用Python实现猜拳可以通过使用随机数生成计算机的选择、获取用户的输入、比较两者的选择来决定胜负、并循环运行游戏以便多次玩。在这篇文章中,我们将详细介绍如何用Python编写一个简单的猜拳游戏,并逐步深入到高级主题,如添加计分系统、使用函数和类来组织代码等。
一、基础实现
在实现一个猜拳游戏时,我们首先需要了解游戏的基本规则:玩家和计算机各自选择“石头”、“剪刀”或“布”中的一个,然后比较两者的选择来决定胜负。游戏的规则是:石头胜剪刀、剪刀胜布、布胜石头。
- 导入必要的模块
首先,我们需要导入Python的random
模块,它允许我们生成随机数,这对于计算机的选择非常重要。
import random
- 定义主要变量
我们需要定义一个列表,包含游戏的三个选择:石头、剪刀、布。这将帮助我们在生成随机选择和比较结果时使用。
choices = ["石头", "剪刀", "布"]
- 获取用户输入
接下来,我们需要获取用户的选择。在Python中,我们可以使用input()
函数来实现这一点。
user_choice = input("请输入您的选择(石头/剪刀/布):")
- 生成计算机选择
使用random.choice()
函数从我们的choices
列表中随机选择一个项目,作为计算机的选择。
computer_choice = random.choice(choices)
print(f"计算机选择了:{computer_choice}")
- 比较和决定胜负
我们需要编写逻辑来比较用户和计算机的选择,然后输出结果。
if user_choice == computer_choice:
print("平局!")
elif (user_choice == "石头" and computer_choice == "剪刀") or \
(user_choice == "剪刀" and computer_choice == "布") or \
(user_choice == "布" and computer_choice == "石头"):
print("您赢了!")
else:
print("计算机赢了!")
二、添加游戏循环
为使游戏更加有趣,我们可以添加一个循环,让玩家可以多次玩游戏。
- 使用while循环
我们可以使用一个while
循环来实现游戏的重复运行,并提供一个退出选项。
while True:
user_choice = input("请输入您的选择(石头/剪刀/布),或输入'退出'结束游戏:")
if user_choice == '退出':
break
if user_choice not in choices:
print("无效选择,请重新输入。")
continue
computer_choice = random.choice(choices)
print(f"计算机选择了:{computer_choice}")
if user_choice == computer_choice:
print("平局!")
elif (user_choice == "石头" and computer_choice == "剪刀") or \
(user_choice == "剪刀" and computer_choice == "布") or \
(user_choice == "布" and computer_choice == "石头"):
print("您赢了!")
else:
print("计算机赢了!")
三、添加计分系统
为了增加游戏的互动性,我们可以添加一个简单的计分系统,记录玩家和计算机的胜利次数。
- 初始化分数
在游戏开始时,初始化玩家和计算机的分数为0。
user_score = 0
computer_score = 0
- 更新分数
在每轮游戏结束后,根据胜负更新分数,并显示当前比分。
if user_choice == computer_choice:
print("平局!")
elif (user_choice == "石头" and computer_choice == "剪刀") or \
(user_choice == "剪刀" and computer_choice == "布") or \
(user_choice == "布" and computer_choice == "石头"):
print("您赢了!")
user_score += 1
else:
print("计算机赢了!")
computer_score += 1
print(f"当前比分 - 您: {user_score}, 计算机: {computer_score}")
四、代码优化和函数化
为了提高代码的可读性和可维护性,我们可以将游戏的各个部分封装到函数中。
- 定义函数
我们可以为用户输入、生成计算机选择和决定胜负等功能定义单独的函数。
def get_user_choice():
return input("请输入您的选择(石头/剪刀/布),或输入'退出'结束游戏:")
def get_computer_choice():
return random.choice(choices)
def determine_winner(user_choice, computer_choice):
if user_choice == computer_choice:
return "平局"
elif (user_choice == "石头" and computer_choice == "剪刀") or \
(user_choice == "剪刀" and computer_choice == "布") or \
(user_choice == "布" and computer_choice == "石头"):
return "用户"
else:
return "计算机"
- 重构主逻辑
使用定义的函数来简化主程序逻辑。
while True:
user_choice = get_user_choice()
if user_choice == '退出':
break
if user_choice not in choices:
print("无效选择,请重新输入。")
continue
computer_choice = get_computer_choice()
print(f"计算机选择了:{computer_choice}")
winner = determine_winner(user_choice, computer_choice)
if winner == "平局":
print("平局!")
elif winner == "用户":
print("您赢了!")
user_score += 1
else:
print("计算机赢了!")
