在Python中,输入和打印操作可以通过内置函数input()和print()实现。这两个函数分别用于从用户获取输入、将结果输出到控制台。首先,使用input()函数可以提示用户输入数据,然后将输入的数据存储在一个变量中;接下来,使用print()函数可以将变量值或者其他信息输出到屏幕上。为了更好地理解这两个函数的使用,以下将详细介绍它们的用法、参数以及一些常见的应用场景。
一、INPUT()函数的使用
input()函数是Python中用于接收用户输入的标准函数。调用这个函数时,程序会暂停运行,等待用户输入数据,并在用户按下回车键后继续执行。
- 基本用法
input()函数可以接受一个字符串参数,该参数用于在用户输入前显示提示信息。例如:
name = input("请输入您的姓名:")
在上面的代码中,程序会显示提示信息“请输入您的姓名:”,用户输入的信息将被存储在变量name中。
- 数据类型转换
需要注意的是,input()函数接收到的输入数据默认是字符串类型。如果需要对输入的数据进行数学运算或其他类型操作,需要将其转换为相应的数据类型。例如:
age = int(input("请输入您的年龄:"))
在这里,我们使用int()函数将输入的数据转换为整数类型。
- 处理异常输入
在实际应用中,用户可能会输入不符合预期的数据类型,例如在需要整数输入时输入了字母。为了避免程序崩溃,可以使用异常处理来捕获错误。例如:
try:
age = int(input("请输入您的年龄:"))
except ValueError:
print("输入的不是有效的数字。")
二、PRINT()函数的使用
print()函数用于将信息输出到控制台,是Python中最常用的输出函数之一。
- 基本用法
print()函数可以接受多个参数,并将它们按顺序输出到控制台。每个参数之间可以使用逗号分隔。例如:
print("姓名:", name, "年龄:", age)
- 格式化输出
为了更好地控制输出格式,可以使用格式化字符串。Python提供了多种字符串格式化方式,包括%格式化、str.format()方法和f-string。以下是f-string格式化的例子:
print(f"姓名:{name},年龄:{age}")
- 自定义分隔符和结束符
print()函数默认使用空格作为多个参数之间的分隔符,并在输出后自动换行。可以通过参数sep和end来自定义分隔符和结束符。例如:
print("姓名", name, sep=":", end="。")
三、INPUT()和PRINT()的综合应用
在实际开发中,input()和print()通常结合使用,以实现用户交互功能。以下是一个简单的例子,演示如何使用这两个函数实现一个简易的用户信息收集程序:
def collect_user_info():
name = input("请输入您的姓名:")
age = input("请输入您的年龄:")
city = input("请输入您所在的城市:")
print("\n用户信息如下:")
print(f"姓名:{name}")
print(f"年龄:{age}")
print(f"城市:{city}")
collect_user_info()
四、ERROR HANDLING IN INPUT AND PRINT
- Handling Input Errors
When using input(), there's always a risk of receiving unexpected data types. For instance, when expecting an integer, a user might input a string that cannot be converted. In such cases, implementing a loop with error handling ensures the program can handle erroneous inputs gracefully:
while True:
try:
age = int(input("请输入您的年龄:"))
break
except ValueError:
print("输入的不是有效的数字,请重新输入。")
This loop will continually prompt the user until a valid integer is entered.
- Handling Print Errors
Though rare, print errors can occur, especially if the output device (like a console or a file) is not writable. It's crucial to ensure that the environment where the print function is used is stable and supports writing operations.
五、ADVANCED USAGE SCENARIOS
- Reading Multiple Inputs
Sometimes, you might need to read multiple values in a single line. You can achieve this by using the split() method:
data = input("请输入姓名和年龄,用空格分隔:").split()
name, age = data[0], int(data[1])
This method is efficient when dealing with structured input data.
- Print to a File
In addition to printing to the console, Python's print() function can also be directed to output to a file:
with open("output.txt", "w") as file:
print("Hello, World!", file=file)
This is useful for logging or storing output data for later analysis.
六、BEST PRACTICES
- Consistent User Prompts
Ensure that prompts given to the user are clear and consistent. This helps in reducing input errors and improves the user experience.
- Input Validation
Always validate user inputs to ensure they meet the expected format and type. This prevents unexpected behavior and potential crashes.
- Efficient Output Formatting
Choose the right formatting method based on the complexity and requirement of the output. For most modern applications, f-strings offer a concise and readable way to format strings.
七、CONCLUSION
Understanding how to effectively use input() and print() is fundamental for any Python programmer. They form the basis of user interaction in many command-line applications. By mastering these functions and employing best practices, developers can create robust and user-friendly applications.
相关问答FAQs:
如何在Python中接受用户输入并将其打印出来?
在Python中,可以使用内置的input()
函数来接受用户的输入。这个函数会暂停程序的执行,等待用户输入数据。输入完成后,按下回车键,程序继续执行。可以将输入的数据存储在一个变量中,然后使用print()
函数将其输出。例如:
user_input = input("请输入一些内容: ")
print("你输入的是:", user_input)
Python是否支持输入多行文本并打印出来?
是的,Python支持输入多行文本。可以使用input()
函数多次调用,直到用户输入一个特定的结束符(如“结束”或“exit”)来终止输入。下面是一个示例:
lines = []
while True:
line = input("输入文本(输入'结束'以结束): ")
if line == "结束":
break
lines.append(line)
print("你输入的内容是:")
for line in lines:
print(line)
如何格式化用户输入的内容再打印?
在Python中,可以使用f-string(格式化字符串)或str.format()
方法来格式化用户输入的内容。这样可以使输出更具可读性和美观。例如:
name = input("请输入你的名字: ")
age = input("请输入你的年龄: ")
print(f"{name},你已经{age}岁了!")
或者使用str.format()
方法:
print("{},你已经{}岁了!".format(name, age))
这种方式可以让打印出来的信息更加生动和个性化。
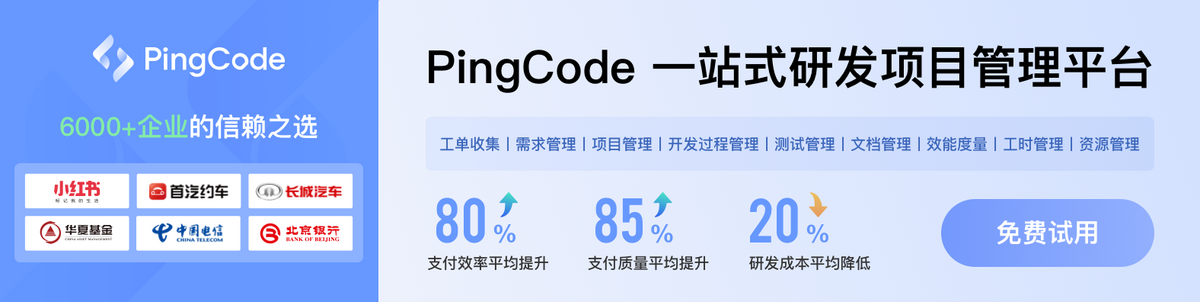