在Python程序中增加字体的方法包括:使用字体库、修改字体属性、加载自定义字体。其中,使用字体库如matplotlib
或pygame
来设置字体是最常见的方法。以下将详细介绍如何在Python中通过不同的途径增加字体。
一、使用FONTS库
- Matplotlib字体设置
matplotlib
是Python中一个强大的绘图库,它不仅可以绘制图形,还可以设置字体。要增加和设置字体,首先需要确保已安装matplotlib
库,然后可以通过rcParams
进行字体设置。
import matplotlib.pyplot as plt
设置全局字体
plt.rcParams['font.family'] = 'serif'
plt.rcParams['font.serif'] = ['Times New Roman']
plt.title('Example Title')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.show()
在上面的代码中,我们使用rcParams
来设置全局字体为Times New Roman
。rcParams
是一个字典结构,允许我们调整matplotlib
的全局设置。
- Pygame字体设置
pygame
是一个用于开发游戏的库,它提供了丰富的多媒体功能,包括字体设置。要在pygame
中增加字体,可以使用pygame.font
模块。
import pygame
初始化pygame
pygame.init()
设置字体
font = pygame.font.SysFont('Arial', 30)
创建窗口
screen = pygame.display.set_mode((800, 600))
渲染字体
text_surface = font.render('Hello, World!', True, (255, 255, 255))
screen.blit(text_surface, (100, 100))
pygame.display.flip()
等待退出
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
pygame.quit()
在pygame
中,通过pygame.font.SysFont
可以加载系统中的字体,并使用render
方法将文本渲染到屏幕上。
二、加载自定义字体
- 使用Matplotlib加载自定义字体
matplotlib
支持加载自定义字体文件(如.ttf
格式)。可以通过FontProperties
类来实现。
from matplotlib.font_manager import FontProperties
import matplotlib.pyplot as plt
加载自定义字体
font_path = '/path/to/font.ttf'
custom_font = FontProperties(fname=font_path)
plt.title('Example Title', fontproperties=custom_font)
plt.xlabel('X-axis', fontproperties=custom_font)
plt.ylabel('Y-axis', fontproperties=custom_font)
plt.show()
通过FontProperties
类的fname
参数,我们可以指定字体文件的路径,从而使用自定义字体。
- 使用Pygame加载自定义字体
在pygame
中,同样可以加载自定义字体文件。
import pygame
初始化pygame
pygame.init()
加载自定义字体
font_path = '/path/to/font.ttf'
font = pygame.font.Font(font_path, 30)
创建窗口
screen = pygame.display.set_mode((800, 600))
渲染字体
text_surface = font.render('Hello, World!', True, (255, 255, 255))
screen.blit(text_surface, (100, 100))
pygame.display.flip()
等待退出
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
pygame.quit()
通过pygame.font.Font
类,我们可以加载任意路径下的字体文件。
三、修改字体属性
- 在Matplotlib中修改字体属性
在matplotlib
中,我们可以通过改变rcParams
字典中的键值对来修改字体的各项属性,包括字体大小、颜色等。
import matplotlib.pyplot as plt
plt.rcParams['font.size'] = 14
plt.rcParams['axes.titlesize'] = 18
plt.rcParams['axes.labelsize'] = 16
plt.title('Example Title')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.show()
以上代码示例展示了如何修改字体大小,通过调整rcParams
中的font.size
、axes.titlesize
等键值对来实现。
- 在Pygame中修改字体属性
在pygame
中,通过创建font
对象时传递不同的参数,可以轻松调整字体大小和样式。
import pygame
