搭建用Python写接口的后台可以通过以下几种步骤来实现:选择合适的框架、定义路由和视图、连接数据库、实现中间件、部署与监控。下面将详细介绍如何通过这些步骤来搭建一个用Python写接口的后台系统。
一、选择合适的框架
选择一个合适的框架是搭建后台系统的第一步。Python有许多流行的框架可以用于开发API接口,其中最常用的包括Flask、Django和FastAPI。不同的框架有不同的特点和优势,选择时需要根据项目需求来决定。
-
Flask
Flask是一个轻量级的Web框架,适合小型项目和快速原型开发。它的设计理念是简单而灵活,开发者可以选择自己需要的组件来搭建系统。
-
Django
Django是一个功能强大的Web框架,适合大型项目和复杂的应用开发。它提供了丰富的内置功能,如ORM、用户认证、管理后台等,可以大大提高开发效率。
-
FastAPI
FastAPI是一个现代、快速(高性能)的Web框架,适合需要高性能和高并发的项目。它基于标准的Python类型提示,能够自动生成文档,并且支持异步编程。
二、定义路由和视图
在选择好框架后,下一步是定义路由和视图。路由是指URL与视图函数之间的映射关系,而视图函数则是处理请求并返回响应的函数。
- Flask示例
from flask import Flask, jsonify, request
app = Flask(__name__)
@app.route('/api/v1/resource', methods=['GET'])
def get_resource():
data = {"message": "Hello, World!"}
return jsonify(data), 200
@app.route('/api/v1/resource', methods=['POST'])
def create_resource():
request_data = request.get_json()
data = {"message": "Resource created", "data": request_data}
return jsonify(data), 201
if __name__ == '__main__':
app.run(debug=True)
- Django示例
from django.urls import path
from django.http import JsonResponse
from django.views.decorators.csrf import csrf_exempt
def get_resource(request):
data = {"message": "Hello, World!"}
return JsonResponse(data, status=200)
@csrf_exempt
def create_resource(request):
if request.method == 'POST':
request_data = json.loads(request.body)
data = {"message": "Resource created", "data": request_data}
return JsonResponse(data, status=201)
urlpatterns = [
path('api/v1/resource', get_resource),
path('api/v1/resource', create_resource),
]
- FastAPI示例
from fastapi import FastAPI
from pydantic import BaseModel
app = FastAPI()
class Resource(BaseModel):
name: str
value: int
@app.get("/api/v1/resource")
async def get_resource():
return {"message": "Hello, World!"}
@app.post("/api/v1/resource")
async def create_resource(resource: Resource):
return {"message": "Resource created", "data": resource}
三、连接数据库
大多数后台系统都需要与数据库进行交互,以存储和检索数据。Python支持多种数据库,可以选择适合项目的数据库,如SQLite、MySQL、PostgreSQL等。
- 使用SQLAlchemy连接数据库(以Flask为例)
from flask import Flask, jsonify, request
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///example.db'
db = SQLAlchemy(app)
class Resource(db.Model):
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(50))
value = db.Column(db.Integer)
@app.route('/api/v1/resource', methods=['GET'])
def get_resource():
resources = Resource.query.all()
data = [{"id": r.id, "name": r.name, "value": r.value} for r in resources]
return jsonify(data), 200
@app.route('/api/v1/resource', methods=['POST'])
def create_resource():
request_data = request.get_json()
resource = Resource(name=request_data['name'], value=request_data['value'])
db.session.add(resource)
db.session.commit()
return jsonify({"message": "Resource created"}), 201
if __name__ == '__main__':
app.run(debug=True)
四、实现中间件
中间件是在请求和响应处理过程中执行的代码,可以用于日志记录、用户认证、请求验证等功能。
- 在Flask中实现中间件
from flask import Flask, request
app = Flask(__name__)
@app.before_request
def log_request():
print(f"Request: {request.method} {request.url}")
@app.after_request
def log_response(response):
print(f"Response: {response.status}")
return response
if __name__ == '__main__':
app.run(debug=True)
- 在Django中实现中间件
class LogMiddleware:
def __init__(self, get_response):
self.get_response = get_response
def __call__(self, request):
print(f"Request: {request.method} {request.path}")
response = self.get_response(request)
print(f"Response: {response.status_code}")
return response
在settings.py中添加中间件
MIDDLEWARE = [
# 其他中间件
'myapp.middleware.LogMiddleware',
]
五、部署与监控
开发完后台系统后,需要将其部署到生产环境,并进行监控以确保系统的稳定运行。
- 使用Gunicorn部署Flask应用
pip install gunicorn
gunicorn -w 4 -b 0.0.0.0:8000 app:app
- 使用Django自带的管理命令进行部署
python manage.py runserver 0.0.0.0:8000
- 使用Uvicorn部署FastAPI应用
pip install uvicorn
uvicorn app:app --host 0.0.0.0 --port 8000
监控后台系统可以使用工具如Prometheus、Grafana、ELK等,这些工具可以帮助收集和分析系统的日志和指标,从而及时发现和解决问题。
通过以上步骤,可以搭建一个用Python写接口的后台系统。选择合适的框架、定义路由和视图、连接数据库、实现中间件、部署与监控是搭建后台系统的关键步骤。希望这篇文章对你有所帮助。
相关问答FAQs:
如何选择合适的框架来搭建Python接口后台?
在搭建Python接口后台时,选择合适的框架至关重要。常用的框架包括Flask和Django。Flask适合小型项目,灵活性高,易于上手;Django则适合大型项目,提供了更多的功能和内置工具,如ORM和管理后台。选择框架时,需考虑项目规模、团队技术栈以及未来的扩展需求。
搭建Python接口后台需要哪些基础知识?
在搭建Python接口后台之前,掌握一些基础知识是很重要的。熟悉Python语言本身是必需的,同时了解HTTP协议、RESTful API的设计原则,以及如何使用数据库(如MySQL或PostgreSQL)进行数据存储。此外,理解基本的前端技术(如HTML、JavaScript)将有助于与前端开发人员的协作。
如何进行Python接口的测试和调试?
测试和调试是确保Python接口正常运行的重要环节。可以使用Postman等工具进行接口测试,模拟请求并检查响应数据。对于单元测试,Python的unittest和pytest库非常有用,它们可以帮助开发人员自动化测试流程,确保代码在修改后仍能正常工作。此外,使用日志记录工具(如logging模块)可以帮助追踪问题和优化性能。
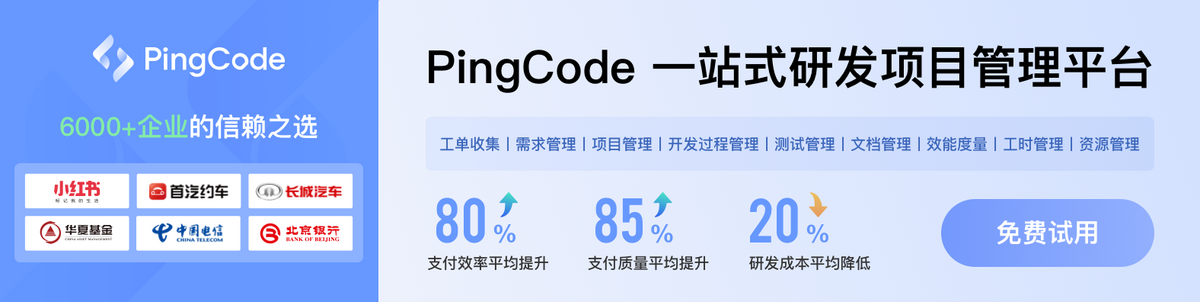