Python中使用HTTPS请求的方法有多种,常见的包括:使用requests
库、使用http.client
模块、使用urllib
模块。推荐使用requests
库,因为它简单易用、功能强大。下面将详细介绍如何使用requests
库来执行HTTPS请求。
requests
库是一个非常强大的HTTP库,可以轻松地处理GET、POST等HTTP请求,并自动处理复杂的HTTP任务,如重定向和会话管理。要使用requests
库,首先需要安装它,可以通过运行pip install requests
命令来安装。
一、安装与导入
在使用requests
库之前,首先需要确保该库已安装。可以通过以下命令进行安装:
pip install requests
安装完成后,在Python脚本中导入该库:
import requests
二、执行GET请求
GET请求用于从服务器请求数据。以下是使用requests
库进行GET请求的基本示例:
response = requests.get('https://api.example.com/data')
print(response.status_code)
print(response.text)
在这个例子中,requests.get()
函数用于向指定的URL发送GET请求。返回的response
对象包含服务器的响应信息,如状态码和响应内容。
1.1、处理响应
在发送请求后,可以通过response
对象获取响应状态码、响应头和响应内容:
print(response.status_code) # 获取响应状态码
print(response.headers) # 获取响应头
print(response.text) # 获取响应内容
1.2、处理JSON响应
如果服务器返回JSON格式的数据,可以使用response.json()
方法将其解析为Python字典:
json_data = response.json()
print(json_data)
三、执行POST请求
POST请求用于向服务器发送数据。以下是使用requests
库进行POST请求的基本示例:
data = {'key1': 'value1', 'key2': 'value2'}
response = requests.post('https://api.example.com/submit', data=data)
print(response.status_code)
print(response.text)
在这个例子中,requests.post()
函数用于向指定的URL发送POST请求,并将数据作为表单格式发送。
2.1、发送JSON数据
如果需要发送JSON格式的数据,可以使用json
参数:
import json
data = {'key1': 'value1', 'key2': 'value2'}
response = requests.post('https://api.example.com/submit', json=data)
print(response.status_code)
print(response.text)
四、添加请求头
有时需要在请求中添加自定义的头信息,可以通过headers
参数实现:
headers = {'Authorization': 'Bearer your_token'}
response = requests.get('https://api.example.com/protected', headers=headers)
print(response.status_code)
print(response.text)
五、使用会话对象
requests
库提供了会话对象Session
,用于跨请求保持某些参数,比如cookie:
session = requests.Session()
session.headers.update({'Authorization': 'Bearer your_token'})
response = session.get('https://api.example.com/protected')
print(response.status_code)
response = session.post('https://api.example.com/submit', json={'key': 'value'})
print(response.status_code)
会话对象允许你在多个请求之间保持一些状态信息,比如cookie和头信息,从而减少重复代码。
六、处理SSL证书
requests
库默认会验证SSL证书。如果你需要忽略SSL验证,可以使用verify=False
参数(不推荐在生产环境中使用):
response = requests.get('https://self-signed.badssl.com/', verify=False)
print(response.status_code)
七、错误处理
在使用requests
库时,可能会遇到网络错误、超时等问题。可以通过捕获异常进行处理:
try:
response = requests.get('https://api.example.com/data', timeout=5)
response.raise_for_status() # 如果响应状态码不是200,抛出异常
except requests.exceptions.HTTPError as http_err:
print(f'HTTP error occurred: {http_err}')
except requests.exceptions.ConnectionError as conn_err:
print(f'Connection error occurred: {conn_err}')
except requests.exceptions.Timeout as timeout_err:
print(f'Timeout error occurred: {timeout_err}')
except requests.exceptions.RequestException as req_err:
print(f'Request error occurred: {req_err}')
通过这种方式,可以更好地控制和处理HTTP请求过程中可能出现的各种异常。
八、总结
使用requests
库进行HTTPS请求是Python中一种简单而强大的方法。通过GET和POST请求,可以轻松地从服务器获取数据或发送数据。使用会话对象可以在多个请求之间保持状态信息,而通过自定义请求头和处理SSL证书,可以满足更复杂的需求。同时,通过异常处理,能够更好地应对网络错误和超时问题。总之,requests
库提供了丰富的功能,能够满足大多数HTTP请求的需求。
相关问答FAQs:
如何在Python中发送HTTPS请求?
在Python中,可以使用requests
库轻松发送HTTPS请求。首先,需要确保安装了requests
库,可以通过命令pip install requests
进行安装。安装完成后,可以使用如下代码发送GET请求:
import requests
response = requests.get('https://example.com')
print(response.text)
对于POST请求,可以使用类似的方式:
response = requests.post('https://example.com', data={'key': 'value'})
print(response.text)
如何处理HTTPS请求中的SSL证书验证?
在发送HTTPS请求时,requests
库会默认验证SSL证书以确保安全性。如果需要禁用SSL验证,可以在请求中设置verify=False
,但这不推荐在生产环境中使用,因为可能会导致安全风险:
response = requests.get('https://example.com', verify=False)
建议在开发过程中只在信任的环境中使用此选项。
如何在Python中处理HTTPS请求的异常情况?
使用requests
库时,可以通过try-except
语句来捕获各种异常,包括连接错误、超时等。这有助于提高代码的健壮性:
try:
response = requests.get('https://example.com')
response.raise_for_status() # 如果响应状态码不是200,会引发HTTPError
except requests.exceptions.HTTPError as errh:
print(f"HTTP错误: {errh}")
except requests.exceptions.ConnectionError as errc:
print(f"连接错误: {errc}")
except requests.exceptions.Timeout as errt:
print(f"请求超时: {errt}")
except requests.exceptions.RequestException as err:
print(f"其他错误: {err}")
通过这种方式,可以有效应对各种网络请求中的问题。
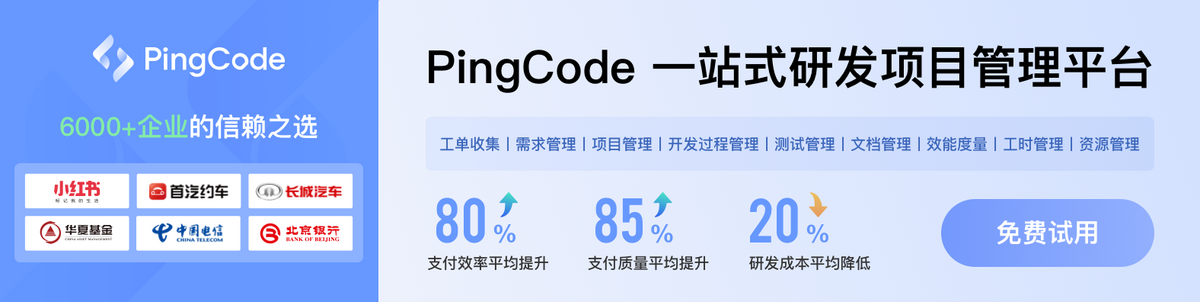