一、验证代理IP的方式
在Python中验证代理IP的有效性,可以通过发送请求、捕获异常、检查响应时间等方式来实现。首先,发送请求是最常用的方法,通过向某个网站发送请求来验证代理IP是否有效。捕获异常可以帮助我们了解代理IP是否被拒绝或无法连接。检查响应时间则可以评估代理IP的速度和稳定性。接下来,我们将详细介绍如何通过发送请求来验证代理IP的有效性。
发送请求是验证代理IP的核心方法之一。在Python中,我们可以使用requests
库来实现这一功能。通过设置代理参数,我们可以向特定的URL发送请求,并检查响应状态码。如果状态码为200,则说明代理IP有效;否则,代理IP可能无效或者被目标服务器拒绝。
import requests
def check_proxy(proxy):
try:
response = requests.get('http://httpbin.org/ip', proxies={'http': proxy, 'https': proxy}, timeout=5)
if response.status_code == 200:
print(f"Proxy {proxy} is valid.")
else:
print(f"Proxy {proxy} is invalid.")
except requests.exceptions.RequestException as e:
print(f"Proxy {proxy} failed. Error: {e}")
Example usage
check_proxy('http://123.123.123.123:8080')
二、捕获异常
在验证代理IP时,捕获异常是另一个重要的步骤。通过捕获异常,我们可以识别由于网络连接错误、代理IP被拒绝或超时等原因导致的请求失败。这样可以更好地了解代理IP的状态,并在必要时更换其他代理。
在requests
库中,我们可以使用try-except
结构来捕获异常。常见的异常包括requests.exceptions.ConnectionError
、requests.exceptions.Timeout
和requests.exceptions.HTTPError
。通过捕获这些异常,我们可以根据不同的情况采取相应的措施。
def check_proxy_with_exception_handling(proxy):
try:
response = requests.get('http://httpbin.org/ip', proxies={'http': proxy, 'https': proxy}, timeout=5)
if response.status_code == 200:
print(f"Proxy {proxy} is valid.")
else:
print(f"Proxy {proxy} is invalid.")
except requests.exceptions.ConnectionError:
print(f"Proxy {proxy} failed. Connection error.")
except requests.exceptions.Timeout:
print(f"Proxy {proxy} failed. Timeout error.")
except requests.exceptions.RequestException as e:
print(f"Proxy {proxy} failed. Other error: {e}")
Example usage
check_proxy_with_exception_handling('http://123.123.123.123:8080')
三、检查响应时间
除了验证代理IP的有效性外,检查响应时间也是一个重要的考虑因素。响应时间能够反映代理IP的速度和稳定性。在某些情况下,代理IP虽然有效,但响应时间过长,可能会影响用户体验。因此,在选择代理IP时,我们应该优先选择那些响应时间较短的。
在Python中,我们可以通过记录请求开始和结束的时间来计算响应时间。使用time
库可以方便地实现这一功能。通过比较多个代理IP的响应时间,我们可以选择出速度最快的代理。
import time
def check_proxy_with_response_time(proxy):
try:
start_time = time.time()
response = requests.get('http://httpbin.org/ip', proxies={'http': proxy, 'https': proxy}, timeout=5)
end_time = time.time()
if response.status_code == 200:
print(f"Proxy {proxy} is valid. Response time: {end_time - start_time:.2f} seconds")
else:
print(f"Proxy {proxy} is invalid.")
except requests.exceptions.RequestException as e:
print(f"Proxy {proxy} failed. Error: {e}")
Example usage
check_proxy_with_response_time('http://123.123.123.123:8080')
四、批量验证代理IP
在实际应用中,我们通常需要验证多个代理IP的有效性。为了提高效率,我们可以编写一个批量验证代理IP的函数。通过遍历代理IP列表,对每个代理IP进行验证,并将结果保存到一个列表中。最终,我们可以输出所有有效的代理IP。
在Python中,编写批量验证代理IP的函数可以使用循环结构。我们可以定义一个函数,接受一个代理IP列表作为参数,并返回一个有效代理IP的列表。通过遍历代理IP列表,对每个代理IP调用验证函数,并将有效的代理IP添加到结果列表中。
def batch_check_proxies(proxies):
valid_proxies = []
for proxy in proxies:
try:
response = requests.get('http://httpbin.org/ip', proxies={'http': proxy, 'https': proxy}, timeout=5)
if response.status_code == 200:
valid_proxies.append(proxy)
except requests.exceptions.RequestException:
continue
return valid_proxies
Example usage
proxies = [
'http://123.123.123.123:8080',
'http://111.111.111.111:8080',
'http://222.222.222.222:8080'
]
valid_proxies = batch_check_proxies(proxies)
print("Valid proxies:", valid_proxies)
五、使用第三方代理验证服务
有时候,为了提高代理验证的准确性和效率,我们可以使用第三方代理验证服务。这些服务通常提供API接口,允许用户提交代理IP进行验证,并返回验证结果。通过使用这些服务,我们可以减少本地资源的消耗,并获得更准确的验证结果。
在Python中,使用第三方代理验证服务通常需要通过发送HTTP请求与服务进行交互。我们可以使用requests
库来发送请求,并根据服务的API文档解析响应数据。使用第三方服务时,需要注意服务的使用限制和费用。
import requests
def check_proxy_with_service(proxy, service_url):
try:
response = requests.get(service_url, params={'proxy': proxy})
if response.status_code == 200 and response.json().get('valid'):
print(f"Proxy {proxy} is valid according to the service.")
