利用Python进行文字匹配的主要方法包括:使用字符串方法、正则表达式、NLTK库。其中,使用正则表达式(Regular Expressions,简称Regex)是最为强大和灵活的一种方法。下面我们详细介绍如何使用正则表达式进行文字匹配。
一、使用字符串方法进行文字匹配
Python内置的字符串方法可以完成一些基本的文字匹配任务。常用的方法包括find()
、index()
、startswith()
和endswith()
。
1.1、find()和index()
find()
方法用于查找子字符串在字符串中的位置,如果找到则返回子字符串的第一个字符的索引,否则返回-1。index()
方法与find()
类似,但如果没有找到子字符串,则会抛出一个异常。
text = "Hello, welcome to the world of Python."
result = text.find("welcome")
print(result) # 输出:7
result = text.find("goodbye")
print(result) # 输出:-1
result = text.index("Python")
print(result) # 输出:26
下面的语句会抛出异常ValueError: substring not found
result = text.index("Java")
1.2、startswith()和endswith()
startswith()
方法用于检查字符串是否以指定的子字符串开头,endswith()
方法用于检查字符串是否以指定的子字符串结尾。
text = "Hello, welcome to the world of Python."
result = text.startswith("Hello")
print(result) # 输出:True
result = text.endswith("Python.")
print(result) # 输出:True
result = text.startswith("world")
print(result) # 输出:False
二、使用正则表达式进行文字匹配
正则表达式是一种用于匹配字符串的强大工具。Python的re
模块提供了对正则表达式的支持。
2.1、基本的正则表达式匹配
使用re.match()
和re.search()
方法进行基本的正则表达式匹配。re.match()
从字符串的开头进行匹配,而re.search()
会搜索整个字符串。
import re
text = "Hello, welcome to the world of Python."
pattern = r"welcome"
result = re.match(pattern, text)
print(result) # 输出:None,因为`welcome`不在开头
result = re.search(pattern, text)
print(result) # 输出:<re.Match object; span=(7, 14), match='welcome'>
2.2、使用正则表达式进行复杂匹配
正则表达式支持多种匹配模式和操作符,可以进行复杂的匹配任务。
import re
text = "The quick brown fox jumps over the lazy dog. 1234567890"
匹配所有单词
pattern = r"\b\w+\b"
matches = re.findall(pattern, text)
print(matches) # 输出:['The', 'quick', 'brown', 'fox', 'jumps', 'over', 'the', 'lazy', 'dog', '1234567890']
匹配所有数字
pattern = r"\d+"
matches = re.findall(pattern, text)
print(matches) # 输出:['1234567890']
匹配单词以o结尾的单词
pattern = r"\b\w+o\b"
matches = re.findall(pattern, text)
print(matches) # 输出:['brown', 'jumps', 'over', 'dog']
三、使用NLTK库进行文字匹配
NLTK(Natural Language Toolkit)是一个用于处理和分析文本数据的Python库。它提供了丰富的工具和数据集,适用于自然语言处理任务。
3.1、分词和词性标注
NLTK库可以方便地进行分词和词性标注。
import nltk
from nltk.tokenize import word_tokenize
from nltk.corpus import stopwords
text = "Hello, welcome to the world of Python."
分词
tokens = word_tokenize(text)
print(tokens) # 输出:['Hello', ',', 'welcome', 'to', 'the', 'world', 'of', 'Python', '.']
词性标注
tagged = nltk.pos_tag(tokens)
print(tagged) # 输出:[('Hello', 'NNP'), (',', ','), ('welcome', 'JJ'), ('to', 'TO'), ('the', 'DT'), ('world', 'NN'), ('of', 'IN'), ('Python', 'NNP'), ('.', '.')]
3.2、去除停用词
NLTK库还提供了常见的停用词表,可以用于去除文本中的停用词。
import nltk
from nltk.corpus import stopwords
text = "This is a sample sentence, showing off the stop words filtration."
分词
tokens = nltk.word_tokenize(text)
去除停用词
filtered_tokens = [word for word in tokens if word.lower() not in stopwords.words('english')]
print(filtered_tokens) # 输出:['This', 'sample', 'sentence', ',', 'showing', 'stop', 'words', 'filtration', '.']