初始化pygame
pygame.init()
设置字体大小
font_size = 40
font = pygame.font.SysFont('Arial', font_size)
创建窗口
screen = pygame.display.set_mode((800, 600))
渲染字体
text_surface = font.render('Hello, World!', True, (255, 255, 255))
screen.blit(text_surface, (100, 100))
pygame.display.flip()
等待退出
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
pygame.quit()
通过调整pygame.font.SysFont
或pygame.font.Font
的第二个参数,可以改变字体的大小。
四、其他Python库中的字体设置
- Tkinter字体设置
Tkinter
是Python的标准GUI库,它也提供了字体设置功能。使用tkinter.font
模块可以设置字体。
import tkinter as tk
from tkinter import font
root = tk.Tk()
设置字体
my_font = font.Font(family='Helvetica', size=12, weight='bold')
label = tk.Label(root, text='Hello, World!', font=my_font)
label.pack()
root.mainloop()
在Tkinter
中,通过font.Font
类可以指定字体的家族、大小和样式。
- PIL(Pillow)字体设置
PIL
(即Pillow
)是Python的一种图像处理库,它也提供了字体设置功能,主要用于在图像上绘制文本。
from PIL import Image, ImageDraw, ImageFont
创建空白图像
image = Image.new('RGB', (800, 600), (255, 255, 255))
加载字体
font_path = '/path/to/font.ttf'
font = ImageFont.truetype(font_path, 40)
在图像上绘制文本
draw = ImageDraw.Draw(image)
draw.text((100, 100), 'Hello, World!', font=font, fill=(0, 0, 0))
显示图像
image.show()
通过ImageFont.truetype
方法,我们可以加载TTF字体文件,然后在图像上绘制文本。
五、总结
在Python程序中增加和设置字体有多种方法,选择合适的工具和库取决于具体的应用场景。无论是用于数据可视化的matplotlib
,还是用于游戏开发的pygame
,亦或是用于GUI的Tkinter
,都提供了丰富的字体设置功能。通过熟练掌握这些库的字体设置方法,我们可以在Python程序中实现更丰富的文本呈现效果。
相关问答FAQs:
如何在Python程序中设置字体大小?
在Python中,可以使用多个库来设置字体大小,例如Tkinter、Pygame或Matplotlib。以Tkinter为例,可以在创建标签或文本框时,通过font
参数来指定字体大小。示例代码如下:
import tkinter as tk
from tkinter import font
root = tk.Tk()
my_font = font.Font(size=20) # 设置字体大小为20
label = tk.Label(root, text="Hello, World!", font=my_font)
label.pack()
root.mainloop()
使用Matplotlib绘图时,如何修改字体大小?
在Matplotlib中,可以通过fontsize
参数来设置字体大小。在绘制图表时,可以针对标题、坐标轴标签和图例单独设置字体大小。例如:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3], [4, 5, 6])
plt.title("Sample Title", fontsize=18) # 设置标题字体大小
plt.xlabel("X-axis", fontsize=14) # 设置X轴标签字体大小
plt.ylabel("Y-axis", fontsize=14) # 设置Y轴标签字体大小
plt.show()
在Pygame中如何改变字体大小?
在Pygame中,使用pygame.font.Font
来创建字体对象,并通过size
参数指定字体大小。以下是一个示例:
import pygame
pygame.init()
screen = pygame.display.set_mode((400, 300))
font = pygame.font.Font(None, 36) # 设置字体大小为36
text = font.render("Hello, Pygame!", True, (255, 255, 255))
screen.blit(text, (50, 50))
pygame.display.flip()
# 事件循环
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
pygame.quit()
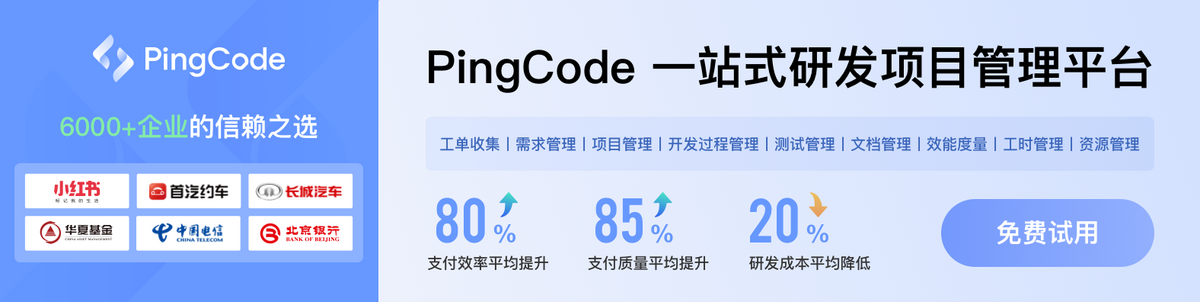