else:
print(f"Proxy {proxy} is invalid according to the service.")
except requests.exceptions.RequestException as e:
print(f"Failed to check proxy {proxy} with service. Error: {e}")
Example usage
service_url = 'http://example.com/proxy-check'
check_proxy_with_service('http://123.123.123.123:8080', service_url)
六、代理IP的选择和维护
在验证代理IP之后,我们还需要考虑如何选择和维护代理IP。选择好的代理IP可以提高访问速度和稳定性,而维护则可以确保代理IP的长期有效性。
选择代理IP时,我们可以根据地理位置、响应时间和匿名性等因素进行筛选。不同的应用场景可能对代理IP有不同的要求,例如,有些场景需要高匿名的代理,而有些场景则需要特定国家或地区的代理。
维护代理IP需要定期验证和更新。代理IP可能会因为各种原因失效,因此,我们需要定期运行验证程序,及时识别和替换失效的代理IP。此外,我们还可以建立一个代理池,动态管理代理IP的使用。
class ProxyManager:
def __init__(self, proxies):
self.proxies = proxies
self.valid_proxies = []
def validate_proxies(self):
self.valid_proxies = batch_check_proxies(self.proxies)
def get_valid_proxies(self):
return self.valid_proxies
def refresh_proxies(self, new_proxies):
self.proxies = new_proxies
self.validate_proxies()
Example usage
proxies = [
'http://123.123.123.123:8080',
'http://111.111.111.111:8080',
'http://222.222.222.222:8080'
]
manager = ProxyManager(proxies)
manager.validate_proxies()
print("Valid proxies:", manager.get_valid_proxies())
七、代理IP验证的注意事项
在实际应用中,验证代理IP时还需要注意一些细节问题。例如,不同的网站对代理IP的支持程度不同,因此在选择验证目标网站时需要谨慎。此外,一些网站可能会对频繁的请求进行限制,因此在批量验证代理IP时需要控制请求频率。
为了提高验证的成功率,我们可以在代码中加入重试机制。在请求失败时,可以尝试重新发送请求,尤其是在网络波动或短暂的连接中断时。此外,为了减少对目标网站的影响,可以在请求之间加入适当的延迟。
def check_proxy_with_retry(proxy, max_retries=3):
retries = 0
while retries < max_retries:
try:
response = requests.get('http://httpbin.org/ip', proxies={'http': proxy, 'https': proxy}, timeout=5)
if response.status_code == 200:
print(f"Proxy {proxy} is valid.")
return True
except requests.exceptions.RequestException:
retries += 1
print(f"Retrying proxy {proxy} ({retries}/{max_retries})")
print(f"Proxy {proxy} failed after {max_retries} retries.")
return False
Example usage
check_proxy_with_retry('http://123.123.123.123:8080')
通过遵循以上步骤和注意事项,我们可以在Python中有效地验证代理IP,并选择合适的代理IP进行使用。无论是单个代理IP的验证,还是批量验证和维护代理IP池,这些方法都能帮助我们提高代理IP的使用效率和效果。
相关问答FAQs:
如何检测代理IP是否有效?
要检测代理IP是否有效,可以使用Python编写一个简单的脚本,通过发送HTTP请求来验证代理的可用性。可以尝试访问一个公共的API(如httpbin.org/ip),如果能正常返回IP地址,则说明代理有效。此外,可以设置超时时间,避免长时间等待无效代理的响应。
使用Python库验证代理IP有哪些推荐?
在Python中,有几个库可以帮助验证代理IP,包括requests
、httpx
和PySocks
。requests
库是最常用的,通过设置代理参数进行请求;httpx
支持异步请求,适合高并发场景;而PySocks
可以处理SOCKS代理,适用范围更广。选择合适的库可以提高验证效率。
如果代理IP验证失败,应该采取什么措施?
当代理IP验证失败时,可以考虑更换代理源,尝试使用其他的代理IP。若使用的是公共代理,可以搜索更新的代理列表;若为私有代理,联系服务提供商获取新的IP。此外,定期维护和更新代理IP的列表,可以有效提升爬虫的稳定性和数据抓取的成功率。
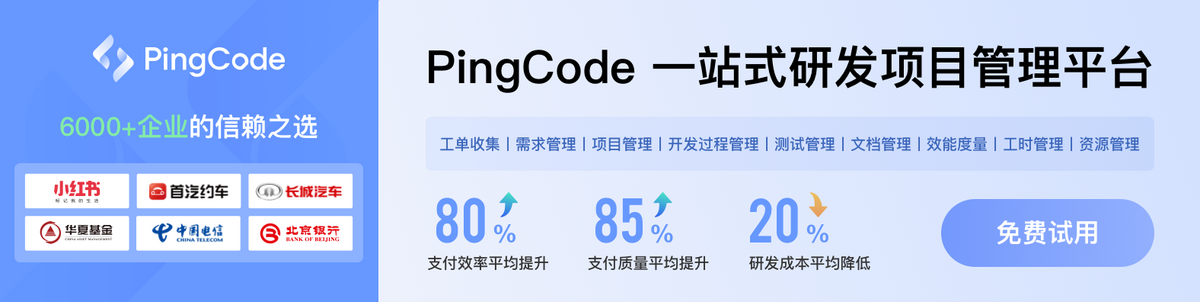