computer_score += 1
print(f"当前比分 - 您: {user_score}, 计算机: {computer_score}")
五、使用类封装游戏逻辑
为了更好地组织代码,我们可以使用类来封装游戏的逻辑和状态。
- 定义游戏类
将所有功能整合到一个类中,以便更好地管理状态和方法。
class RockPaperScissorsGame:
def __init__(self):
self.choices = ["石头", "剪刀", "布"]
self.user_score = 0
self.computer_score = 0
def get_user_choice(self):
return input("请输入您的选择(石头/剪刀/布),或输入'退出'结束游戏:")
def get_computer_choice(self):
return random.choice(self.choices)
def determine_winner(self, user_choice, computer_choice):
if user_choice == computer_choice:
return "平局"
elif (user_choice == "石头" and computer_choice == "剪刀") or \
(user_choice == "剪刀" and computer_choice == "布") or \
(user_choice == "布" and computer_choice == "石头"):
return "用户"
else:
return "计算机"
def play(self):
while True:
user_choice = self.get_user_choice()
if user_choice == '退出':
break
if user_choice not in self.choices:
print("无效选择,请重新输入。")
continue
computer_choice = self.get_computer_choice()
print(f"计算机选择了:{computer_choice}")
winner = self.determine_winner(user_choice, computer_choice)
if winner == "平局":
print("平局!")
elif winner == "用户":
print("您赢了!")
self.user_score += 1
else:
print("计算机赢了!")
self.computer_score += 1
print(f"当前比分 - 您: {self.user_score}, 计算机: {self.computer_score}")
- 运行游戏
创建游戏类的实例并调用play
方法来开始游戏。
game = RockPaperScissorsGame()
game.play()
通过这些步骤,我们就可以用Python实现一个完整的、可循环的猜拳游戏,并且通过使用类和函数来提高代码的组织性和可维护性。这种设计不仅可以帮助理解编程的基本概念,还可以为日后实现更复杂的游戏打下良好的基础。
相关问答FAQs:
如何用Python编写一个简单的猜拳游戏?
要创建一个猜拳游戏,您可以使用Python的基本控制结构和随机模块。首先,导入随机模块以生成电脑的选择。接着,使用输入函数获取用户的选择,并通过条件语句来判断胜负。以下是一个简单的实现示例:
import random
choices = ["石头", "剪刀", "布"]
user_choice = input("请输入你的选择(石头、剪刀、布):")
computer_choice = random.choice(choices)
print(f"电脑选择了:{computer_choice}")
if user_choice == computer_choice:
print("平局!")
elif (user_choice == "石头" and computer_choice == "剪刀") or \
(user_choice == "剪刀" and computer_choice == "布") or \
(user_choice == "布" and computer_choice == "石头"):
print("你赢了!")
else:
print("你输了!")
如何在猜拳游戏中增加多个回合?
可以通过循环结构来实现多个回合的猜拳游戏。在每个回合结束后,可以询问用户是否继续游戏。如果用户选择继续,则重新开始游戏,否则结束程序。以下是如何实现的示例:
while True:
user_choice = input("请输入你的选择(石头、剪刀、布):")
computer_choice = random.choice(choices)
# 判断胜负的代码...
again = input("想再玩一次吗?(是/否)")
if again.lower() != "是":
break
如何处理用户输入的错误?
在猜拳游戏中,用户可能会输入无效的选项。为了提高用户体验,可以在程序中添加输入验证功能,确保用户输入的内容是有效的。可以使用循环来不断提示用户,直到他们输入有效的选项为止。以下是一个示例:
while True:
user_choice = input("请输入你的选择(石头、剪刀、布):")
if user_choice not in choices:
print("无效选择,请重新输入。")
else:
break
通过以上方法,您可以创建一个交互性强且用户友好的猜拳游戏。
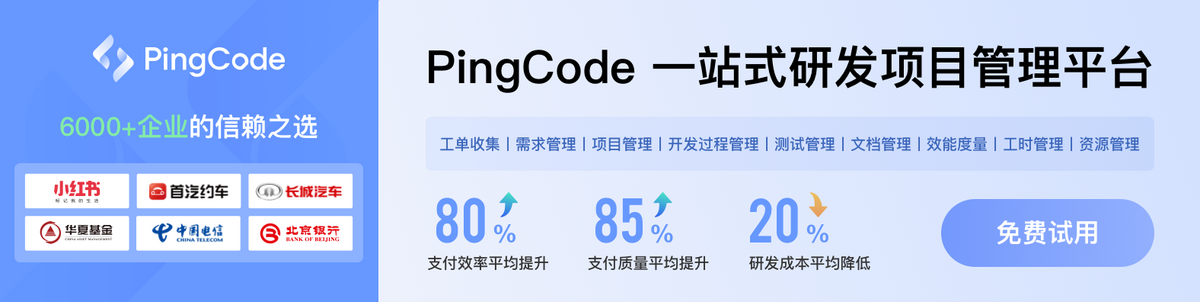