Connection to {host}:{port} successful.")
except (socket.timeout, socket.error) as e:
print(f"Connection to {host}:{port} failed: {e}")
check_tcp_service("google.com", 80)
这段代码尝试连接目标主机的指定端口,如果连接成功,说明该服务可用。
- 使用UDP检测
UDP是一种无连接协议,我们可以使用它来检测网络的连通性。
def check_udp_service(host, port):
try:
with socket.socket(socket.AF_INET, socket.SOCK_DGRAM) as s:
s.settimeout(5)
s.sendto(b"", (host, port))
print(f"UDP packet sent to {host}:{port}.")
except (socket.timeout, socket.error) as e:
print(f"Failed to send UDP packet to {host}:{port}: {e}")
check_udp_service("google.com", 80)
需要注意的是,UDP检测不会像TCP那样得到明确的连接成功或失败的反馈。
三、使用第三方库
Python有许多强大的第三方库可以用于网络检测,以下是一些常用的库。
- Scapy库
Scapy是一个强大的网络工具库,能够进行抓包、构建和发送网络包等操作。
from scapy.all import ICMP, IP, sr1
def scapy_ping(host):
pkt = IP(dst=host)/ICMP()
response = sr1(pkt, timeout=2, verbose=0)
if response:
print(f"{host} is reachable.")
else:
print(f"{host} is not reachable.")
scapy_ping("google.com")
使用Scapy,我们可以更灵活地构建网络包,适用于高级网络检测。
- Requests库
Requests库主要用于HTTP请求,但也可以用于检测网络的连通性。
import requests
def check_http_service(url):
try:
response = requests.get(url, timeout=5)
if response.status_code == 200:
print(f"HTTP service at {url} is reachable.")
else:
print(f"HTTP service at {url} returned status code {response.status_code}.")
except requests.RequestException as e:
print(f"Failed to reach HTTP service at {url}: {e}")
check_http_service("https://www.google.com")
Requests库的优势在于其简单易用,适合快速检测HTTP服务的可用性。
四、网络性能检测
网络性能检测不仅仅是判断网络是否连通,还包括检测网络延迟、带宽等性能指标。
- 使用ping检测网络延迟
通过前面的ping命令,我们可以获取网络延迟信息。
- 使用speedtest库检测带宽
Speedtest是一个用于检测网络带宽的工具,Python的speedtest-cli
库可以方便地调用这个工具。
import speedtest
def check_bandwidth():
st = speedtest.Speedtest()
st.download()
st.upload()
print(f"Download speed: {st.results.download / 1_000_000:.2f} Mbps")
print(f"Upload speed: {st.results.upload / 1_000_000:.2f} Mbps")
check_bandwidth()
通过speedtest库,我们可以轻松获取网络的上下行带宽信息。
五、故障排除和日志记录
在网络检测过程中,故障排除和日志记录是非常重要的。
- 日志记录
import logging
logging.basicConfig(filename='network_detection.log', level=logging.INFO)
def log_ping_result(host, result):
logging.info(f"Ping {host}: {result}")
log_ping_result("google.com", "reachable")
通过日志记录,我们可以追踪网络检测的历史记录,帮助分析网络问题。
- 故障排除
在检测网络问题时,可以从以下几个方面着手:检查硬件连接、重启路由器、检查防火墙配置、使用多种工具进行交叉验证等。
综上所述,使用Python进行网络检测是一个非常灵活的过程,可以根据具体需求选择不同的方法和工具。通过合理地使用这些工具,我们可以有效地检测和分析网络的连通性和性能问题。
相关问答FAQs:
如何使用Python检测网络连接的状态?
可以使用Python的ping
命令或socket
库来检测网络连接的状态。通过发送ICMP请求或尝试与特定服务器建立TCP连接,可以判断网络是否正常。例如,利用subprocess
模块调用系统的ping命令,或者使用socket
库的create_connection
方法进行端口检测,都是有效的方法。
有哪些Python库可以帮助进行网络检测?
常用的Python库包括ping3
、scapy
和requests
。ping3
能够轻松实现ICMP ping操作,scapy
则提供了更复杂的网络数据包处理功能,而requests
库适合于HTTP请求的网络状态检测。选择合适的库可以根据你的具体需求和使用场景而定。
如何处理Python网络检测中的异常情况?
在进行网络检测时,可能会遇到各种异常情况,比如网络超时、无法连接等。可以通过try-except
语句捕获这些异常,并提供相应的错误处理机制。例如,可以在连接失败时重试几次,或者记录错误信息以便后续分析,确保程序的稳定性与可靠性。
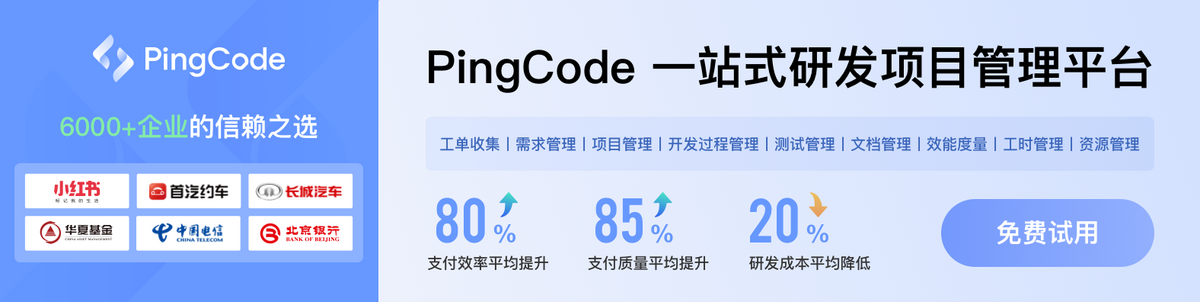