在Python中,变量字符匹配可以通过使用正则表达式、字符串方法(如find、index)和条件判断来实现。具体来说,正则表达式提供了强大的模式匹配能力,适用于复杂的字符匹配需求;字符串方法如find和index则适用于简单的匹配场景;条件判断则用于基本的相等性检查。下面将详细介绍如何使用这三种方法进行字符匹配。
一、正则表达式匹配
正则表达式(Regular Expression)是一种强大的字符串模式匹配工具。在Python中,我们可以使用re
模块来实现复杂的字符匹配。
1、基本使用
正则表达式通过匹配模式定义字符的规则。可以使用re.match()
、re.search()
和re.findall()
等方法进行匹配。
import re
pattern = r"hello"
text = "hello world"
使用 match 从字符串的开头开始匹配
match = re.match(pattern, text)
if match:
print("Match found:", match.group())
else:
print("No match found")
2、搜索和提取
如果需要在字符串的任意位置搜索,可以使用re.search()
:
search = re.search(pattern, text)
if search:
print("Search found:", search.group())
要提取所有匹配的字符串,可以使用re.findall()
:
all_matches = re.findall(pattern, text)
print("All matches found:", all_matches)
二、字符串方法匹配
Python提供了一些内置的字符串方法来进行简单的字符匹配,这些方法操作简单且直观。
1、使用 find 和 index
find()
和index()
方法用于查找子字符串在主字符串中的位置。不同之处在于,find()
在找不到子字符串时返回-1,而index()
则抛出异常。
text = "hello world"
使用 find
pos = text.find("world")
if pos != -1:
print("Found at position:", pos)
else:
print("Not found")
使用 index
try:
pos = text.index("world")
print("Found at position:", pos)
except ValueError:
print("Not found")
2、startswith 和 endswith
startswith()
和endswith()
方法用于检查字符串是否以特定的子字符串开始或结束。
# 检查是否以 hello 开头
if text.startswith("hello"):
print("Starts with 'hello'")
检查是否以 world 结束
if text.endswith("world"):
print("Ends with 'world'")
三、条件判断匹配
对于简单的字符匹配,可以使用条件判断来实现。
1、相等性检查
直接使用==
运算符来比较两个字符串是否相等。
text = "hello world"
if text == "hello world":
print("Exact match found")
2、包含检查
使用in
运算符来检查一个字符串是否包含在另一个字符串中。
if "world" in text:
print("'world' is in text")
四、正则表达式高级用法
1、分组和捕获
正则表达式支持分组和捕获,这对提取子字符串非常有用。
pattern = r"(hello) (world)"
match = re.match(pattern, text)
if match:
print("Groups:", match.groups())
2、替换
使用re.sub()
方法可以替换匹配的字符串。
new_text = re.sub(r"world", "Python", text)
print("Replaced text:", new_text)
五、注意事项和优化
1、性能考虑
正则表达式虽然强大,但在处理大数据时可能会影响性能。应尽量使用简单的字符串方法来处理简单的匹配任务。
2、错误处理
使用字符串方法时,应注意处理可能的异常,例如index()
方法可能抛出的ValueError
。
3、模式设计
设计正则表达式模式时,应尽量避免使用过于复杂或不必要的匹配规则,以提高可读性和性能。
六、实践应用
1、日志分析
在日志分析中,可以使用正则表达式匹配特定的日志格式,提取关键信息。
log_entry = "2023-10-21 10:00:00 ERROR User not found"
pattern = r"(\d{4}-\d{2}-\d{2}) (\d{2}:\d{2}:\d{2}) (\w+) (.+)"
match = re.match(pattern, log_entry)
if match:
print("Date:", match.group(1))
print("Time:", match.group(2))
print("Level:", match.group(3))
print("Message:", match.group(4))
2、数据清洗
在数据清洗过程中,可以使用正则表达式去除无用的字符或格式化数据。
raw_data = "Name: John Doe, Age: 30, Email: john.doe@example.com"
clean_data = re.sub(r"[^a-zA-Z0-9@. ]", "", raw_data)
print("Clean data:", clean_data)
通过以上介绍,可以看到Python提供了多种字符匹配的方法,适用于不同复杂程度的需求。根据具体的应用场景选择合适的方法,可以有效提高开发效率和代码的可读性。
相关问答FAQs:
如何在Python中使用正则表达式进行字符串匹配?
在Python中,可以使用re
模块来执行正则表达式匹配。通过导入re
模块,您可以使用re.match()
, re.search()
和re.findall()
等函数进行复杂的字符串匹配。这些函数允许您根据特定模式搜索字符串,识别子字符串,甚至进行替换操作。例如,使用re.search(r'\d+', string)
可以找到字符串中的所有数字。
Python中有没有内置函数可以进行简单的字符串匹配?
是的,Python提供了一些内置方法用于简单的字符串匹配。常用的方法包括in
关键字、str.startswith()
和str.endswith()
。例如,if "hello" in my_string:
可以用来检查字符串my_string
中是否包含“hello”。这些方法简单易用,适合不需要复杂模式匹配的场景。
在Python中如何处理大小写不敏感的字符串匹配?
处理大小写不敏感的字符串匹配可以通过将字符串转换为统一的大小写来实现。可以使用str.lower()
或str.upper()
方法将字符串转换为小写或大写。例如,if my_string.lower() == "hello":
可以确保在比较时不受大小写影响。此外,在使用正则表达式时,可以在模式字符串中添加re.IGNORECASE
标志,以实现大小写不敏感匹配。
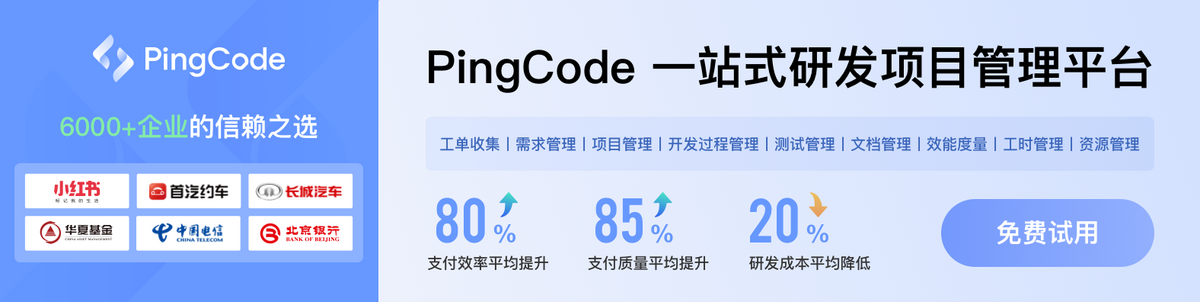