四、综合应用实例
为了更好地理解如何利用Python进行文字匹配,我们可以通过一个综合应用实例来展示。
假设我们有一段文本,包含多个句子,我们需要完成以下任务:
- 找出所有包含特定关键词的句子。
- 统计每个单词出现的次数。
- 去除所有停用词。
- 按照词频排序输出结果。
import re
import nltk
from collections import Counter
from nltk.corpus import stopwords
示例文本
text = """
Python is an amazing programming language.
It is widely used in web development, data science, artificial intelligence, and more.
Python has a simple syntax that is easy to learn.
Many developers love Python for its versatility and ease of use.
"""
1. 找出所有包含特定关键词的句子
keyword = "Python"
sentences = text.split('\n')
keyword_sentences = [sentence for sentence in sentences if re.search(keyword, sentence, re.IGNORECASE)]
print("包含关键词的句子:")
for sentence in keyword_sentences:
print(sentence)
2. 统计每个单词出现的次数
tokens = nltk.word_tokenize(text)
word_counts = Counter(tokens)
print("\n单词出现次数:")
for word, count in word_counts.items():
print(f"{word}: {count}")
3. 去除所有停用词
filtered_tokens = [word for word in tokens if word.lower() not in stopwords.words('english')]
filtered_word_counts = Counter(filtered_tokens)
print("\n去除停用词后的单词出现次数:")
for word, count in filtered_word_counts.items():
print(f"{word}: {count}")
4. 按照词频排序输出结果
sorted_word_counts = sorted(filtered_word_counts.items(), key=lambda x: x[1], reverse=True)
print("\n按照词频排序后的单词出现次数:")
for word, count in sorted_word_counts:
print(f"{word}: {count}")
总结
利用Python进行文字匹配的方法多种多样,从简单的字符串方法到强大的正则表达式,再到专业的自然语言处理库NLTK,各有其适用场景和优势。在实际应用中,可以根据具体需求选择合适的方法,甚至可以结合多种方法来完成更复杂的文本处理任务。通过这些工具和技术,我们可以轻松地进行文字匹配和文本分析,从而更好地理解和利用文本数据。
相关问答FAQs:
利用Python进行文字匹配的基本方法是什么?
Python提供了多种方法来进行文字匹配,包括使用内置字符串方法、正则表达式和第三方库。对于简单的匹配,可以使用字符串的in
关键字或str.find()
方法。如果需要更复杂的模式匹配,Python的re
模块提供了强大的正则表达式支持,可以用于查找、替换和分割字符串。
在Python中如何使用正则表达式进行文字匹配?
使用正则表达式进行文字匹配时,可以导入re
模块并使用如re.match()
, re.search()
, re.findall()
等函数。re.match()
用于从字符串的开始位置匹配模式,re.search()
会扫描整个字符串寻找匹配,而re.findall()
则返回所有匹配的结果列表。正则表达式的语法非常灵活,可以构建复杂的匹配规则,例如使用元字符、字符类和量词等。
如何提高Python文字匹配的效率?
为了提高文字匹配的效率,可以考虑使用编译正则表达式。通过re.compile()
函数将正则表达式编译成一个可重用的模式对象,这样在多次匹配时会显著提高性能。此外,尽量减少不必要的匹配操作,例如在进行大文本处理时可以先使用简单的字符串方法过滤出可能匹配的部分,再应用复杂的正则表达式进行精确匹配。
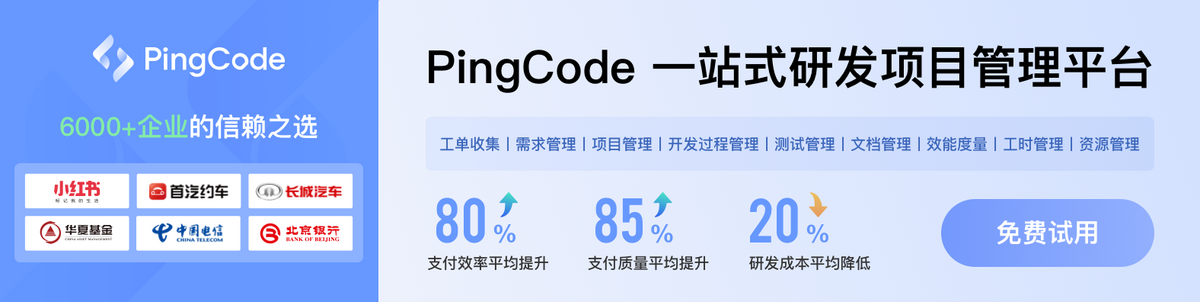